Exploring JavaScript Function Overloading Techniques
JavaScript is a versatile and dynamic programming language, and one of its essential features is the ability to create flexible and powerful functions. Function overloading is a technique that allows us to define multiple versions of a function with different parameters or behavior, enabling us to handle various use cases gracefully. In this blog, we will dive deep into the world of JavaScript function overloading and explore different techniques to harness its potential. From traditional approaches to modern ones, we will provide code samples and examples to help you grasp the concept and apply it effectively in your projects.
Table of Contents
Understanding Function Overloading
Function overloading is the practice of defining multiple functions with the same name but different parameters or argument types. When a function is called, JavaScript determines which version of the function to execute based on the number and types of arguments passed to it. This enables us to create functions that adapt to different scenarios without cluttering our code with conditional statements.
Traditional Approach: Using Conditional Statements
The traditional way to achieve function overloading in JavaScript involves using conditional statements to check the number of arguments and their types. Let’s consider an example where we want to create a function to calculate the area of different shapes: circle, rectangle, and triangle.
javascript function calculateArea(shape, ...args) { if (shape === 'circle' && args.length === 1) { const [radius] = args; return Math.PI * radius * radius; } else if (shape === 'rectangle' && args.length === 2) { const [length, width] = args; return length * width; } else if (shape === 'triangle' && args.length === 2) { const [base, height] = args; return (base * height) / 2; } else { throw new Error('Invalid shape or arguments.'); } }
While this approach works, it becomes cumbersome and less maintainable as the number of shapes and their corresponding arguments increases. Let’s explore more elegant and dynamic ways to achieve function overloading.
Approach 1: Argument-based Overloading
In this approach, we utilize the concept of function overloading by dynamically handling different argument combinations. We will use an object to map the expected argument patterns to their corresponding implementation functions.
javascript function calculateArea(...args) { const shapeHandlers = { circle: (radius) => Math.PI * radius * radius, rectangle: (length, width) => length * width, triangle: (base, height) => (base * height) / 2, }; const [shape, ...rest] = args; if (shape in shapeHandlers && shapeHandlers[shape].length === rest.length) { return shapeHandlers[shape](...rest); } else { throw new Error('Invalid shape or arguments.'); } }
In this approach, we extract the shape argument from the rest of the arguments. Then, we check if the shape is a valid key in our shapeHandlers object and whether the corresponding function has the same number of arguments as the ones provided. If the conditions are met, we call the appropriate function with the extracted arguments. This way, we can easily add or modify new shapes and their respective calculations without modifying the main function.
Approach 2: Type-based Overloading with Function Properties
Another way to implement function overloading in JavaScript is by associating functions with specific argument types using function properties. We will define separate functions for each argument type, and then use the function properties to select the appropriate implementation.
javascript function calculateArea(circle) { return Math.PI * circle.radius * circle.radius; } function calculateArea(rectangle) { return rectangle.length * rectangle.width; } function calculateArea(triangle) { return (triangle.base * triangle.height) / 2; }
In this approach, we create separate functions with the same name but different parameter names, each representing a specific shape and its arguments. When calling the calculateArea function, JavaScript will choose the correct implementation based on the argument’s structure.
Approach 3: Using the arguments object
The arguments object is a built-in feature in JavaScript that provides a way to access all the arguments passed to a function, regardless of the number of declared parameters. We can use this object to manually handle different argument combinations.
javascript function calculateArea() { const shape = arguments[0]; if (shape === 'circle' && arguments.length === 2) { const radius = arguments[1]; return Math.PI * radius * radius; } else if (shape === 'rectangle' && arguments.length === 3) { const length = arguments[1]; const width = arguments[2]; return length * width; } else if (shape === 'triangle' && arguments.length === 3) { const base = arguments[1]; const height = arguments[2]; return (base * height) / 2; } else { throw new Error('Invalid shape or arguments.'); } }
In this approach, we retrieve the first argument (representing the shape) using arguments[0] and then check the number of arguments to determine the appropriate calculation for each shape. While this method is dynamic, it lacks clarity and can lead to confusion, especially when handling a large number of arguments.
Approach 4: ES6 Destructuring and Default Parameters
With the introduction of ES6, we have access to features like destructuring and default parameters, which offer more concise ways to implement function overloading.
javascript function calculateArea({ shape, radius, length, width, base, height }) { switch (shape) { case 'circle': return Math.PI * radius * radius; case 'rectangle': return length * width; case 'triangle': return (base * height) / 2; default: throw new Error('Invalid shape or arguments.'); } }
In this approach, we use destructuring to extract the required arguments from a single object parameter. Depending on the shape provided, the corresponding calculation will be performed. This approach enhances readability and maintainability, making it easier to understand the function’s behavior.
Conclusion
Function overloading is a powerful technique in JavaScript that allows us to create versatile and adaptive functions. By exploring various approaches, we can improve code clarity, maintainability, and flexibility. Whether you choose the traditional conditional statements, argument-based overloading, type-based overloading with function properties, using the arguments object, or the modern ES6 destructuring, understanding function overloading opens up new possibilities for building robust and extensible applications. Choose the technique that best suits your project’s needs and leverage the true potential of JavaScript function overloading. Happy coding!
Table of Contents
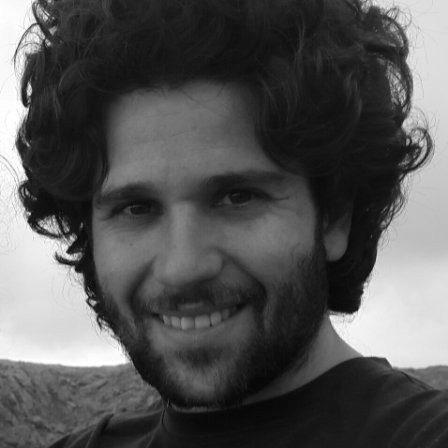
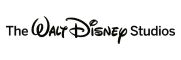