Swing and JavaFX: Crafting User-Friendly Java GUI Applications
Java’s Swing and AWT (Abstract Window Toolkit) libraries have been powerful tools for developing Graphic User Interfaces (GUIs) for a long time. These are the tools proficient Java developers, who are available for hire, use to create robust and user-friendly applications. However, many developers have since switched to JavaFX for a modern touch. This is an important consideration when looking to hire Java developers, as JavaFX experience can bring a modern flare to your project. Throughout this article, we will take a look at the core components of Java’s GUI programming tools and how they can be used to create user-friendly interfaces. By understanding these elements, you can ensure you hire Java developers who are well-equipped to build superior GUI applications.
Introduction to Java GUI
The Graphic User Interface (GUI) is a form of user interface that allows users to interact with electronic devices through graphical icons and audio indicators such as primary notation. Java provides various ways to design a GUI, with some of the most popular libraries being Swing and JavaFX.
Swing, which is a part of Java Foundation Classes (JFC), is used for creating window-based applications. It provides a rich set of widgets and packages to make sophisticated GUI components for Java applications.
JavaFX, on the other hand, is a software platform for creating and delivering desktop applications, as well as for embedded systems and internet browsers. It’s the successor to the Java Swing libraries and provides a simple and more powerful way to design and develop GUI applications in Java.
Let’s take a deeper dive into both.
Swing GUI
To create a simple Swing GUI application, we start by creating a frame. The JFrame class, part of the javax.swing package, is used to create a frame. Let’s look at an example:
```java import javax.swing.JFrame; public class MyWindow extends JFrame { public MyWindow() { setTitle("My First Swing App"); setSize(400, 200); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { MyWindow myWindow = new MyWindow(); myWindow.setVisible(true); } } ```
In the above example, we’re creating a new window with the title “My First Swing App”. The setSize() method is used to set the initial size of the frame, while setDefaultCloseOperation() method ensures that the application exits when the window is closed.
To make the interface more user-friendly, you may want to add components like buttons, checkboxes, text fields, etc. Let’s add a button to our frame:
```java import javax.swing.JFrame; import javax.swing.JButton; public class MyWindow extends JFrame { public MyWindow() { setTitle("My Swing App With Button"); setSize(400, 200); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JButton button = new JButton("Click Me"); this.getContentPane().add(button); } public static void main(String[] args) { MyWindow myWindow = new MyWindow(); myWindow.setVisible(true); } } ```
This code creates a button with the text “Click Me” and adds it to our JFrame. When the window is opened, the button is displayed in it.
JavaFX GUI
JavaFX has been developed to replace Swing as the standard GUI library for Java, as it offers a modern and feature-rich approach for GUI programming. To create a JavaFX application, you extend the javafx.application.Application class and override its start() method.
Here’s an example:
```java import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.stage.Stage; public class MyWindowFX extends Application { @Override public void start(Stage primaryStage) { Button btn = new Button("Click Me"); Scene scene = new Scene(btn, 400, 200); primaryStage.setTitle("My First JavaFX App"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } } ```
In this code, we’re creating a new window with a button just like we did with Swing. But JavaFX uses Stages and Scenes. The Stage class is the top-level JavaFX container, and the Scene class is the container for all content in a scene graph.
The start() method is the main entry point for all JavaFX applications. A Button is created with the text “Click Me”. A Scene is created with the button and given dimensions of 400 x 200 pixels. The Scene is then set on the primaryStage (our window).
Enhancing User Experience
We have created basic windows with a simple button, but that is not enough to make our application user-friendly. Some additional features you might consider adding include:
- Event Handling: Users should be able to interact with GUI components. In Java, we handle this with Event Listeners. Below is an example of how to add a click event listener to a button in JavaFX:
```java Button btn = new Button("Click Me"); btn.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent event) { System.out.println("Button was clicked!"); } }); ```
- Layout Management: Both Swing and JavaFX offer different Layout Managers to arrange components in a particular manner. For example, in JavaFX, we have layouts such as HBox, VBox, GridPane, and more.
- Validation and Error Handling: Proper validation and error handling provide feedback to the user and prevent misuse of the application. For example, you could use JavaFX dialogs to display error messages.
- Consistency and familiarity: Users should be able to predict how your application works. Using common interface elements and keeping your interface consistent improves usability.
- Responsive Design: Your GUI should look good on all devices and screen sizes. JavaFX makes this easier with percentage-based layouts and adaptable font sizes.
Conclusion
Java GUI programming, with Swing or JavaFX, enables developers, particularly those you may wish to hire, to create user-friendly interfaces with rich functionalities. These hired Java developers can proficiently utilize GUI elements such as frames, buttons, and labels to make interactions with the application more intuitive for users. Enhanced features like event handling, layout management, and validation can further improve the user experience.
With the shift towards more modern and user-friendly applications, understanding Java GUI programming’s basic and advanced aspects is crucial for developers. When looking to hire Java developers, their familiarity with both Swing and JavaFX can be key, as while Swing provides a solid foundation, JavaFX brings additional flexibility and power to create sophisticated applications.
By focusing on users’ needs and expectations and leveraging the capabilities of these Java libraries, developers, such as those you might hire, can create highly engaging, interactive, and user-friendly GUI applications.
Table of Contents
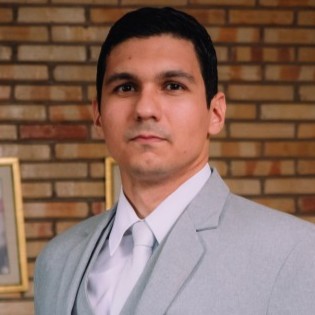
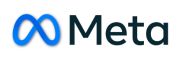