Java Web Services: Building SOAP and RESTful APIs
In the realm of web development, building robust and efficient APIs (Application Programming Interfaces) is crucial for facilitating communication between different software applications. Two common approaches to building APIs in Java are SOAP (Simple Object Access Protocol) and REST (Representational State Transfer). In this post, we’ll delve into the concepts of SOAP and RESTful APIs, explore their differences, and provide examples of how to implement them in Java.
Understanding SOAP and RESTful APIs
SOAP and RESTful APIs serve the same purpose of enabling communication between different systems, but they differ significantly in their architecture and approach.
SOAP
SOAP is a protocol for exchanging structured information in the implementation of web services. It relies on XML (eXtensible Markup Language) for message format and typically operates over HTTP or SMTP (Simple Mail Transfer Protocol). SOAP APIs are known for their strict adherence to standards and support for complex operations such as transactions and security.
RESTful
REST, on the other hand, is an architectural style for designing networked applications. It uses simple HTTP protocols for communication and typically operates over HTTP. RESTful APIs are characterized by their statelessness, scalability, and ease of use. They often leverage common HTTP methods like GET, POST, PUT, and DELETE for CRUD (Create, Read, Update, Delete) operations.
Building SOAP APIs in Java
To illustrate how to build SOAP APIs in Java, let’s consider a simple example of a currency conversion service. We’ll use the JAX-WS (Java API for XML Web Services) framework, which provides tools for building SOAP-based web services.
1. Define the Service Interface
import javax.jws.WebService; @WebService public interface CurrencyConverter { double convertUSDToEUR(double amount); }
2. Implement the Service
@WebService(endpointInterface = "com.example.CurrencyConverter") public class CurrencyConverterImpl implements CurrencyConverter { public double convertUSDToEUR(double amount) { // Perform currency conversion logic return amount * 0.83; // Example conversion rate } }
3. Publish the Service
import javax.xml.ws.Endpoint; public class CurrencyConverterPublisher { public static void main(String[] args) { Endpoint.publish("http://localhost:8080/currency-converter", new CurrencyConverterImpl()); } }
Building RESTful APIs in Java
Now, let’s demonstrate how to build RESTful APIs in Java using the Spring framework. We’ll create a simple CRUD API for managing tasks.
1. Define the Task Entity
public class Task { private Long id; private String title; private boolean completed; // Getters and setters }
2. Implement the Controller
import org.springframework.web.bind.annotation.*; @RestController @RequestMapping("/tasks") public class TaskController { @GetMapping("/{id}") public Task getTaskById(@PathVariable Long id) { // Retrieve task from database } @PostMapping public void createTask(@RequestBody Task task) { // Create task in database } @PutMapping("/{id}") public void updateTask(@PathVariable Long id, @RequestBody Task task) { // Update task in database } @DeleteMapping("/{id}") public void deleteTask(@PathVariable Long id) { // Delete task from database } }
3. Configure Spring Boot Application
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class TaskManagerApplication { public static void main(String[] args) { SpringApplication.run(TaskManagerApplication.class, args); } }
Conclusion
In this post, we’ve explored the concepts of SOAP and RESTful APIs and provided examples of how to implement them in Java. While SOAP offers a robust and standardized approach to web services, RESTful APIs are simpler, more flexible, and widely adopted in modern web development.
By understanding the differences between SOAP and REST, and knowing how to implement them effectively in Java, developers can choose the most suitable approach for their specific project requirements.
External Resources
Table of Contents
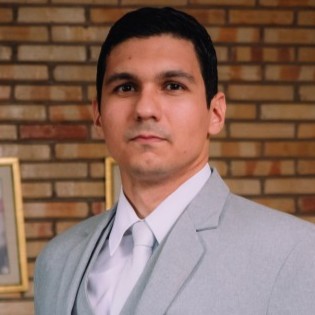
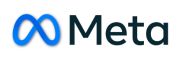