The Art of JavaScript Function Composition
JavaScript is a versatile and powerful programming language, allowing developers to build complex and interactive web applications. One of the key features that make JavaScript stand out is its ability to use functions as first-class citizens. In JavaScript, functions can be passed as arguments to other functions, returned from functions, and even assigned to variables. This powerful concept forms the foundation of “Function Composition.”
Table of Contents
What is Function Composition?
Function composition is a functional programming technique where multiple functions are combined to create a new function. The output of one function becomes the input of another, enabling developers to build a pipeline of operations, akin to assembling building blocks. Function composition promotes code modularity, reusability, and maintainability.
Advantages of Function Composition
- Code Reusability: Composing functions allows you to reuse specific blocks of functionality in multiple parts of your application.
- Readability: By breaking complex operations into smaller functions, you can enhance code readability and make it easier to understand.
- Maintainability: Separate functions are easier to maintain and test, making it simpler to identify and fix issues.
- Debugging: Debugging becomes less cumbersome as you can focus on individual functions and their outputs.
- Flexibility: Composing functions grants you the flexibility to rearrange or replace components without affecting the entire codebase.
Understanding Pure Functions
Before diving into function composition, it’s crucial to understand “Pure Functions.” A pure function is a function that always returns the same output for the same given input and has no side effects. Side effects include modifying external state, I/O operations, or anything that affects the outside world.
Consider this example of a pure function:
javascript function add(a, b) { return a + b; }
The add function takes two arguments and returns their sum. It doesn’t modify any external state and will always produce the same result for the same inputs.
Composing Functions in JavaScript
JavaScript provides several ways to compose functions. Let’s explore some popular methods for function composition.
1. Using Helper Functions
Helper functions are utility functions that allow you to combine functions in a readable manner. Let’s say we have two functions, double and addTen, which double a number and then add ten to it, respectively.
javascript function double(x) { return x * 2; } function addTen(x) { return x + 10; }
We can create a helper function, compose, to combine these functions:
javascript function compose(f, g) { return function (x) { return f(g(x)); }; } const doubleThenAddTen = compose(addTen, double); console.log(doubleThenAddTen(5)); // Output: 20 (double(5) => 10, addTen(10) => 20)
The compose function takes two functions, f and g, and returns a new function that first applies g to the input, then applies f to the result.
2. Using the pipe Function
Similar to compose, the pipe function allows you to combine functions, but in a different order. With pipe, the data flows left to right, applying functions in sequence.
javascript function pipe(f, g) { return function (x) { return g(f(x)); }; } const addTenThenDouble = pipe(addTen, double); console.log(addTenThenDouble(5)); // Output: 30 (addTen(5) => 15, double(15) => 30)
3. Using Ramda Library
The Ramda library is a functional programming library that provides a rich set of tools for working with data and functions. It offers a handy compose function, making function composition even more straightforward.
First, install Ramda using npm or yarn:
bash npm install ramda
Now, you can use the R.compose function:
javascript const R = require('ramda'); const addTenThenDouble = R.compose(double, addTen); console.log(addTenThenDouble(5)); // Output: 30 (addTen(5) => 15, double(15) => 30)
Real-World Example
Let’s explore a real-world example of function composition. Consider a web application that needs to filter a list of products based on various criteria: price range, category, and availability. Instead of writing a monolithic filtering function, we can create smaller, reusable functions and then compose them to get the final filtered result.
javascript // Sample list of products const products = [ { name: 'Product A', price: 20, category: 'Electronics', inStock: true }, { name: 'Product B', price: 50, category: 'Clothing', inStock: false }, { name: 'Product C', price: 30, category: 'Electronics', inStock: true }, // Add more products here... ]; // Helper functions function filterByPrice(min, max) { return function (product) { return product.price >= min && product.price <= max; }; } function filterByCategory(category) { return function (product) { return product.category === category; }; } function filterByAvailability(inStock) { return function (product) { return product.inStock === inStock; }; } // Combining the filters const filteredProducts = products.filter( R.allPass([ filterByPrice(20, 40), filterByCategory('Electronics'), filterByAvailability(true), ]) ); console.log(filteredProducts); // Output: [{ name: 'Product A', price: 20, category: 'Electronics', inStock: true }]
In this example, we defined three filter functions and used Ramda’s R.allPass to compose them into a single filter function. The resulting filteredProducts array contains products that satisfy all three filter conditions.
Conclusion
Function composition is a powerful technique in JavaScript that allows developers to build modular and reusable code. By breaking complex operations into smaller, pure functions and combining them, we create a more manageable and elegant codebase. Whether using simple helper functions or leveraging libraries like Ramda, mastering the art of function composition will undoubtedly enhance your JavaScript programming skills. Embrace the functional programming paradigm and unlock endless possibilities in your coding journey!
Table of Contents
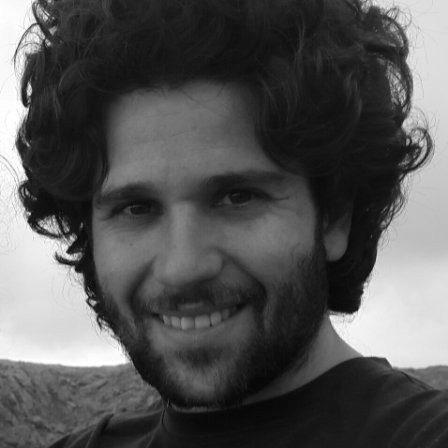
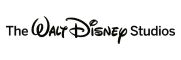