Introduction to JavaFX Layouts: Building Responsive UIs
In the world of modern software development, creating user interfaces that are not only visually appealing but also responsive across various devices and screen sizes is crucial. JavaFX, a powerful framework for building Java applications with graphical user interfaces (GUIs), provides a range of layout options to achieve this goal. In this guide, we’ll delve into the fundamentals of JavaFX layouts and how they can help you build dynamic and responsive UIs for your applications.
Table of Contents
1. Understanding the Importance of Layouts
When it comes to creating user interfaces, layout is the foundation. A well-designed layout ensures that UI elements are positioned correctly and adapt gracefully to different screen sizes. Without proper layouts, your application’s UI might appear distorted or cluttered on certain devices, leading to a poor user experience.
JavaFX addresses this challenge by offering a variety of layout containers, each catering to specific design requirements. These layout containers automatically handle the arrangement and sizing of UI components, making it easier to create responsive interfaces that look and feel consistent across devices.
2. Exploring JavaFX Layout Options
JavaFX provides several layout containers, each designed to handle different scenarios. Let’s explore some of the most commonly used layout containers:
2.1. VBox (Vertical Box):
The VBox layout arranges its child nodes in a single vertical column. This is particularly useful when you want to stack UI elements on top of each other, such as in a settings panel or a list of items.
java VBox vbox = new VBox(); vbox.getChildren().addAll(label1, textField1, button1);
2.2. HBox (Horizontal Box):
Contrary to VBox, the HBox layout places its child nodes in a single horizontal row. It’s great for creating toolbars, button panels, or any scenario where elements need to be aligned horizontally.
java HBox hbox = new HBox(); hbox.getChildren().addAll(button1, button2, button3);
2.3. BorderPane:
The BorderPane layout divides its area into five regions: top, bottom, left, right, and center. This is ideal for creating complex UIs with distinct sections, such as a navigation bar at the top, a status bar at the bottom, and the main content in the center.
java BorderPane borderPane = new BorderPane(); borderPane.setTop(menuBar); borderPane.setCenter(contentArea); borderPane.setBottom(statusBar);
2.4. GridPane:
GridPane allows you to create a grid of rows and columns, where you can place UI elements in specific cells. This is excellent for creating forms and other layouts with a structured grid-like arrangement.
java GridPane gridPane = new GridPane(); gridPane.add(label1, 0, 0); gridPane.add(textField1, 1, 0); gridPane.add(label2, 0, 1); gridPane.add(textField2, 1, 1);
2.5. StackPane:
The StackPane layout stacks its child nodes on top of each other, allowing you to layer UI elements. It’s useful for creating overlays, dialogs, and scenarios where components need to overlap.
java StackPane stackPane = new StackPane(); stackPane.getChildren().addAll(backgroundImage, content);
3. Implementing Responsive Designs
Building responsive UIs means ensuring that your application adapts gracefully to different screen sizes and orientations. JavaFX layouts make this process easier by providing features that enable responsive design:
3.1. Percent Width and Height:
You can set the width and height of layout regions using percentage values. This allows components to take a relative portion of the available space, making them suitable for responsive designs.
java ColumnConstraints col1 = new ColumnConstraints(); col1.setPercentWidth(50);
3.2. Priority:
JavaFX layout containers allow you to set priorities for different regions. This is helpful when dealing with resizable layouts, as it determines which elements will grow or shrink first when the container is resized.
java HBox.setHgrow(button1, Priority.ALWAYS); HBox.setHgrow(button2, Priority.SOMETIMES);
3.3. Alignment:
Proper alignment of UI elements enhances the overall appearance of your UI. JavaFX layouts provide alignment options, ensuring that components are positioned as intended.
java VBox.setVgrow(label1, Priority.ALWAYS); VBox.setMargin(button1, new Insets(10));
4. Bringing It All Together: A Responsive UI Example
Let’s create a simple example to demonstrate the concepts we’ve discussed. We’ll build a settings panel using JavaFX layouts.
java public class SettingsPanel extends VBox { public SettingsPanel() { Label titleLabel = new Label("Settings"); Button saveButton = new Button("Save"); Button cancelButton = new Button("Cancel"); // Configure layout setSpacing(10); setPadding(new Insets(20)); setAlignment(Pos.CENTER); // Add components getChildren().addAll(titleLabel, new Separator(), createOption("Option 1"), createOption("Option 2"), new Separator(), new HBox(saveButton, cancelButton)); } private Node createOption(String optionName) { CheckBox checkBox = new CheckBox(optionName); checkBox.setPadding(new Insets(5)); return checkBox; } }
In this example, we’ve used VBox and HBox layouts to create a settings panel with options and buttons. The layout adjusts itself to the content, and the alignment and spacing are set for better readability.
Conclusion
JavaFX layouts are essential tools for building responsive and dynamic user interfaces in Java applications. By selecting the appropriate layout containers and utilizing responsive design features, you can ensure that your UI looks and behaves as intended across a variety of devices and screen sizes. Experiment with different layouts, explore their capabilities, and combine them to create visually appealing and user-friendly applications.
In the next part of this series, we’ll dive deeper into advanced layout techniques and explore how to style your JavaFX UI components for a polished look.
Remember, a well-designed UI enhances user satisfaction and plays a significant role in the success of your application. So, keep practicing, experimenting, and refining your UI design skills using JavaFX layouts.
Table of Contents
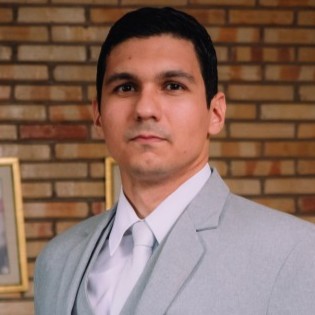
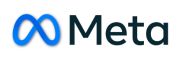