Implementing JavaScript Timing Functions: setTimeout and setInterval
When building modern web applications, developers often need to introduce time-based behaviors, such as delaying an action, running code at regular intervals, or animating elements on a webpage. JavaScript provides two essential timing functions, setTimeout and setInterval, which allow us to schedule tasks and manage time-based events effectively.
Table of Contents
In this blog, we will dive into the world of JavaScript timing functions, exploring their differences, use cases, and best practices. Whether you are a seasoned developer or a newcomer to JavaScript, understanding these timing functions will help you build more sophisticated and interactive web applications.
1. Understanding setTimeout:
The setTimeout function allows us to execute a piece of code once after a specified delay. It is commonly used when you want to trigger an action after a certain time has passed, like showing a pop-up message or updating data periodically.
The Syntax of setTimeout:
javascript const timerId = setTimeout(callback, delay, ...args);
- callback: The function to be executed after the delay.
- delay: The time delay in milliseconds before the callback is invoked.
- …args (optional): Additional arguments to pass to the callback.
Executing Code After a Delay:
Let’s see a simple example of using setTimeout to log a message after 2 seconds:
javascript console.log("Start"); setTimeout(() => { console.log("Delayed log after 2 seconds"); }, 2000); console.log("End");
Output:
mathematica Start End Delayed log after 2 seconds
Canceling a setTimeout:
If you need to cancel a scheduled setTimeout before it triggers, you can use the clearTimeout function and provide the timer ID as its argument. This can be useful when dealing with conditional behaviors or user interactions.
javascript const timerId = setTimeout(() => { console.log("This won't be executed"); }, 5000); clearTimeout(timerId);
Practical Example: Creating a Simple Notification System:
Imagine you want to display a notification message on your website and hide it after a few seconds. Let’s implement a simple notification system using setTimeout:
html <!DOCTYPE html> <html> <head> <title>Simple Notification System</title> </head> <body> <div id="notification" style="display: none;">This is a notification!</div> <script> function showNotification(message) { const notification = document.getElementById("notification"); notification.textContent = message; notification.style.display = "block"; setTimeout(() => { notification.style.display = "none"; }, 3000); } showNotification("Hello, this is a test notification!"); </script> </body> </html>
2. Leveraging setInterval:
Unlike setTimeout, which triggers a function once, setInterval repeatedly executes a function at defined intervals until it is stopped. This makes it perfect for tasks that require periodic updates, such as real-time data fetching or building clocks and timers.
The Syntax of setInterval:
javascript const intervalId = setInterval(callback, interval, ...args);
- callback: The function to be executed at every interval.
- interval: The time delay in milliseconds between each execution.
- …args (optional): Additional arguments to pass to the callback.
Repeated Execution of Code:
Here’s a simple example of using setInterval to log a message every 2 seconds:
javascript const intervalId = setInterval(() => { console.log("This message will be logged every 2 seconds"); }, 2000);
Stopping an Ongoing setInterval:
To stop the continuous execution of setInterval, you can use the clearInterval function, passing the interval ID as its argument.
javascript const intervalId = setInterval(() => { console.log("This will run forever until clearInterval is called"); }, 1000); // Stop the interval after 5 seconds setTimeout(() => { clearInterval(intervalId); console.log("Interval stopped"); }, 5000);
Practical Example: Building a Countdown Timer:
Let’s create a simple countdown timer using setInterval:
html <!DOCTYPE html> <html> <head> <title>Countdown Timer</title> </head> <body> <div id="countdown"></div> <script> let remainingSeconds = 10; const countdownElement = document.getElementById("countdown"); function updateCountdown() { if (remainingSeconds >= 0) { countdownElement.textContent = `Time remaining: ${remainingSeconds} seconds`; remainingSeconds--; } else { clearInterval(countdownInterval); countdownElement.textContent = "Time's up!"; } } const countdownInterval = setInterval(updateCountdown, 1000); </script> </body> </html>
3. Choosing Between setTimeout and setInterval:
Both setTimeout and setInterval have distinct use cases and best practices. Understanding the differences between the two will help you use them effectively in your projects.
Use Cases for setTimeout:
- Displaying notifications or messages with a delay.
- Implementing delayed actions after user interactions, like debouncing or throttling input.
- Triggering animations or transitions after a certain period.
Use Cases for setInterval:
- Updating data in real-time, like stock market prices or social media feeds.
- Creating countdown timers or clocks that require continuous updates.
- Implementing animations and visual effects that need to repeat in a loop.
Best Practices for Using Timing Functions:
- Avoid using setInterval for short intervals as it might lead to performance issues or excessive resource consumption. Use requestAnimationFrame for smoother animations.
- Always clear your timers (using clearTimeout and clearInterval) when they are no longer needed to prevent memory leaks and unexpected behavior.
- When working with setTimeout, consider using Promises or async/await for cleaner and more maintainable asynchronous code.
4. Combining setTimeout and setInterval:
In some cases, you might need to combine both timing functions to achieve complex timing patterns or create cascading and nested timers.
Cascading and Nested Timers:
javascript const outerTimeout = setTimeout(() => { console.log("Outer timeout executed"); const innerInterval = setInterval(() => { console.log("Inner interval executed every 1 second"); }, 1000); setTimeout(() => { clearInterval(innerInterval); console.log("Inner interval stopped after 5 seconds"); }, 5000); }, 3000);
Practical Example: Creating an Animated Slideshow:
Let’s build an animated slideshow that displays images one by one using a combination of setTimeout and setInterval:
html <!DOCTYPE html> <html> <head> <title>Animated Slideshow</title> </head> <body> <div id="slideshow"> <img src="image1.jpg" /> </div> <script> const images = ["image1.jpg", "image2.jpg", "image3.jpg"]; const slideshowElement = document.getElementById("slideshow"); let currentImageIndex = 0; function changeImage() { slideshowElement.innerHTML = `<img src="${images[currentImageIndex]}" />`; currentImageIndex = (currentImageIndex + 1) % images.length; } function startSlideshow() { changeImage(); // Display the first image immediately // Change image every 3 seconds const slideshowInterval = setInterval(changeImage, 3000); // Stop the slideshow after 15 seconds setTimeout(() => { clearInterval(slideshowInterval); }, 15000); } startSlideshow(); </script> </body> </html>
5. Managing Timer Precision and Performance:
When dealing with JavaScript timing functions, it’s essential to be aware of timer precision and potential performance impacts.
Dealing with Timer Drift:
Timers in JavaScript are not guaranteed to be perfectly accurate, and they can suffer from timer drift, where the actual execution time deviates from the intended time. To mitigate timer drift, prefer using requestAnimationFrame for smoother animations.
Minimizing Performance Impact:
Frequent use of setInterval with short intervals can negatively affect the performance of your web application, as it may lead to increased CPU usage. Opt for larger intervals or use requestAnimationFrame when dealing with animations.
Utilizing Modern Approaches: requestAnimationFrame:
For animations and smooth visual effects, it’s recommended to use requestAnimationFrame. Unlike setTimeout and setInterval, requestAnimationFrame synchronizes with the browser’s rendering, reducing stuttering and providing a more efficient and smoother experience.
Conclusion
In this blog, we’ve explored two crucial JavaScript timing functions, setTimeout and setInterval, and how they can be used to control the execution of code and create dynamic, interactive web applications. By understanding the differences between these timing functions and their best practices, you can build robust, time-based behaviors in your projects. Remember to manage timers carefully, clear them when they’re no longer needed, and consider modern approaches like requestAnimationFrame for optimal performance and user experience. Whether you’re building simple notifications, animated slideshows, or real-time data updates, mastering these timing functions will undoubtedly level up your JavaScript programming skills. Happy coding!
Table of Contents
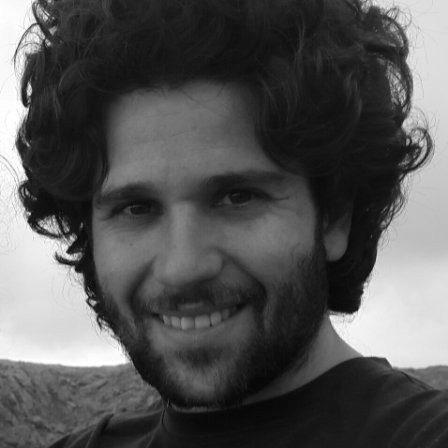
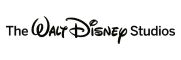