Understanding JavaScript Pure Functions in Functional Programming
In the realm of functional programming, pure functions stand as one of its foundational principles. These functions hold a pivotal role in ensuring predictable, maintainable, and scalable codebases. Whether you’re a seasoned JavaScript developer or just starting your programming journey, comprehending pure functions is essential for writing efficient and robust code.
Table of Contents
1. Introduction to Pure Functions
In the realm of functional programming, the term “pure function” refers to a specific type of function that produces consistent and deterministic results based solely on its inputs. This means that given the same set of input values, a pure function will always return the same output, without causing any side effects. Side effects include modifying external state, such as changing variables or interacting with I/O operations.
1.1. Characteristics of Pure Functions
- Pure functions exhibit a set of defining characteristics that distinguish them from other types of functions:
- Deterministic: As mentioned earlier, pure functions are deterministic in nature. This determinism ensures that the same inputs will always yield the same outputs, making them predictable and reliable.
- No Side Effects: Pure functions do not cause side effects, which means they don’t alter variables outside their scope or modify external state. This property contributes to code predictability and makes debugging and testing easier.
- Referential Transparency: Referential transparency implies that you can replace a function call with its corresponding output value without affecting the program’s behavior. This property simplifies reasoning about the code.
Function Purity: The purity of a function is a binary property – it’s either pure or not. There’s no middle ground. If a function has even a single line of code that introduces a side effect, it loses its purity.
2. Benefits of Using Pure Functions
2.1. Predictability and Testability
Predictable code is a developer’s dream. When your code relies heavily on pure functions, you can be confident that a specific input will always yield a specific output. This predictability simplifies debugging and testing, as you don’t need to consider unexpected side effects or hidden dependencies.
Unit testing becomes more straightforward with pure functions. Since they don’t modify external state, you can test them in isolation, providing different inputs and verifying the corresponding outputs. This isolated testing approach leads to more robust and maintainable test suites.
2.2. Reusability
Pure functions encourage reusability. Because they don’t rely on external state or mutable data, you can use them across different parts of your application without worrying about unintended consequences. This promotes modularity and helps you build more modular and composable code.
2.3. Parallel Execution
The lack of side effects in pure functions enables parallel execution. Since pure functions don’t interact with shared state, they can be executed simultaneously without causing race conditions or unexpected behavior. This property becomes crucial in today’s multi-core and distributed computing environments.
3. Code Examples
3.1. Basic Pure Function
Let’s start with a simple example of a pure function in JavaScript:
javascript function square(x) { return x * x; }
In this example, the square function takes an input x and returns the square of that value. It adheres to the characteristics of pure functions: it always produces the same output for the same input, and it doesn’t have any side effects.
3.2. Impure vs. Pure Functions
To further understand pure functions, let’s contrast them with impure functions:
javascript // Impure Function let multiplier = 2; function multiply(x) { return x * multiplier; } // Pure Function function pureMultiply(x, factor) { return x * factor; }
In the multiply function, the result depends on the external variable multiplier, which introduces an element of uncertainty. On the other hand, pureMultiply is a pure function since it solely depends on its inputs and doesn’t access any external state.
3.3. Functional Composition
One of the powerful aspects of pure functions is their compatibility with functional composition. Functional composition involves chaining pure functions together to create more complex functions. This approach promotes a declarative and modular coding style.
javascript function addTwo(x) { return x + 2; } function multiplyByThree(x) { return x * 3; } // Composing pure functions const composedFunction = (x) => multiplyByThree(addTwo(x)); console.log(composedFunction(5)); // Output: 21
In this example, addTwo and multiplyByThree are pure functions that each perform a specific transformation. By composing these functions, you can create a new function that combines their effects.
4. Guidelines for Writing Pure Functions
While using pure functions offers numerous benefits, writing them effectively requires adhering to certain guidelines:
4.1. Avoiding Side Effects
To maintain the purity of a function, ensure that it doesn’t modify external variables, interact with I/O operations, or make API calls. Any form of external interaction breaks the determinism and predictability of pure functions.
4.2. Immutable Data
Pure functions work best with immutable data. Immutable data cannot be changed after creation, which aligns with the principle of avoiding side effects. If your function requires modification of data, consider creating a new copy with the necessary changes instead.
4.3. Deterministic
The deterministic nature of pure functions is their cornerstone. Avoid using random number generators or other sources of non-determinism within pure functions. This ensures that the same inputs consistently yield the same outputs.
Conclusion
Understanding pure functions is pivotal in mastering functional programming with JavaScript. Their predictability, reusability, and compatibility with parallel execution make them essential building blocks of maintainable and efficient codebases. By adhering to the principles of pure functions, you pave the way for more reliable software that’s easier to debug, test, and scale. So, whether you’re just starting or are already knee-deep in functional programming, make pure functions your allies in the journey to writing cleaner and more robust JavaScript code.
Table of Contents
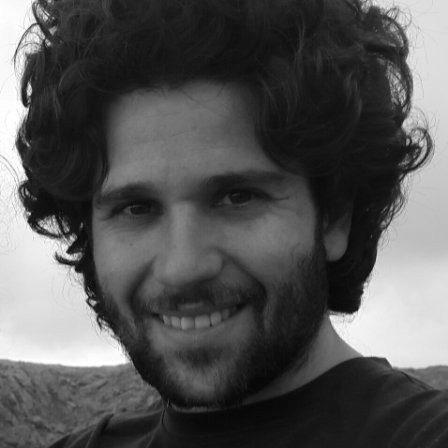
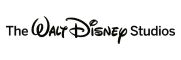