The Magic of JavaScript Event Listeners: Harnessing Functionality
In the dynamic realm of web development, creating engaging and interactive user experiences is paramount. The ability to respond to user actions such as clicks, inputs, and other events is a fundamental aspect of achieving this goal. This is where JavaScript event listeners come into play. These unassuming lines of code possess an incredible power to bring life to your web pages, making them responsive, user-friendly, and downright magical. In this guide, we’ll embark on a journey to unravel the enchanting world of JavaScript event listeners, exploring their mechanisms, applications, and the art of harnessing their full functionality.
Table of Contents
1. Understanding Events: The Building Blocks of Interactivity
Before we dive into the world of event listeners, let’s establish a solid foundation by understanding what events are. Events are occurrences that take place in the browser – they can be triggered by the user’s actions or by other parts of the application. Common examples of events include clicks, keypresses, mouse movements, form submissions, and more. These events provide a means for your website to respond to user interactions in real time.
2. The Event Object: A Window into User Actions
In JavaScript, when an event occurs, an associated event object is created. This object holds a wealth of information about the event, including details about the target element, the type of event, and any additional data relevant to the specific event. By accessing this object, you can tap into a treasure trove of information that empowers you to tailor your application’s response to the user’s action.
javascript // Example of accessing event object properties document.getElementById('myButton').addEventListener('click', function(event) { console.log('Button clicked!'); console.log('Target element:', event.target); console.log('Type of event:', event.type); });
3. Enter Event Listeners: Your Key to Interactive Sorcery
Event listeners are JavaScript constructs that allow you to “listen” for specific events on HTML elements and execute custom code in response. This is where the magic truly happens – you can create captivating interactions that respond to a user’s every move.
3.1. Adding Event Listeners: The Spellbinding Ritual
The process of adding an event listener involves selecting a target element and specifying the event you want to listen for. When the event occurs, the provided callback function is executed. This is the heart of event-driven programming.
javascript // Adding a click event listener const myButton = document.getElementById('myButton'); myButton.addEventListener('click', function() { alert('Button clicked! Enchantment in progress.'); });
3.2. Types of Event Listeners: A Spellbook of Possibilities
JavaScript offers a plethora of event types you can listen for. Some common event types include:
3.2.1. Click Events
javascript const clickableElement = document.getElementById('clickableElement'); clickableElement.addEventListener('click', function() { console.log('Element clicked!'); });
3.2.2. Input Events
javascript const inputField = document.getElementById('inputField'); inputField.addEventListener('input', function(event) { console.log('Input value changed:', event.target.value); });
3.2.3. Keydown Events
javascript document.addEventListener('keydown', function(event) { console.log('Key pressed:', event.key); });
4. Event Bubbling and Capturing: Navigating the Spellbinding Web
Understanding event propagation is vital to becoming a true sorcerer of event handling. When an event occurs on an element, it doesn’t just affect that element – it ripples through the DOM tree. This phenomenon is known as event propagation and involves two phases: capturing and bubbling.
4.1. Capturing Phase
During the capturing phase, the event starts from the root of the DOM tree and trickles down to the target element. This is less commonly used in practice but can be a powerful tool in certain scenarios.
4.2. Bubbling Phase
The bubbling phase is the default behavior. After the event is triggered on the target element, it bubbles up through its parent elements in the DOM tree. This makes it easier to capture events at a higher level in the hierarchy.
javascript // Example of stopping event propagation const parentElement = document.getElementById('parentElement'); const childElement = document.getElementById('childElement'); parentElement.addEventListener('click', function() { console.log('Parent element clicked!'); }); childElement.addEventListener('click', function(event) { console.log('Child element clicked!'); event.stopPropagation(); // Prevent the event from bubbling up });
5. Enchanting User Experiences: Practical Applications of Event Listeners
Now that we’ve grasped the essence of event listeners, let’s explore their practical applications to craft enchanting user experiences.
5.1. Form Validation: Guiding Your Users
Form validation is a classic use case for event listeners. By listening to the ‘submit’ event on a form, you can prevent it from being submitted if the provided data is invalid.
javascript const form = document.getElementById('myForm'); form.addEventListener('submit', function(event) { event.preventDefault(); // Prevent the form from submitting // Validate form fields if (/* validation fails */) { // Display error messages } else { // Submit the form } });
5.2. Dynamic Content Loading: Unveiling Hidden Truths
You can employ event listeners to load content dynamically as users interact with your page. This is often seen in scenarios where additional information or images are loaded when a user clicks on a certain element.
javascript const loadMoreButton = document.getElementById('loadMoreButton'); const contentContainer = document.getElementById('contentContainer'); loadMoreButton.addEventListener('click', function() { // Fetch and append more content to the container });
5.3. Creating Interactive Games: Spells of Entertainment
Event listeners are the core ingredients for creating interactive games that respond to player input. Whether it’s a puzzle, quiz, or a full-fledged game, event listeners enable you to capture player actions and trigger corresponding game mechanics.
6. The Art of Event Delegation: Efficient Spellcasting
As your web applications grow in complexity, managing event listeners can become a challenge. This is where the art of event delegation comes into play. Event delegation involves placing a single event listener on a common ancestor element of multiple target elements. This approach is especially useful when dealing with dynamically generated content.
javascript // Using event delegation to handle clicks on multiple elements const listContainer = document.getElementById('listContainer'); listContainer.addEventListener('click', function(event) { if (event.target.tagName === 'LI') { console.log('List item clicked:', event.target.textContent); } });
7. Unleash the Magic: Best Practices and Considerations
As you embark on your journey of mastering JavaScript event listeners, keep these best practices and considerations in mind:
- Performance: Be mindful of adding too many event listeners, as it can affect page performance. Consider using event delegation for efficiency.
- Memory Management: Remove event listeners when they are no longer needed to prevent memory leaks.
- Accessibility: Ensure that your interactive elements remain accessible to users with disabilities by providing proper keyboard and screen reader support.
- Compatibility: While modern browsers support event listeners well, test your code across different browsers to ensure consistent behavior.
Conclusion
JavaScript event listeners are like the wands of web development – they allow you to wield the power of interactivity and responsiveness. Armed with the knowledge of events, event listeners, and their applications, you are now equipped to enchant your web pages with captivating interactions. From simple clicks to complex game mechanics, the possibilities are boundless. So go forth, harness the magic of event listeners, and create web experiences that leave users spellbound.
Table of Contents
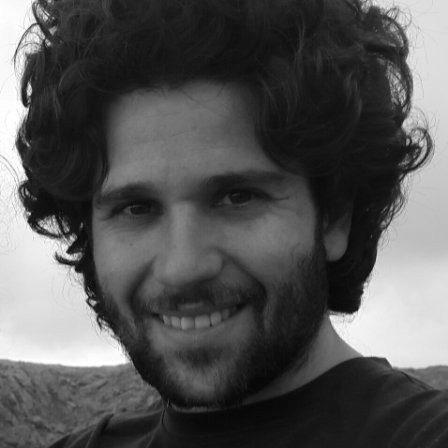
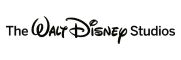