Introduction to Java EE: Enterprise Development Made Easy
Java Enterprise Edition (Java EE) is a powerful platform designed to simplify the development of large-scale, distributed, and scalable enterprise applications. With its extensive set of specifications, libraries, and tools, Java EE provides a robust framework for building enterprise-grade applications that meet the complex needs of modern businesses.
Key Components of Java EE
Java EE is composed of various APIs and services that address different aspects of enterprise application development. Below, we explore some of the core components that make Java EE a preferred choice for enterprise development.
1. Enterprise JavaBeans (EJB): Simplifying Business Logic
Enterprise JavaBeans (EJB) is a server-side component architecture that enables the development of modular, reusable, and scalable business logic. EJBs manage transactions, security, and concurrency, making it easier to focus on the core functionality of your application.
Example: A Simple Stateless Session Bean
Here’s an example of a basic Stateless Session Bean in Java EE:
```java import javax.ejb.Stateless; @Stateless public class CalculatorBean { public int add(int a, int b) { return a + b; } public int subtract(int a, int b) { return a - b; } } ```
In this example, the `CalculatorBean` is a stateless session bean that provides basic arithmetic operations.
2. Java Persistence API (JPA): Managing Data Persistence
The Java Persistence API (JPA) simplifies database interactions by providing an object-relational mapping (ORM) framework. JPA allows developers to work with relational data in an object-oriented manner, reducing the complexity of database operations.
Example: Defining an Entity with JPA
Here’s how you can define a simple JPA entity:
```java import javax.persistence.Entity; import javax.persistence.Id; @Entity public class Employee { @Id private Long id; private String name; private String department; // Getters and setters } ```
This `Employee` class is mapped to a database table, where each instance corresponds to a row in the table.
3. JavaServer Faces (JSF): Building User Interfaces
JavaServer Faces (JSF) is a web application framework that simplifies the development of user interfaces for Java EE applications. JSF provides reusable UI components and an event-driven programming model, making it easier to build complex web applications.
Example: A Simple JSF Page
Below is a basic JSF page that displays a form for user input:
```xml <h:form> <h:outputLabel value="Enter your name:" /> <h:inputText value="#{userBean.name}" /> <h:commandButton value="Submit" action="#{userBean.submit}" /> </h:form> ```
This example shows a simple form that binds to a managed bean (`userBean`) for processing user input.
4. Java Message Service (JMS): Asynchronous Communication
Java Message Service (JMS) enables the development of distributed, loosely coupled, and reliable applications through messaging. JMS allows different parts of an application to communicate asynchronously, enhancing the scalability and reliability of the system.
Example: Sending a Message with JMS
Here’s how you can send a simple message using JMS:
```java import javax.jms.Connection; import javax.jms.ConnectionFactory; import javax.jms.MessageProducer; import javax.jms.Queue; import javax.jms.Session; import javax.jms.TextMessage; import javax.naming.InitialContext; public class JMSSender { public static void main(String[] args) throws Exception { InitialContext ctx = new InitialContext(); ConnectionFactory connectionFactory = (ConnectionFactory) ctx.lookup("jms/ConnectionFactory"); Queue queue = (Queue) ctx.lookup("jms/Queue"); Connection connection = connectionFactory.createConnection(); Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE); MessageProducer producer = session.createProducer(queue); TextMessage message = session.createTextMessage("Hello, JMS!"); producer.send(message); connection.close(); } } ```
This example demonstrates how to send a text message to a JMS queue.
5. Java API for RESTful Web Services (JAX-RS): Creating RESTful Services
JAX-RS is a Java EE API that simplifies the development of RESTful web services. It provides annotations and interfaces that allow developers to quickly create and deploy RESTful endpoints.
Example: A Simple RESTful Service with JAX-RS
Here’s an example of a basic RESTful service using JAX-RS:
```java import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; @Path("/hello") public class HelloService { @GET @Produces(MediaType.TEXT_PLAIN) public String sayHello() { return "Hello, World!"; } } ```
In this example, the `HelloService` class defines a REST endpoint that returns a simple text response.
Conclusion
Java EE is a comprehensive platform that simplifies enterprise application development. With its rich set of APIs and tools, developers can build scalable, secure, and maintainable applications that meet the demands of modern businesses. By leveraging components like EJB, JPA, JSF, JMS, and JAX-RS, you can streamline your development process and deliver robust enterprise solutions.
Further Reading:
Table of Contents
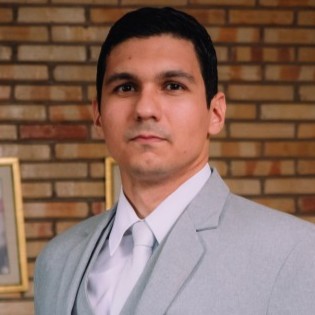
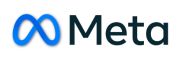