Building RESTful APIs with Java and Spring Boot
In today’s world of software development, building robust and efficient APIs is a critical skill. Representational State Transfer (REST) has emerged as the standard architectural style for designing networked applications. Java, being a versatile and widely-used programming language, along with the Spring Boot framework, provides a powerful combination for creating RESTful APIs that are scalable, maintainable, and secure. In this guide, we’ll take you through the process of building RESTful APIs with Java and Spring Boot, covering everything from the basics to advanced topics.
Table of Contents
1. Introduction to RESTful APIs
1.1. What are RESTful APIs?
A RESTful API (Application Programming Interface) is an architectural style for designing networked applications based on the principles of Representational State Transfer (REST). It enables communication between different software systems over standard HTTP methods. REST APIs are designed around resources and use standard HTTP verbs (GET, POST, PUT, DELETE) to perform operations on these resources. This design makes them scalable, stateless, and easy to understand.
1.2. Why use Java and Spring Boot?
Java is known for its platform independence, strong community support, and a wide range of libraries and frameworks. Spring Boot, a project under the Spring Framework umbrella, simplifies the development of Java applications by providing a cohesive, opinionated set of tools and libraries. It helps streamline the creation of RESTful APIs by offering features like dependency injection, automatic configuration, and built-in security. Spring Boot’s integrated development environment, along with its robust ecosystem, makes it an excellent choice for building RESTful APIs.
2. Setting Up Your Development Environment
2.1. Installing Java and Spring Boot
Before you start building RESTful APIs with Java and Spring Boot, you need to set up your development environment. First, ensure that you have Java Development Kit (JDK) 8 or later installed on your system. You can download it from the official Oracle website or use OpenJDK, an open-source alternative.
Once Java is installed, you can proceed to install Spring Boot. Spring Boot can be easily installed using tools like Maven or Gradle, or you can use the Spring Initializr web-based tool to generate a project structure.
2.2. Creating a New Spring Boot Project
To create a new Spring Boot project, follow these steps:
2.2.1. Using Spring Initializr:
- Open your web browser and go to the Spring Initializr website.
- Select the project options, such as language (Java), Spring Boot version, and packaging (JAR or WAR).
- Choose the necessary dependencies, including “Spring Web” for building web applications.
- Click on the “Generate” button to download a zip file containing your project template.
2.2.2. Using Spring Tool Suite (STS) or IntelliJ IDEA:
If you’re using an integrated development environment (IDE) like STS or IntelliJ IDEA, you can create a new Spring Boot project directly from the IDE’s interface.
Select the project options and dependencies as mentioned earlier.
The IDE will generate the project structure for you.
Once you have your Spring Boot project set up, you’re ready to start building your first API endpoint.
3. Creating Your First API Endpoint
3.1. Defining the Project Structure
Before we dive into coding, let’s understand the basic project structure:
css my-rest-api/ ??? src/ ? ??? main/ ? ? ??? java/ ? ? ? ??? com/ ? ? ? ??? myapp/ ? ? ? ??? MyRestApiApplication.java ? ? ? ??? controller/ ? ? ? ? ??? ApiController.java ? ? ? ??? model/ ? ? ? ??? Product.java ? ? ??? resources/ ? ? ??? application.properties ? ??? test/ ? ??? java/ ? ??? com/ ? ??? myapp/ ? ??? controller/ ? ??? ApiControllerTest.java ??? pom.xml
In this example, MyRestApiApplication.java is the main application class, ApiController.java is where we’ll define our API endpoints, and Product.java is a simple model class.
3.2. Implementing a Simple GET Endpoint
Let’s create a simple API endpoint that returns a list of products. Open ApiController.java and define the following code:
java package com.myapp.controller; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; import java.util.ArrayList; import java.util.List; @RestController public class ApiController { @GetMapping("/products") public List<String> getProducts() { List<String> products = new ArrayList<>(); products.add("Product 1"); products.add("Product 2"); products.add("Product 3"); return products; } }
In this code, we’ve annotated the ApiController class with @RestController, indicating that this class handles web requests and produces JSON responses. The getProducts() method is annotated with @GetMapping(“/products”), which maps the method to the URL path “/products” and associates it with the HTTP GET request method.
3.3. Testing the Endpoint with cURL
With the endpoint implemented, let’s test it using the command-line tool cURL:
bash curl http://localhost:8080/products
You should receive a JSON response containing the list of products:
json ["Product 1","Product 2","Product 3"]
Congratulations! You’ve created your first API endpoint using Spring Boot.
4. Request and Response Formats
4.1. Handling Different HTTP Methods
RESTful APIs support various HTTP methods, such as GET, POST, PUT, and DELETE, to perform different actions on resources. Spring Boot’s @RequestMapping annotation, along with method-specific annotations, makes it easy to define endpoints for different HTTP methods.
Let’s add a method to our ApiController to handle POST requests for creating new products:
java @PostMapping("/products") public ResponseEntity<String> createProduct(@RequestBody String productName) { // Logic to create the product return ResponseEntity.status(HttpStatus.CREATED).body("Product created: " + productName); }
In this example, we’ve used @PostMapping(“/products”) to map the method to the URL path “/products” for POST requests. The @RequestBody annotation indicates that the productName parameter should be extracted from the request body.
4.2. Sending and Receiving JSON Data
JSON (JavaScript Object Notation) is a common format for data exchange in RESTful APIs. Spring Boot’s built-in Jackson library automatically converts Java objects to JSON and vice versa.
Let’s modify our Product class and update the createProduct() method to work with JSON data:
java public class Product { private String name; // getters and setters } @PostMapping("/products") public ResponseEntity<Product> createProduct(@RequestBody Product product) { // Logic to create the product return ResponseEntity.status(HttpStatus.CREATED).body(product); }
Now, when you send a POST request with JSON data like this:
json { "name": "New Product" }
You’ll receive a JSON response with the created product.
4.3. Handling Errors and Status Codes
In a real-world API, it’s important to handle errors gracefully and provide meaningful responses. Spring Boot allows you to customize error handling and return appropriate HTTP status codes and error messages.
java @ExceptionHandler(Exception.class) public ResponseEntity<String> handleException(Exception ex) { return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("An error occurred"); }
In this code, the @ExceptionHandler annotation is used to handle exceptions of type Exception.class. The method returns an error response with the status code 500 (Internal Server Error) and a simple error message.
5. Building a Complete CRUD API
5.1. Implementing POST, PUT, and DELETE Endpoints
A complete API often includes CRUD (Create, Read, Update, Delete) operations for resources. Let’s enhance our ApiController to include methods for updating and deleting products:
java @PutMapping("/products/{productId}") public ResponseEntity<String> updateProduct(@PathVariable String productId, @RequestBody Product updatedProduct) { // Logic to update the product return ResponseEntity.ok("Product updated: " + updatedProduct.getName()); } @DeleteMapping("/products/{productId}") public ResponseEntity<String> deleteProduct(@PathVariable String productId) { // Logic to delete the product return ResponseEntity.ok("Product deleted"); }
In the updateProduct() method, we use @PutMapping to handle HTTP PUT requests for updating products. The @PathVariable annotation extracts the {productId} parameter from the URL.
The deleteProduct() method uses @DeleteMapping to handle HTTP DELETE requests for deleting products.
5.2. Validating Request Data
Data validation is crucial to ensure the integrity of your API’s data. Spring Boot provides validation annotations that you can use to validate request data.
java @PostMapping("/products") public ResponseEntity<String> createProduct(@Valid @RequestBody Product product) { // Logic to create the product return ResponseEntity.status(HttpStatus.CREATED).body("Product created: " + product.getName()); }
By adding @Valid before the @RequestBody annotation, you enable automatic validation for the Product object. If validation fails, Spring Boot will automatically return an error response with the appropriate status code and error details.
5.3. Connecting to a Database with Spring Data JPA
To make your API more useful, you’ll likely want to store data in a database. Spring Boot’s integration with Spring Data JPA simplifies database operations by providing a higher-level abstraction over JDBC.
Define a JPA entity class representing your data, such as ProductEntity.
java @Entity public class ProductEntity { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; // getters and setters }
Create a JPA repository interface for the entity.
java public interface ProductRepository extends JpaRepository<ProductEntity, Long> { }
Update your controller methods to use the repository for database operations.
java @Autowired private ProductRepository productRepository; @PostMapping("/products") public ResponseEntity<ProductEntity> createProduct(@Valid @RequestBody ProductEntity product) { ProductEntity createdProduct = productRepository.save(product); return ResponseEntity.status(HttpStatus.CREATED).body(createdProduct); } @GetMapping("/products/{productId}") public ResponseEntity<ProductEntity> getProduct(@PathVariable Long productId) { Optional<ProductEntity> product = productRepository.findById(productId); return product.map(ResponseEntity::ok).orElse(ResponseEntity.notFound().build()); } // Similarly, update other methods to use the repository
By connecting your API to a database, you’ve completed the essential components of a CRUD API with Spring Boot.
Conclusion
In this comprehensive guide, we’ve covered the essentials of building RESTful APIs with Java and Spring Boot. Starting from the basics of setting up your development environment, we explored creating endpoints, handling different HTTP methods, sending and receiving JSON data, and implementing complete CRUD operations.
With Spring Boot’s powerful features and extensive ecosystem, you have the tools to create robust, maintainable, and secure APIs that can handle a variety of use cases. As you continue your journey in API development, consider exploring advanced topics such as authentication, versioning, testing, and deployment to enhance the quality and scalability of your APIs.
Table of Contents
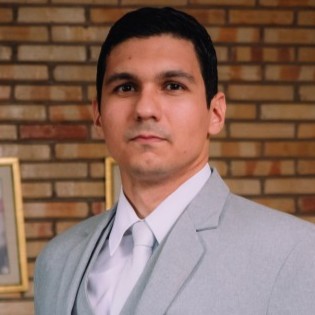
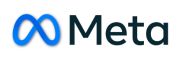