Exploring JavaFX: Building Modern User Interfaces
In the ever-evolving landscape of software development, user interfaces play a pivotal role in capturing user engagement and satisfaction. JavaFX, a powerful framework for building graphical user interfaces (GUIs), has gained significant popularity due to its ability to create modern, visually appealing, and interactive UIs for desktop, mobile, and embedded applications. In this blog, we will embark on a journey to explore the intricacies of JavaFX and learn how to build contemporary user interfaces that seamlessly integrate functionality with aesthetics.
Table of Contents
1. Understanding JavaFX: An Overview
1.1. What is JavaFX?
JavaFX, introduced by Oracle, is a robust framework for creating rich client applications with visually appealing user interfaces. It was designed to replace the older Swing toolkit and provides a wide array of tools and features for UI development. JavaFX allows developers to create cross-platform applications that run on desktops, browsers, and mobile devices with consistent look and feel.
1.2. Features that Set JavaFX Apart
JavaFX comes with several features that make it stand out in the world of UI development:
- Rich Set of UI Controls: JavaFX offers a comprehensive set of built-in UI controls like buttons, labels, text fields, tables, and more, allowing developers to easily create diverse UI elements.
- Scene Graph: JavaFX employs a hierarchical structure called the scene graph to manage and arrange UI components. This makes it easier to handle complex UI layouts.
- CSS Styling: Styling in JavaFX is achieved using CSS (Cascading Style Sheets), enabling developers to apply consistent themes and create visually appealing interfaces.
- 2D and 3D Graphics: JavaFX supports both 2D and 3D graphics, making it suitable for creating not only traditional interfaces but also visually immersive experiences.
- Multimedia Integration: The framework seamlessly integrates multimedia elements like audio and video, enhancing the overall user experience.
3. Getting Started with JavaFX
3.1. Setting Up the Development Environment
Before diving into JavaFX development, ensure that you have Java Development Kit (JDK) 8 or later installed on your system. JavaFX is included in JDK 8 and JDK 11, but for later JDK versions, it’s available as a separate module.
3.2. Creating Your First JavaFX Application
Let’s create a simple “Hello World” JavaFX application to get a taste of how things work:
java import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.stage.Stage; public class HelloWorldApp extends Application { @Override public void start(Stage primaryStage) { Label label = new Label("Hello, JavaFX!"); Scene scene = new Scene(label, 300, 200); primaryStage.setTitle("HelloWorld JavaFX App"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
This minimalistic example demonstrates the fundamental structure of a JavaFX application. We define the UI components, such as a Label, create a Scene to hold them, and set up the primary Stage to display the scene.
4. JavaFX Essentials: Building Blocks of UI
4.1. Stage and Scene
In JavaFX, the primary container for UI elements is the Stage. It represents the main window of the application. Within the Stage, you organize your UI using Scene objects. A Scene holds a hierarchical tree of nodes, which are the building blocks of your UI.
4.2. Nodes: Layouts and Controls
Nodes are the visual components that make up your UI. JavaFX provides a variety of nodes, categorized into two main groups: layout nodes and control nodes. Layout nodes are used to arrange and manage the positioning of other nodes, while control nodes are interactive elements like buttons and text fields.
java import javafx.scene.layout.*; import javafx.scene.control.*; public class NodeExampleApp extends Application { @Override public void start(Stage primaryStage) { // Using VBox as a layout node VBox vbox = new VBox(); // Adding control nodes Button button = new Button("Click Me"); TextField textField = new TextField(); vbox.getChildren().addAll(button, textField); Scene scene = new Scene(vbox, 400, 300); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
4.3. Styling with CSS
JavaFX allows you to style your UI using CSS, enabling you to achieve a consistent and visually pleasing design across your application. Here’s a simple example of applying CSS styles to JavaFX nodes:
java import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.stage.Stage; public class StylingExampleApp extends Application { @Override public void start(Stage primaryStage) { Button styledButton = new Button("Styled Button"); styledButton.getStyleClass().add("styled-button"); Scene scene = new Scene(styledButton, 300, 200); scene.getStylesheets().add("styles.css"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
In this example, the styled-button style class is defined in an external CSS file named styles.css.
5. Designing Modern UIs with JavaFX
5.1. Material Design Principles
JavaFX enables you to create modern UIs by incorporating design principles like Google’s Material Design. Material Design emphasizes visual hierarchy, consistent typography, and meaningful motion. By adhering to these principles, you can make your JavaFX application look and feel contemporary.
5.2. Responsive and Adaptive UI
With the increasing variety of screen sizes and devices, creating responsive and adaptive UIs has become crucial. JavaFX provides tools to create UIs that automatically adapt to different screen sizes and orientations. The Region class and its subclasses offer features like layout constraints and responsiveness.
java import javafx.scene.layout.*; import javafx.scene.control.Label; import javafx.stage.Stage; public class ResponsiveUIApp extends Application { @Override public void start(Stage primaryStage) { BorderPane root = new BorderPane(); Label centerLabel = new Label("Center"); root.setCenter(centerLabel); Scene scene = new Scene(root, 800, 600); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
6. Enhancing User Experience
6.1. Animation and Effects
JavaFX provides powerful animation and visual effects capabilities. You can create smooth animations to enhance the user experience and provide visual feedback. The Timeline class is often used to define animations that change over time.
java import javafx.animation.*; import javafx.scene.shape.Circle; import javafx.stage.Stage; public class AnimationExampleApp extends Application { @Override public void start(Stage primaryStage) { Circle circle = new Circle(50, 50, 30); TranslateTransition translate = new TranslateTransition(Duration.seconds(2), circle); translate.setByX(200); translate.setCycleCount(Animation.INDEFINITE); translate.setAutoReverse(true); translate.play(); Group root = new Group(circle); Scene scene = new Scene(root, 400, 300); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
6.2. Transitions and Keyframes
Transitions and keyframes allow you to create complex animations by specifying intermediate states for your UI elements. Keyframes define the values of properties at specific points in time, and transitions interpolate between these keyframes.
7. Exploring Advanced JavaFX Concepts
7.1. JavaFX 3D Graphics
JavaFX isn’t limited to 2D graphics. It offers built-in support for 3D graphics, enabling you to create immersive 3D environments and experiences within your applications.
7.2. FXML: Declarative UI Design
FXML is an XML-based language that allows you to define your UI layouts in a declarative manner. This approach separates the UI design from the application logic, promoting better code organization and collaboration between designers and developers.
7.3. Custom Controls and Components
JavaFX lets you create custom controls and components by extending existing ones or building them from scratch. This enables you to tailor your UI to your application’s specific needs.
Conclusion
JavaFX has emerged as a versatile and powerful framework for building modern user interfaces in Java applications. Its wide array of features, from rich UI controls to styling capabilities, animation, and 3D graphics, empowers developers to create engaging and visually appealing applications across various platforms. By understanding the fundamental building blocks, design principles, and advanced concepts of JavaFX, you can unlock the potential to craft user interfaces that seamlessly blend form and function, providing exceptional user experiences in your applications. So, embark on your JavaFX journey, and start building captivating user interfaces that captivate and delight users.
Table of Contents
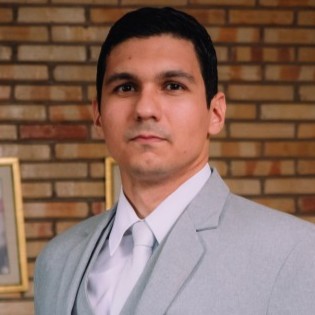
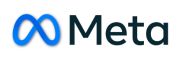