Java and the Four Pillars of Object-Oriented Programming: A Comprehensive Exploration
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of ‘objects’. Objects are entities that hold data, in the form of fields (often known as attributes), and code, in the form of procedures (also known as methods). Java, being an object-oriented language, encapsulates the OOP principles into its core. This characteristic makes Java an ideal choice for developing flexible and maintainable code. Consequently, businesses looking to scale up their software infrastructure often choose to hire Java developers who are proficient in applying these OOP principles in real-world scenarios.
Principles of Object-Oriented Programming
Four fundamental principles define Object-Oriented Programming – encapsulation, inheritance, polymorphism, and abstraction. Let’s dig deeper into each of these principles using Java examples.
1. Encapsulation
Encapsulation is the mechanism of bundling the data and the methods that operate on the data into a single unit. In Java, a ‘class’ provides the blueprint for encapsulation.
Consider this example of a ‘Book’ class:
```java public class Book { private String title; private String author; private double price; public Book(String title, String author, double price) { this.title = title; this.author = author; this.price = price; } public String getTitle() { return title; } public String getAuthor() { return author; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } } ```
Here, the attributes `title`, `author`, and `price` are encapsulated in the `Book` class. The methods `getTitle`, `getAuthor`, `getPrice`, and `setPrice` provide the interface to access and modify these attributes, ensuring that the attributes are safe from unauthorized access and modification.
2. Inheritance
Inheritance is a principle that allows a class to inherit the fields and methods of another class. This promotes code reusability and a logical, hierarchical class structure. In Java, inheritance is achieved using the ‘extends‘ keyword.
For example, consider a ‘TextBook’ class that inherits from the ‘Book’ class:
```java public class TextBook extends Book { private String subject; public TextBook(String title, String author, double price, String subject) { super(title, author, price); this.subject = subject; } public String getSubject() { return subject; } public void setSubject(String subject) { this.subject = subject; } } ```
In this example, `TextBook` inherits all fields and methods from the `Book` class and adds an additional field `subject` and corresponding methods. Any instance of `TextBook` will now have all properties of a `Book`, plus its `subject`.
3. Polymorphism
Polymorphism is the ability of an object to take on many forms. In Java, polymorphism is achieved by using method overriding and interfaces.
Consider the following example with a ‘Printable’ interface and two classes ‘Book’ and ‘Magazine’ implementing this interface:
```java public interface Printable { void printDetails(); } public class Book implements Printable { private String title; private String author; // constructors and getters setters go here @Override public void printDetails() { System.out.println("Book{" + "title='" + title + '\'' + ", author='" + author + '\'' + '}'); } } public class Magazine implements Printable { private String name; private String publisher; // constructors and getters setters go here @Override public void printDetails() { System.out.println("Magazine{" + "name='" + name + '\'' + ", publisher='" + publisher + '\'' + '}'); } } ```
In this example, `Book` and `Magazine` classes implement the `Printable` interface, and both provide their implementation of the `printDetails` method. This means that both `Book` and `Magazine` objects can be used wherever a `Printable` object is expected, thereby allowing the object to take on many forms.
4. Abstraction
Abstraction is a principle that focuses on hiding the complex details and showing only the necessary information. In Java, abstraction is achieved by using abstract classes and interfaces.
Consider the following example with a ‘Vehicle’ abstract class and two classes ‘Car’ and ‘Bike’ extending this class:
```java public abstract class Vehicle { abstract void startEngine(); } public class Car extends Vehicle { @Override void startEngine() { System.out.println("Starting the car engine..."); } } public class Bike extends Vehicle { @Override void startEngine() { System.out.println("Starting the bike engine..."); } } ```
In this example, `Vehicle` is an abstract class with an abstract method `startEngine`. The classes `Car` and `Bike` extend `Vehicle` and provide their implementation of the `startEngine` method. Thus, the client classes can use `Vehicle` objects without knowing their actual implementation, thereby promoting abstraction.
Advantages of OOP in Java
The use of OOP in Java offers several advantages:
- Code Reusability and Recycling: Classes created once can be reused in multiple programs. This reduces code redundancy and increases code efficiency.
- Improved Code Maintenance: Object-oriented programs are easy to understand and modify, making them more maintainable.
- Faster Development: Reuse enables faster development as existing components can be adapted for use.
- Better Productivity: With inheritance, programmers can build on the existing codebase, improving their productivity.
- Enhanced Security: Encapsulation provides data hiding, preventing unauthorized access to class members.
Conclusion
Object-Oriented Programming is a core aspect of Java programming. The four principles of OOP – encapsulation, inheritance, polymorphism, and abstraction – provide a strong framework for organizing and structuring software programs. This mastery of these principles leads to code that is more efficient, maintainable, secure, and flexible. It’s precisely this proficiency that you should seek when looking to hire Java developers. After all, Java and OOP together have been, and continue to be, a powerful combination in software development. Therefore, hiring Java developers well-versed in these principles will bring invaluable skills to your project or team.
Table of Contents
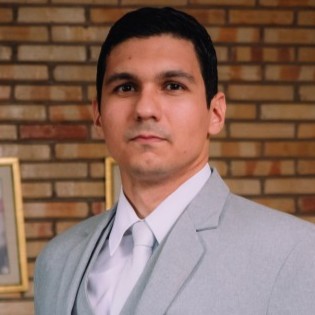
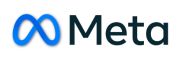