JavaScript Function Composition Patterns for Modular Code
JavaScript’s flexibility allows for a variety of programming paradigms, including functional programming. One of the most powerful techniques in functional programming is function composition. By composing functions, developers can build modular and reusable code that is both easier to test and maintain. This article explores JavaScript function composition patterns, providing practical examples of how to use them effectively.
Understanding Function Composition
Function composition involves combining multiple functions to create a new function. This new function applies each of the composed functions in a specific order, passing the result of one function as the input to the next. Function composition is useful for creating more modular code by breaking down complex logic into simpler, reusable functions.
Basic Function Composition in JavaScript
JavaScript allows function composition using simple function calls and the `compose` utility. Let’s start with a basic example.
Example: Basic Function Composition
Suppose we have two functions: `add` and `multiply`.
```javascript // Basic functions const add = x => x + 2; const multiply = x => x * 3; // Composing functions const compose = (f, g) => x => f(g(x)); // Composed function const addThenMultiply = compose(multiply, add); console.log(addThenMultiply(5)); // Output: 21 (5 + 2) * 3 ```
In this example, `addThenMultiply` first applies the `add` function and then the `multiply` function.
Advanced Function Composition with Multiple Functions
For more complex scenarios, you may need to compose multiple functions. You can extend the `compose` function to handle more than two functions.
Example: Composing Multiple Functions
```javascript const subtract = x => x - 1; const divide = x => x / 2; // Composing multiple functions const composeMultiple = (...fns) => x => fns.reduceRight((acc, fn) => fn(acc), x); const finalFunction = composeMultiple(divide, subtract, multiply, add); console.log(finalFunction(8)); // Output: 10 (8 + 2) * 3 - 1 / 2 ```
Here, `composeMultiple` combines `divide`, `subtract`, `multiply`, and `add` into a single function, applying them from right to left.
Using Function Composition with Libraries
JavaScript libraries like Lodash and Ramda provide built-in support for function composition, simplifying the process and adding useful features.
Example: Using Lodash for Function Composition
```javascript const _ = require('lodash'); const composedFunction = _.flow([ add, multiply, subtract, divide ]); console.log(composedFunction(8)); // Output: 10 (8 + 2) * 3 - 1 / 2 ```
Lodash’s `flow` function creates a composition of functions that executes them in sequence.
Creating Reusable and Modular Code
Function composition promotes code reuse and modularity. By breaking down complex functions into smaller, composable pieces, you can create more maintainable and testable code.
Example: Modular Functions for User Data
```javascript const capitalize = str => str.charAt(0).toUpperCase() + str.slice(1); const formatDate = date => new Date(date).toLocaleDateString(); const processUser = compose( user => ({ ...user, name: capitalize(user.name) }), user => ({ ...user, registrationDate: formatDate(user.registrationDate) }) ); const user = { name: 'john doe', registrationDate: '2024-08-01' }; console.log(processUser(user)); // Output: { name: 'John doe', registrationDate: '8/1/2024' } ```
In this example, `processUser` uses function composition to format user data, making the code more modular and reusable.
Conclusion
Function composition is a powerful technique in JavaScript that enhances code modularity and reusability. By composing functions, developers can create cleaner, more maintainable code and simplify complex logic. Whether using basic function composition or leveraging libraries, this approach can significantly improve your JavaScript codebase.
Further Reading:
Table of Contents
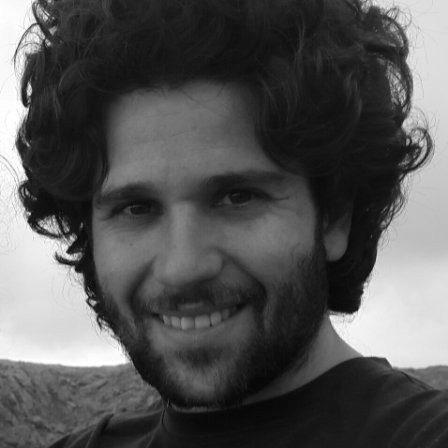
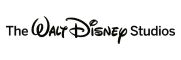