Java Lambdas and Streams: Functional Programming Paradigm
In the world of Java programming, where object-oriented principles have long reigned supreme, a paradigm shift has been unfolding. Enter functional programming, a programming style that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. In this journey towards more concise, readable, and efficient code, Java Lambdas and Streams have emerged as powerful tools. In this blog, we will delve into the functional programming paradigm, understand the significance of Java Lambdas and Streams, and explore how they can be harnessed to elevate your code to new heights.
Table of Contents
1. Understanding Functional Programming
Functional programming is a programming paradigm that revolves around the concept of treating computation as the evaluation of functions. It emphasizes immutability, avoiding side effects, and the use of higher-order functions. In simple terms, functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and returned as values from functions. This enables developers to write more modular, reusable, and expressive code.
2. The Rise of Java Lambdas
2.1. Introducing Lambdas
In Java 8, a game-changing feature was introduced: Lambdas. A lambda expression is a concise representation of an anonymous function that can be passed around like any other variable. It allows you to treat functionality as a method argument or code as data. The syntax for a lambda expression involves parameters, an arrow (->), and an expression or a block of code.
java // Traditional approach Runnable traditionalRunnable = new Runnable() { @Override public void run() { System.out.println("Hello, world!"); } }; // Lambda expression Runnable lambdaRunnable = () -> { System.out.println("Hello, world!"); };
2.2. Benefits of Lambdas
Lambdas bring a multitude of benefits to the table. They enhance code readability, enable a more functional style of programming, and make code more concise by reducing boilerplate. With lambdas, Java code can be more expressive and closer to the problem domain.
3. Unleashing the Power of Streams
3.1. Introducing Streams
In conjunction with lambdas, Java introduced the Stream API. Streams provide a way to process sequences of data elements in a functional style. A stream is not a data structure but rather a sequence of elements that can be processed in parallel or sequentially. Streams allow you to perform operations like filtering, mapping, and reducing on data with incredible ease.
3.2. Pipeline of Stream Operations
A stream operation can be divided into two types: intermediate and terminal operations. Intermediate operations are operations like filter or map that transform a stream into another stream. They are typically lazy, meaning they don’t execute until a terminal operation is invoked. Terminal operations, on the other hand, produce a result or a side effect, such as forEach or collect.
java List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10); int sumOfEvenSquares = numbers.stream() .filter(num -> num % 2 == 0) .map(num -> num * num) .reduce(0, Integer::sum); System.out.println("Sum of squares of even numbers: " + sumOfEvenSquares);
3.3. Parallelism with Streams
One of the standout features of the Stream API is the ease with which you can leverage parallelism. Many operations in the Stream API can be executed in parallel by invoking the parallel() method on a stream. This can lead to significant performance improvements on multi-core processors.
java int sumOfEvenSquaresParallel = numbers.parallelStream() .filter(num -> num % 2 == 0) .map(num -> num * num) .reduce(0, Integer::sum);
4. Embracing a Functional Mindset
Advantages of Functional Programming in Java
The adoption of a functional programming paradigm in Java through Lambdas and Streams brings several advantages:
- Readability: Code becomes more concise and closer to natural language, enhancing readability.
- Concurrent and Parallel Execution: Functional programming encourages immutable data and stateless functions, making it easier to write code that is thread-safe and amenable to parallel execution.
- Modularity: Functions become isolated units of logic, promoting modularity and reusability.
- Testing: Pure functions with no side effects are easier to test and reason about.
- Debugging: Immutability reduces the chances of unexpected state changes, simplifying debugging.
5. When to Use Lambdas and Streams
5.1. Scenarios for Lambdas
Lambdas are particularly useful when:
- Passing behavior as an argument to a method (e.g., sorting a collection with a custom comparator).
- Implementing functional interfaces (interfaces with a single abstract method) like Runnable or Callable.
- Writing concise inline implementations for small tasks.
5.2. Scenarios for Streams
Streams shine in the following scenarios:
- Working with large datasets that require filtering, mapping, and aggregation.
- Simplifying complex operations like groupings, reductions, and transformations.
- Exploiting parallelism for improved performance.
6. Potential Drawbacks and Considerations
6.1. Learning Curve
Adopting the functional programming paradigm, especially for developers accustomed to an object-oriented mindset, can have a learning curve. Understanding the principles of immutability and pure functions might take time.
6.2. Performance Overhead
While streams can provide performance improvements through parallelism, there might be cases where the overhead of setting up parallel streams outweighs the benefits, especially for small datasets.
Conclusion
The Java programming landscape has evolved, and the inclusion of Lambdas and Streams has brought the functional programming paradigm into the spotlight. Embracing this paradigm can lead to more expressive, modular, and efficient code. Lambdas enable the treatment of functionality as a first-class citizen, while Streams provide a powerful mechanism for processing data in a functional style. By adopting a functional mindset, developers can write code that is not only easier to read and maintain but also takes advantage of concurrency and parallelism.
As you embark on your journey with Java Lambdas and Streams, remember that while they introduce powerful tools, their effectiveness depends on understanding when and how to use them appropriately. With practice, you can master the art of functional programming in Java, opening doors to more elegant and efficient solutions to the challenges you face in your coding endeavors.
So, embrace the paradigm shift, experiment with Lambdas and Streams, and elevate your Java programming skills to new horizons. Happy coding!
Table of Contents
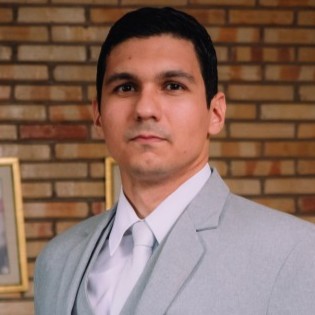
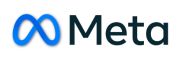