The Art of Building Dynamic Web Applications with Java
When it comes to developing dynamic web applications, Java stands as one of the most popular and powerful languages. Its features such as platform independence, robustness, and simplicity have made it not only a preferred choice among developers but also a top reason for businesses to hire Java developers.
In this blog post, we’ll take a deep dive into how to build dynamic web applications using Java, covering topics such as Servlets, Java Server Pages (JSP), and Java Server Faces (JSF). Whether you’re looking to enhance your skills or are a business looking to hire Java developers, this post can serve as a helpful guide. We’ll walk through the basics and also provide examples to enhance understanding of these key areas of Java web development.
Java Servlets
Java Servlets are server-side programs that handle client requests and return a customized or dynamic response for each request. The dynamic response could be based on user input, server state, or data retrieved from databases or other sources.
Let’s consider a basic Servlet that displays a “Hello, World!” message on the web page.
```java import java.io.*; import javax.servlet.*; import javax.servlet.http.*; public class HelloWorldServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { PrintWriter out = response.getWriter(); out.println("<html><body>"); out.println("<h1>Hello, World!</h1>"); out.println("</body></html>"); } } ```
This Servlet can be mapped to a URL pattern in the `web.xml` file of your application. When this URL is accessed, it triggers the `doGet()` method, generating a dynamic HTML page.
Java Server Pages (JSP)
JSPs are another technology that allows writing dynamic web pages in Java. JSPs are more convenient to write than Servlets because they allow mixing Java and HTML. The server executes the Java code, and it can change the HTML content dynamically.
Here’s an example of a simple JSP:
```jsp <%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html> <html> <head> <meta charset="ISO-8859-1"> <title>Insert title here</title> </head> <body> <h1>Hello, <%= request.getParameter("name") %>!</h1> </body> </html> ```
In this JSP, the `request.getParameter(“name”)` Java code gets executed on the server. It retrieves the “name” parameter from the HTTP request, and this can be used to generate a customized greeting.
JavaServer Faces (JSF)
JSF is a Java web application framework and is a part of the official standard for developing Java EE web applications. JSF includes a set of APIs for representing UI components and managing their state, handling events and input validation, defining page navigation, and supporting internationalization and accessibility.
Below is an example of a basic JSF application that receives a name as an input and displays a customized greeting message.
```java // Managed Bean @ManagedBean @SessionScoped public class HelloWorld { private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getGreeting() { return "Hello, " + name + "!"; } } ``` ```xml <!-- JSF Page --> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://xmlns.jcp.org/jsf/html"> <h:head> <title>Hello World JSF Example</title> </h:head> <h:body> <h:form> Name: <h:inputText value="#{helloWorld.name}" /> <h:commandButton value="Say Hello" action="hello" /> </h:form> </h:body> </html> ```
In this example, the `name` field is bound to an input text box on the form. When the user submits the form, the `helloWorld.name` bean property is updated with the submitted value.
Conclusion
Building dynamic web applications with Java is a multifaceted process that involves a variety of technologies such as Servlets, JSP, and JSF. Businesses seeking to leverage these technologies frequently choose to hire Java developers due to their capability to create efficient, dynamic, and responsive web applications.
These Java technologies offer a blend of power, flexibility, and standardization that’s tough to beat. With the simple examples provided, we aim to illustrate how these technologies collaborate to build a Java web application. While these examples are basic, they can be a starting point for many projects, further underscoring why many businesses opt to hire Java developers.
Our examples serve as a testament to the capabilities of Java web development technologies in the hands of a skilled developer. They offer vast potential for creating advanced, user-friendly web applications, emphasizing the value when you hire Java developers for your projects. Happy coding!
Table of Contents
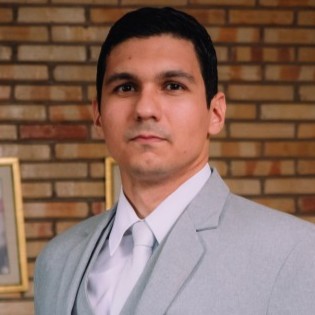
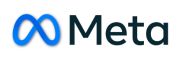