Mastering Functions in Java: A Comprehensive Guide with Practical Examples
Java, a high-level, class-based, object-oriented programming language, has made a significant impact on the software industry. Its robustness, ease of learning, and platform independence make it a powerful tool in the arsenal of many software developers, making it a popular choice for organizations looking to hire Java developers. Java stands strong among other programming languages, bolstered by its diverse functionalities.
In this blog post, we will focus on the functions of Java, delving deep into the nuts and bolts of how these building blocks work. Understanding these functionalities is crucial for those looking to become proficient in Java, whether they are beginners starting their journey or experienced developers wishing to brush up their knowledge. This in-depth understanding of Java’s features not only enriches a developer’s skill set but also makes them more marketable for organizations planning to hire Java developers.
Functions in Java
Functions, also referred to as methods in Java, are blocks of code designed to perform a specific task. They provide a way to structure your code in bite-sized, logical pieces, allowing for code reuse and a more readable, maintainable code base.
The general form of a function in Java is as follows:
```java modifier returnType nameOfMethod (Parameter List){ // method body } ```
The `modifier` signifies the access type for the function, `returnType` is the data type the function returns, `nameOfMethod` is the identifier used to call the function, and `Parameter List` contains the input parameters.
Let’s dig deeper and explore various examples of functions in Java.
Example 1: Basic Function
A simple example to start off with would be a function that adds two numbers:
```java public class Main { static int addNumbers(int a, int b) { return a + b; } public static void main(String[] args) { int sum = addNumbers(10, 20); System.out.println("The sum is: " + sum); } } ```
In this example, `addNumbers` is a function that takes two integer parameters, adds them, and returns the result. In the `main` function, we call `addNumbers` with two integers and print the result.
Example 2: Void Functions
Void functions in Java are those that do not return a value. Instead, they perform an action. Consider the following example:
```java public class Main { static void printName(String name) { System.out.println("Hello, " + name); } public static void main(String[] args) { printName("Alice"); } } ```
In this case, `printName` is a void function that prints a greeting. We call this function in `main` with a string parameter.
Example 3: Recursive Function
A recursive function is a function that calls itself. This is especially useful for tasks that can be divided into simpler, identical tasks. For instance, calculating the factorial of a number:
```java public class Main { static long factorial(int n) { if (n == 1) return 1; else return (n * factorial(n - 1)); } public static void main(String[] args) { long result = factorial(5); System.out.println("The factorial is: " + result); } } ```
In this case, `factorial` is a recursive function that calls itself to calculate the factorial of a number.
Example 4: Functions with Variable Arguments (Varargs)
Java functions can take a variable number of arguments. These are known as varargs and can be particularly useful when you don’t know how many arguments will be passed to the function. Here’s an example:
```java public class Main { static void printNumbers(int... numbers) { for (int number : numbers) { System.out.print(number + " "); } } public static void main(String[] args) { printNumbers(1, 2, 3, 4, 5); } } ```
In this example, `printNumbers` is a function that takes a variable number of integer arguments and prints them. This function uses the enhanced for-loop, a feature in Java used for iterating over arrays or collections.
Example 5: Lambda Functions
Java 8 introduced the concept of Lambda functions, which are anonymous functions that can be used to implement functional programming concepts. Here’s an example of a lambda function that sorts a list of strings based on their length:
```java import java.util.Arrays; import java.util.List; public class Main { public static void main(String[] args) { List<String> words = Arrays.asList("Java", "Python", "C++", "JavaScript", "C"); words.sort((s1, s2) -> Integer.compare(s1.length(), s2.length())); System.out.println(words); } } ```
In this example, the `sort` function is passed a lambda function as its argument. This lambda function is used to sort the list of words by their length.
Conclusion
In Java, functions are essential tools that bring modularity, reusability, and readability to your code. This clarity and efficiency make Java a popular choice for many organizations looking to hire Java developers. Whether you are defining a simple void function, implementing recursive logic, handling an unknown number of arguments with varargs, or venturing into the functional programming paradigm with lambda functions, the power of functions in Java is indisputable.
Understanding and mastering functions is crucial for every Java developer. This knowledge base is often a primary criterion when companies decide to hire Java developers. Regular practice in writing and using different types of functions in Java is recommended to enhance your proficiency, making your programming journey easier, smoother, and more enjoyable. It also makes you a more desirable candidate for organizations looking to hire skilled and dedicated Java developers.
Table of Contents
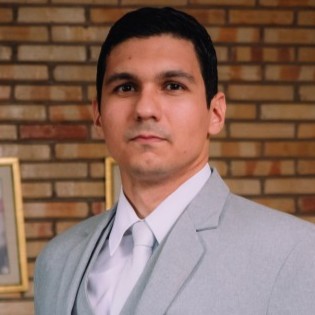
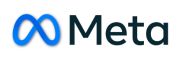