JavaScript Recursive Functions: Unleashing Their Power
JavaScript is a versatile and powerful programming language, known for its ability to create interactive and dynamic web applications. One of its essential features is the capability to use recursive functions, allowing developers to solve complex problems and implement efficient algorithms. In this blog, we will explore the concept of recursive functions, how they work, and various scenarios where they can be leveraged to simplify code and improve performance.
Table of Contents
Understanding Recursive Functions
A recursive function is a programming technique where a function calls itself within its body to solve a smaller version of the same problem. This process continues until a base case is reached, upon which the function starts to unwind the calls and return results back through the chain of function calls.
The structure of a recursive function typically consists of two parts:
- Base Case: The condition that determines when the recursion should stop and the function should start returning results.
- Recursive Case: The part of the function where it calls itself with a reduced version of the problem to be solved.
Recursion can be a challenging concept to grasp initially, but with a clear understanding and careful implementation, it becomes a powerful tool for solving various programming challenges.
The Beauty of Recursion: Simplifying Problems
Recursive functions can significantly simplify the implementation of certain algorithms by breaking down complex problems into smaller, more manageable sub-problems. This approach is particularly useful when dealing with tasks that exhibit repetitive patterns.
Example: Calculating Factorials
Let’s explore one of the classic examples of recursion, the calculation of factorials. The factorial of a non-negative integer n is the product of all positive integers less than or equal to n. Mathematically, it is denoted as n!.
javascript function factorial(n) { // Base case: factorial of 0 is 1 if (n === 0) { return 1; } // Recursive case: calculate factorial(n-1) and multiply it by n return n * factorial(n - 1); } // Test the function console.log(factorial(5)); // Output: 120
In this example, the recursive function factorial calculates the factorial of a given number n. The base case is defined when n is 0, where the function immediately returns 1. For any other positive n, the function recursively calls itself with n-1, gradually reducing the problem size until the base case is reached.
Example: Fibonacci Sequence
The Fibonacci sequence is another classic problem that can be elegantly solved using recursion. The sequence starts with 0 and 1, and each subsequent number is the sum of the two preceding ones.
javascript function fibonacci(n) { // Base cases: fibonacci(0) is 0, fibonacci(1) is 1 if (n === 0) { return 0; } if (n === 1) { return 1; } // Recursive case: calculate fibonacci(n-1) and fibonacci(n-2), then add them together return fibonacci(n - 1) + fibonacci(n - 2); } // Test the function console.log(fibonacci(7)); // Output: 13
Similar to the factorial example, the Fibonacci function uses recursion to compute the nth number in the sequence. The base cases are defined for n equal to 0 and 1, returning 0 and 1, respectively. For any other value of n, the function recursively calls itself twice with n-1 and n-2 and returns the sum of the two results.
Managing Function Call Stack
One crucial aspect to consider when working with recursive functions is the function call stack. Each function call consumes memory on the call stack, and an excessive number of recursive calls without proper termination conditions can lead to a stack overflow error.
To avoid this issue, it is essential to define the base case correctly, ensuring that the recursion stops when the problem size is reduced to its simplest form. Additionally, tail recursion optimization, which some programming languages offer, can also be utilized to optimize recursive functions and avoid stack overflows.
Recursion vs. Iteration: When to Use Which?
Recursion and iteration are two common approaches to solving programming problems, and while they can achieve similar results, each has its strengths and weaknesses.
Advantages of Recursion
- Elegant Code: Recursive solutions often result in more concise and readable code, especially for problems with inherent recursive nature.
- Divide and Conquer: Recursion naturally breaks down complex problems into smaller, more manageable sub-problems, making the code easier to understand and maintain.
- Tree-like Structures: Problems involving tree-like structures, such as tree traversal or graph algorithms, can be elegantly handled using recursion.
Advantages of Iteration
- Efficiency: In some cases, iteration can be more efficient than recursion, as it avoids the overhead of function calls.
- Space Complexity: Recursive solutions can consume more memory due to the function call stack, while iteration generally requires less memory.
- Tail Recursion Limitation: Not all programming languages and environments support tail recursion optimization, which can lead to stack overflow errors for large inputs.
It’s important to assess the specific requirements of a problem and consider the limitations of both approaches before choosing between recursion and iteration.
Practical Use Cases for Recursive Functions
Let’s explore some practical use cases where recursive functions can shine and offer elegant solutions.
Directory Traversal
Consider a scenario where you need to traverse a complex directory structure and perform a specific operation on each file. A recursive function can efficiently handle this task, as it can recursively explore each subdirectory and its files, eliminating the need for complex nested loops.
javascript function processFilesInDirectory(directory) { const files = getFilesInDirectory(directory); for (const file of files) { if (isFile(file)) { // Process the file // ... } else if (isDirectory(file)) { // Recursively process subdirectory processFilesInDirectory(file); } } }
The processFilesInDirectory function utilizes recursion to explore each directory within the given directory, processing files along the way.
Tree Traversal
Tree data structures are prevalent in computer science, and their traversal is often required to perform various operations. Whether it’s a binary search tree or a DOM tree, recursive functions can elegantly traverse the tree’s nodes.
javascript class TreeNode { constructor(value) { this.value = value; this.left = null; this.right = null; } } function preOrderTraversal(node) { if (node === null) { return; } // Process the current node console.log(node.value); // Recursively traverse left subtree preOrderTraversal(node.left); // Recursively traverse right subtree preOrderTraversal(node.right); }
In this example, the preOrderTraversal function performs a pre-order traversal on a binary tree. It visits the root node, then recursively traverses the left subtree before moving to the right subtree.
Conclusion
JavaScript recursive functions are a powerful tool in a developer’s toolkit. They provide an elegant way to solve complex problems by breaking them down into simpler sub-problems. However, with great power comes great responsibility. Care must be taken to define the base case correctly and consider potential stack overflow errors.
By understanding the concept of recursion, its advantages, and practical applications, you can leverage recursive functions to write cleaner, more efficient code and tackle a wide range of programming challenges. So go ahead, unleash the power of recursion, and take your JavaScript coding skills to new heights!
Table of Contents
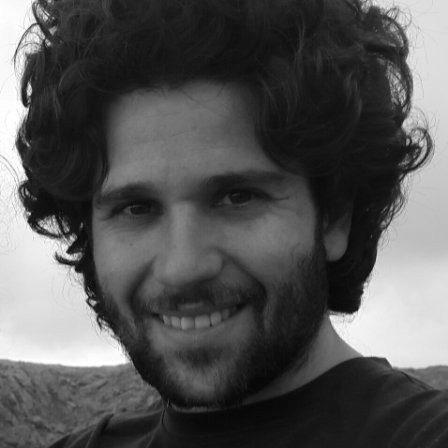
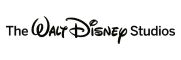