Java Lambda Expressions: Streamlining Functional Programming
Java has evolved significantly over the years, and one of the most notable additions is lambda expressions. Lambda expressions, introduced in Java 8, bring functional programming concepts to the language, allowing developers to write more concise and expressive code. This blog explores how lambda expressions can simplify Java programming and provides practical examples to illustrate their benefits.
Understanding Lambda Expressions
Lambda expressions are a way to create anonymous methods or functions that can be used as arguments to other methods or stored in variables. They enable a more functional style of programming, making it easier to work with collections and perform operations in a more declarative manner.
Using Lambda Expressions in Java
Lambda expressions in Java are used primarily to implement functional interfaces. A functional interface is an interface with a single abstract method. Lambda expressions provide a clear and concise way to represent instances of these interfaces.
Here are some key aspects and examples demonstrating how lambda expressions can be utilized in Java.
1. Basic Syntax of Lambda Expressions
The syntax of a lambda expression consists of the following parts:
– Parameters: The input parameters of the lambda expression.
– Arrow Token (`->`): Separates the parameters from the body.
– Body: The code to be executed.
Example: Simple Lambda Expression
Here’s a basic example of a lambda expression that prints a message.
```java public class LambdaExample { public static void main(String[] args) { Runnable runnable = () -> System.out.println("Hello, Lambda!"); runnable.run(); } } ```
2. Using Lambda Expressions with Collections
Lambda expressions are particularly powerful when working with collections. They can be used with Java’s Stream API to perform operations on collections in a more functional style.
Example: Filtering and Mapping a List
Suppose you have a list of numbers and you want to filter out the even numbers and then square them.
```java import java.util.Arrays; import java.util.List; import java.util.stream.Collectors; public class LambdaWithCollections { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6); List<Integer> squaredEvens = numbers.stream() .filter(n -> n % 2 == 0) .map(n -> n * n) .collect(Collectors.toList()); System.out.println(squaredEvens); // Output: [4, 16, 36] } } ```
3. Implementing Functional Interfaces
Lambda expressions can be used to implement functional interfaces like `Predicate`, `Function`, and `Consumer`.
Example: Using Predicate to Test Conditions
Here’s how you might use a lambda expression with a `Predicate` to test if a number is positive.
```java import java.util.function.Predicate; public class LambdaFunctionalInterfaces { public static void main(String[] args) { Predicate<Integer> isPositive = num -> num > 0; System.out.println(isPositive.test(10)); // Output: true System.out.println(isPositive.test(-5)); // Output: false } } ```
4. Combining Lambda Expressions with Method References
Method references are a shorthand notation of a lambda expression to call a method. They can make your code even more concise.
Example: Using Method References
Here’s an example of using a method reference to print each element of a list.
```java import java.util.Arrays; import java.util.List; public class MethodReferences { public static void main(String[] args) { List<String> names = Arrays.asList("Alice", "Bob", "Charlie"); names.forEach(System.out::println); } } ```
Conclusion
Java lambda expressions provide a powerful way to streamline functional programming in Java. They offer a more concise and expressive way to write code, particularly when dealing with collections and functional interfaces. By leveraging lambda expressions effectively, you can write cleaner, more maintainable code and take full advantage of Java’s functional programming capabilities.
Further Reading:
Table of Contents
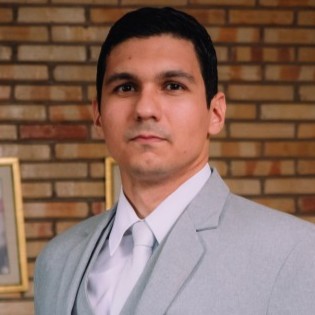
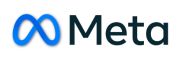