Java Swing: Creating Desktop Applications
In the world of software development, desktop applications remain a fundamental aspect of various industries, offering powerful and feature-rich user experiences. Java Swing, a graphical user interface (GUI) toolkit for Java, has been a cornerstone for creating such applications. In this article, we will delve into the realm of Java Swing, exploring its capabilities, benefits, and providing step-by-step guidance along with code samples to create compelling desktop applications.
Table of Contents
1. Understanding Java Swing
Java Swing, often simply referred to as Swing, is a robust GUI toolkit bundled with the Java Standard Edition platform. It provides a comprehensive set of components for creating graphical user interfaces for desktop applications. Swing is built on top of the Abstract Window Toolkit (AWT), which enables developers to create platform-independent and visually appealing interfaces.
2. Benefits of Using Java Swing
Swing offers a range of advantages that have contributed to its popularity over the years:
- Platform Independence: Swing applications are written in Java, making them inherently platform-independent. A Swing application developed on one platform can run on various operating systems without modification.
- Rich Set of Components: Swing provides an extensive library of components, including buttons, text fields, labels, tables, and more. This allows developers to create complex and interactive interfaces with ease.
- Customizability: Developers can customize the look and feel of Swing components using pluggable look-and-feel (PLAF) themes. This enables applications to match the native appearance of the underlying operating system.
- MVC Architecture: Swing encourages the Model-View-Controller (MVC) design pattern, which promotes separation of concerns. This makes it easier to manage application logic, user interface, and data representation separately.
- Event Handling: Swing incorporates a robust event-handling mechanism. Components can listen for events and respond accordingly, enabling user interactions and dynamic behavior.
- Extensibility: Developers can extend existing Swing components or create custom components, allowing for the implementation of unique and specialized features.
3. Getting Started with Java Swing
To start creating desktop applications with Java Swing, follow these steps:
Step 1: Setting Up Your Development Environment
Ensure that you have the Java Development Kit (JDK) installed on your system. Swing is included in the standard Java library, so no additional setup is required.
Step 2: Creating a Swing Application
Begin by creating a new Java project in your preferred Integrated Development Environment (IDE). Within the project, you can create a new class that will serve as your main application class.
java import javax.swing.*; public class SwingApplication { public static void main(String[] args) { // Create and show the GUI on the Event Dispatch Thread SwingUtilities.invokeLater(() -> { createAndShowGUI(); }); } private static void createAndShowGUI() { // Create a JFrame (main window) JFrame frame = new JFrame("Java Swing Application"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Add components and customize the interface // ... // Pack and display the window frame.pack(); frame.setVisible(true); } }
Step 3: Adding Components
Within the createAndShowGUI method, you can add various Swing components to your application’s main window. For example, adding a JButton:
java JButton button = new JButton("Click Me"); frame.getContentPane().add(button);
Step 4: Running the Application
Run your application. You should see a simple window with the “Click Me” button. This demonstrates the basic structure of a Swing application.
4. Creating Interactive Interfaces
Swing allows you to create interactive interfaces by handling user events. Here’s how you can respond to a button click event:
java button.addActionListener(e -> { JOptionPane.showMessageDialog(frame, "Button Clicked!"); });
In this example, when the button is clicked, a dialog box will appear with the message “Button Clicked!”
5. Layout Management
Layout management is crucial for arranging components within a container. Swing offers various layout managers to help you design your interface effectively. Some common layout managers include:
FlowLayout: Arranges components in a linear fashion, either horizontally or vertically.
BorderLayout: Divides the container into five regions: north, south, east, west, and center.
GridLayout: Organizes components in a grid with rows and columns.
GridBagLayout: Provides more complex grid-based layout options.
java // Using BorderLayout frame.setLayout(new BorderLayout()); frame.add(new JButton("North"), BorderLayout.NORTH); frame.add(new JButton("South"), BorderLayout.SOUTH); frame.add(new JButton("East"), BorderLayout.EAST); frame.add(new JButton("West"), BorderLayout.WEST); frame.add(new JButton("Center"), BorderLayout.CENTER);
6. Advanced Components and Customization
Swing offers advanced components like JTable, JTree, and JFileChooser for handling tables, trees, and file selection, respectively. Additionally, you can customize the appearance of components using PLAF themes.
java // Using JTable to display data String[][] data = {{"Alice", "25"}, {"Bob", "30"}, {"Carol", "28"}}; String[] columns = {"Name", "Age"}; JTable table = new JTable(data, columns); frame.getContentPane().add(new JScrollPane(table));
7. Deploying Swing Applications
Once your Swing application is complete, you can package it for distribution. This typically involves creating executable JAR files that contain all the necessary resources and libraries. Most IDEs offer options to export your project as a JAR file.
Conclusion
Java Swing remains a powerful toolkit for creating desktop applications with sophisticated user interfaces. Its platform independence, rich set of components, and customization options make it a preferred choice for developers. By understanding the fundamentals of Swing and exploring its features, you can embark on a journey to build dynamic and engaging desktop applications that cater to diverse user needs. So, start experimenting with Java Swing and unlock the potential to craft stunning desktop experiences.
Table of Contents
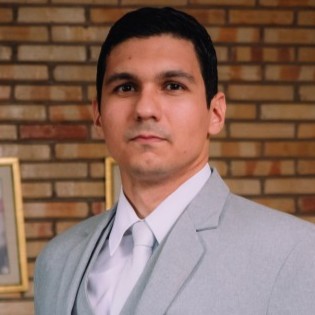
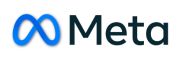