Java Reflection: Exploring Dynamic Class Loading
In the world of Java programming, there’s a powerful technique that allows you to peer into the inner workings of classes and objects, enabling you to manipulate them dynamically at runtime. This technique is known as Java Reflection. But what exactly is reflection, and how does it relate to dynamic class loading? In this blog post, we’ll dive deep into the world of Java Reflection, understand its concepts, and explore how it facilitates dynamic class loading for building flexible and extensible applications.
Table of Contents
1. Understanding Java Reflection
At its core, Java Reflection is a mechanism that enables you to examine or modify the runtime behavior of applications running on the Java Virtual Machine (JVM). It provides the ability to inspect and manipulate classes, methods, fields, constructors, and other structural elements of a Java program during runtime. This introspective capability opens up a plethora of possibilities, from building powerful debugging tools to implementing advanced frameworks.
2. The java.lang.reflect Package
Java Reflection is made possible through the java.lang.reflect package, which contains classes and interfaces that provide the necessary tools for examining and interacting with classes and their members. Some of the key classes and interfaces in this package include:
- Class: Represents a class or interface and provides methods for examining its metadata.
- Field: Represents a field (member variable) within a class.
- Method: Represents a method (member function) within a class.
- Constructor: Represents a constructor for creating instances of a class.
- Modifier: Provides methods to interpret the access modifiers of classes and members.
- Proxy: Facilitates the creation of dynamic proxy instances for interfaces.
3. Dynamic Class Loading
Dynamic class loading is a critical aspect of Java Reflection. It allows you to load and utilize classes during runtime that were not known at compile time. This capability enables you to build more flexible and extensible applications, as you can introduce new functionality without modifying or recompiling the existing codebase.
4. The ClassLoader
In Java, classes are loaded into memory by a class loader. The JVM has three built-in class loaders:
- Bootstrap Class Loader: Responsible for loading core Java classes from the system classpath.
- Extension Class Loader: Loads classes from the extension directories.
- Application Class Loader: Loads classes from the classpath specified while running the application.
You can also create custom class loaders that extend the ClassLoader class, enabling you to control how classes are loaded into memory. This feature is fundamental to achieving dynamic class loading.
5. Using Class.forName()
One common way to dynamically load classes is by using the Class.forName(String className) method. This method takes a fully qualified class name as a parameter and returns a Class object that represents the loaded class. Here’s a simple example:
java try { String className = "com.example.DynamicClass"; Class<?> dynamicClass = Class.forName(className); } catch (ClassNotFoundException e) { e.printStackTrace(); }
In this example, if the class com.example.DynamicClass is not present in the classpath, a ClassNotFoundException will be thrown.
6. Loading Resources with ClassLoader.getResource()
The ClassLoader class also provides a method called getResource(String name) that allows you to load resources (such as configuration files) associated with a class. This method returns a URL object pointing to the resource. Here’s how you can use it:
java ClassLoader classLoader = getClass().getClassLoader(); URL resourceUrl = classLoader.getResource("config.properties");
7. Instantiate Objects with Reflection
Java Reflection not only lets you load classes dynamically but also create instances of classes and invoke their methods. The newInstance() method, present in the Class class, lets you create an instance of a class:
java try { Class<?> dynamicClass = Class.forName("com.example.DynamicClass"); Object instance = dynamicClass.newInstance(); } catch (ClassNotFoundException | InstantiationException | IllegalAccessException e) { e.printStackTrace(); }
8. Accessing Fields and Methods
Reflection also allows you to access fields and methods of a class dynamically. The Field class provides methods like get(Object obj) and set(Object obj, Object value) for manipulating fields:
java try { Class<?> dynamicClass = Class.forName("com.example.DynamicClass"); Object instance = dynamicClass.newInstance(); Field field = dynamicClass.getDeclaredField("fieldName"); field.setAccessible(true); Object value = field.get(instance); Method method = dynamicClass.getDeclaredMethod("methodName"); method.setAccessible(true); method.invoke(instance); } catch (ClassNotFoundException | InstantiationException | IllegalAccessException | NoSuchFieldException | NoSuchMethodException | InvocationTargetException e) { e.printStackTrace(); }
9. Use Cases of Java Reflection and Dynamic Class Loading
The ability to dynamically load and manipulate classes opens up a wide range of use cases, including:
9.1. Plugin Architectures
Reflection and dynamic class loading are often used to implement plugin architectures. With these techniques, you can design applications that allow external modules or plugins to be added without altering the core codebase. The application can dynamically discover and load these plugins at runtime, enhancing extensibility.
9.2. Dependency Injection Frameworks
Frameworks like Spring heavily utilize Java Reflection for dependency injection. By examining class metadata and using dynamic class loading, these frameworks can inject the appropriate dependencies into classes at runtime, reducing the coupling between components.
9.3. Configuration Management
Java applications often rely on configuration files. Reflection enables you to load and process configuration files dynamically, adapting your application’s behavior based on the provided configuration.
9.4. Unit Testing
Reflection can simplify unit testing by allowing you to access private methods and fields of classes during testing. While this practice should be used judiciously, it can be beneficial in certain testing scenarios.
10. Best Practices and Considerations
While Java Reflection and dynamic class loading provide powerful capabilities, they should be used carefully due to potential security and performance concerns:
- Security: Reflection can be misused to bypass access controls and compromise security. Only use reflection when absolutely necessary, and ensure that your code follows best security practices.
- Performance: Reflection operations are slower than direct method invocations or field accesses. If performance is crucial, consider alternative approaches that avoid reflection.
- Type Safety: Since reflection works with raw Object types, it can lead to runtime type errors. Use casting and type checks to mitigate this risk.
- Code Complexity: Excessive use of reflection can lead to complex and hard-to-maintain code. Use it sparingly and consider alternative design patterns when possible.
Conclusion
Java Reflection is a powerful feature that empowers developers to create highly flexible, dynamic, and extensible applications. By allowing the introspection and manipulation of classes, fields, methods, and more at runtime, reflection enables a whole new level of programming possibilities. When combined with dynamic class loading, Java Reflection becomes a cornerstone for building modular, plugin-friendly architectures that can adapt and evolve over time. As you venture into the world of Java programming, don’t overlook the incredible capabilities that reflection brings to the table. Use it wisely, and it will become a valuable tool in your programming arsenal.
So, go ahead and explore the realms of Java Reflection and dynamic class loading. Embrace the power of introspection and flexibility that these techniques offer, and watch your Java applications reach new heights of innovation.
Happy coding!
Table of Contents
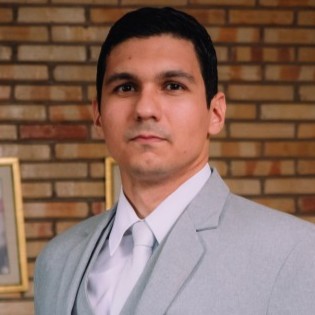
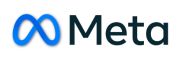