Error-Free Coding: Unlocking Professional Java Exception Handling Techniques
In the realm of programming, the unexpected is always expected. Whether you’re a seasoned developer or a company looking to hire Java developers, everyone involved in the process needs to be prepared to handle every error that might arise. In Java, this is achieved through a concept called ‘exceptions’. Exception handling is a core aspect of robust and fault-tolerant Java code. As we delve into mastering Java exceptions, it becomes clear how pivotal this knowledge is, not only for coding professionals but also when deciding to hire Java developers. Let’s navigate through this complex yet intriguing facet of Java and handle errors like a pro!
What are Java Exceptions?
In Java, an exception is an event that disrupts the regular flow of a program’s instructions during runtime. These abnormal conditions may occur due to several reasons, such as user input errors, hardware failures, or simply because some file you need to open doesn’t exist.
Java exceptions are objects – instances of a class derived from the `Throwable` class. The two main subclasses that branch off from `Throwable` are `Exception` and `Error`. While `Error` class exceptions are thrown by the Java Virtual Machine (JVM) and are generally not meant to be caught by your code, the `Exception` class covers exceptions that your program should handle.
Understanding Try, Catch, and Finally Blocks
Exception handling in Java is achieved using five keywords: `try`, `catch`, `finally`, `throw`, and `throws`. We’ll start with the first three, which work together to handle exceptions.
```java try { // Code that may throw an exception } catch (ExceptionType1 e) { // Code to handle ExceptionType1 } catch (ExceptionType2 e) { // Code to handle ExceptionType2 } finally { // Code to be executed regardless of an exception } ```
In this structure, the `try` block contains the code segment that may throw an exception, while the `catch` block contains the code to handle the exception. Java will attempt to execute the code in the `try` block. If an exception occurs, it will immediately halt execution in that block and pass control to the appropriate `catch` block. If no exceptions are thrown, the `catch` blocks are skipped.
The `finally` block is optional and contains code that is always executed, whether an exception is thrown or not. This block is often used for clean-up activities, such as closing files or network connections.
Throw and Throws Keywords
The `throw` keyword is used to manually throw an exception. For instance, you might be checking user input, and if invalid, you’d want to throw an exception.
```java if (userInput < 0) { throw new IllegalArgumentException("Input cannot be negative"); } ```
On the other hand, the `throws` keyword is used in method signatures to indicate that this method might throw specific exceptions, giving a heads-up to the caller to handle them. It doesn’t actually throw an exception; it only signifies the possibility of an exception being thrown.
```java public void readFile(String file) throws FileNotFoundException { // Reading the file } ```
Custom Exceptions
Java allows the creation of custom exception types to help make your exceptions more descriptive and meaningful. These are just classes that extend the `Exception` class.
```java class CustomException extends Exception { public CustomException(String message) { super(message); } } ```
Best Practices for Exception Handling
- Don’t Suppress Exceptions: Catching an exception and doing nothing is a common pitfall. This can lead to a hard-to-diagnose error.
```java try { // Code that may throw an exception } catch (Exception e) { // Do nothing } ```
This code would be better as:
```java try { // Code that may throw an exception } catch (Exception e) { e.printStackTrace(); } ```
- Catch the Most Specific Exceptions First: Java executes the first matching `catch` block it finds, and won’t check any other `catch` blocks. So, list your `catch` blocks from most specific to most general.
```java try { // Code that may throw an exception } catch (FileNotFoundException e) { // Handle file not found exception } catch (IOException e) { // Handle I/O exception } catch (Exception e) { // Handle all other exceptions } ```
- Don’t Throw `Throwable` or `Exception`: These are too general and make it hard to catch specific exceptions downstream.
```java public void readFile(String file) throws Exception { // Don't do this // Reading the file } ```
- Use `finally` to Clean Up: `finally` blocks should be used to close resources like files, database connections, and network sockets regardless of whether an exception was thrown.
```java FileReader reader = new FileReader("test.txt"); try { // Do something with reader } catch (IOException e) { // Handle I/O exception } finally { if (reader != null) { reader.close(); } } ```
- Use Custom Exceptions for Better Readability: Custom exceptions can make your code much more readable and maintainable.
```java if (order.getTotal() < 0) { throw new InvalidOrderException("Order total cannot be negative"); } ```
Conclusion
Exception handling is a powerful tool in Java that, when used effectively, can greatly enhance the robustness and maintainability of your code. Whether you’re looking to upskill or planning to hire Java developers, understanding different types of exceptions is crucial. By mastering the use of `try`, `catch`, `finally`, `throw`, and `throws`, and following the outlined best practices, you or the Java developers you hire can handle errors like a pro, taking Java programming to the next level. This knowledge makes a significant difference when aiming for high-quality, resilient code. Happy coding!
Table of Contents
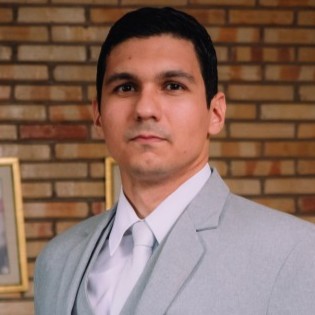
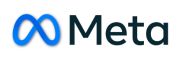