Improving Code Readability with Named JavaScript Functions
Code readability is a crucial aspect of software development. Readable code not only makes it easier for developers to understand and maintain the codebase but also contributes to better collaboration and reduced error rates. JavaScript, being one of the most widely used programming languages, demands special attention to readability due to its dynamic and flexible nature. One effective technique to enhance code readability in JavaScript is the use of named functions. In this article, we’ll delve into the importance of named functions and explore how they can significantly improve the clarity and maintainability of your JavaScript code.
Table of Contents
1. The Significance of Code Readability
Before we delve into the specifics of named functions, let’s briefly discuss why code readability is so important. As software projects grow in size and complexity, maintaining and modifying code becomes increasingly challenging. Readable code, characterized by meaningful names, consistent formatting, and clear logic, significantly reduces the cognitive load on developers. It ensures that even months or years after the code was written, developers can quickly grasp its purpose, behavior, and structure. This, in turn, leads to more efficient debugging, easier collaboration among team members, and smoother onboarding of new developers.
2. Introducing Named Functions
Named functions are functions in JavaScript that have a user-defined name, as opposed to anonymous functions that lack a name or are assigned to a variable. While both anonymous and named functions have their place, named functions offer distinct advantages when it comes to code readability.
2.1. Clarity in Stack Traces
When an error occurs in your code and a stack trace is generated, having named functions greatly improves the error message’s clarity. Instead of seeing something like:
javascript TypeError: Cannot read property 'x' of undefined at <anonymous>:2:19
You’ll get:
javascript TypeError: Cannot read property 'x' of undefined at calculateDistance (script.js:5:15)
In the second example, the error message includes the name of the function where the error originated (i.e., calculateDistance). This allows you to pinpoint the exact location of the error and understand the context more easily.
3. Improved Self-Documenting Code
Named functions act as a form of self-documentation. When you give a function a meaningful name that reflects its purpose, you create a clear link between the function’s name and its behavior. This reduces the need for excessive comments and helps developers understand what a function does without having to dive into its implementation details.
Consider the following example:
javascript // Without named function const getUser = async function(id) { // ... code to fetch user by id }; // With named function async function fetchUserById(id) { // ... code to fetch user by id }
In the second example, the function’s name (fetchUserById) conveys its purpose without requiring additional comments. This not only makes the code easier to understand but also encourages consistent naming conventions throughout the codebase.
4. Encapsulation and Modularity
Named functions promote encapsulation and modularity in your codebase. By giving meaningful names to functions, you encourage the creation of smaller, focused functions that handle specific tasks. This compartmentalization of functionality makes your codebase more organized and maintainable. Additionally, the descriptive function names can guide other developers to use these functions appropriately, reducing the chances of misusing or misunderstanding their behavior.
5. Best Practices for Using Named Functions
To fully reap the benefits of named functions and improve code readability, consider the following best practices:
5.1. Choose Descriptive Names
When naming your functions, opt for names that succinctly describe the purpose and functionality of the function. A well-chosen name should provide a clear understanding of what the function does without needing to read its implementation.
5.2. Follow Naming Conventions
Adhere to established naming conventions within your project or organization. Consistency in naming makes the codebase more coherent and predictable. For instance, if your project uses camelCase naming, make sure your named functions follow the same convention.
5.3. Keep Functions Small and Focused
Named functions work best when they have a clear and specific purpose. Aim to create functions that do one thing and do it well. If a function becomes too large or takes on too many responsibilities, consider refactoring it into smaller, more focused functions.
5.4. Limit the Use of Anonymous Functions
While anonymous functions have their uses, relying on them exclusively can lead to less readable code. Reserve anonymous functions for cases where they improve code structure, and prefer named functions for anything more complex.
6. Code Examples
Let’s explore a couple of code examples to highlight the practical advantages of named functions:
Example 1: Event Handling
javascript // Anonymous function element.addEventListener('click', function() { // ... code to handle the click event }); // Named function function handleClick() { // ... code to handle the click event } element.addEventListener('click', handleClick);
In this example, using a named function (handleClick) improves readability by clearly indicating the purpose of the function and its association with the event listener.
Example 2: Data Transformation
javascript // Anonymous function const modifiedData = rawData.map(function(item) { // ... code to transform the data return transformedItem; }); // Named function function transformData(item) { // ... code to transform the data return transformedItem; } const modifiedData = rawData.map(transformData);
By using a named function (transformData), the intent behind the data transformation becomes more evident, enhancing the overall readability of the code.
Conclusion
Named functions are a powerful tool in your arsenal for improving code readability in JavaScript. Their ability to provide clear stack traces, self-documenting code, and promote modularity makes them a valuable asset for any project. By following best practices and adopting named functions where appropriate, you can create a codebase that is not only easier to understand but also more maintainable and robust. Ultimately, prioritizing code readability enhances developer productivity, reduces error rates, and contributes to the long-term success of your software projects.
Table of Contents
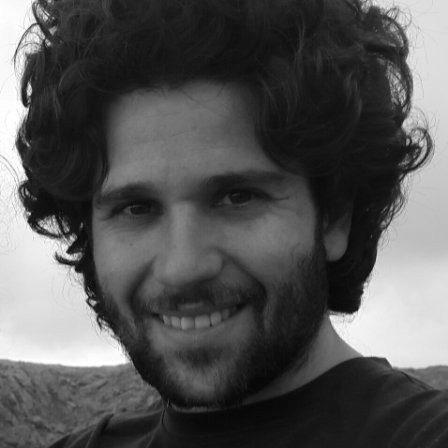
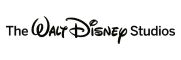