From Android to Chatbots: Kotlin’s Seamless Transition to Conversational UI
In the age of digitization, chatbots have become an essential part of many businesses and applications. Whether you’re booking a ticket, getting product support, or even setting up reminders, a chatbot can often provide a quick and efficient solution. Kotlin, a modern language running on the Java Virtual Machine (JVM), has been a game-changer in developing Android apps. Its simplicity and efficiency can also be extended to build chatbots. In this post, we’ll delve into how to make chatbots using Kotlin, simplifying the conversational UI for both developers and users.
1. Why Kotlin?
Kotlin’s distinct features make it stand out:
- Null Safety: Say goodbye to the dreaded NullPointerException.
- Concise: Write less code for the same functionality.
- Interoperable: Easily use Java libraries and frameworks.
- Functional Programming: Provides a plethora of functional programming tools.
2. Building a Basic Chatbot in Kotlin
To start off, let’s build a simple chatbot that can perform basic arithmetic operations.
```kotlin fun main() { val chatbot = Chatbot() println("Hello! I'm CalcBot. Ask me basic math questions!") while (true) { print("You: ") val input = readLine() ?: continue if (input.toLowerCase() == "exit") break println("CalcBot: ${chatbot.getResponse(input)}") } } class Chatbot { fun getResponse(input: String): String { return when { input.contains("+") -> { val numbers = input.split("+").map { it.trim().toDouble() } "${numbers.sum()}" } input.contains("-") -> { val numbers = input.split("-").map { it.trim().toDouble() } "${numbers[0] - numbers[1]}" } input.contains("*") -> { val numbers = input.split("*").map { it.trim().toDouble() } "${numbers[0] * numbers[1]}" } input.contains("/") -> { val numbers = input.split("/").map { it.trim().toDouble() } "${numbers[0] / numbers[1]}" } else -> "I'm not sure how to compute that." } } } ```
This simple chatbot accepts arithmetic expressions with basic operations and provides a result.
3. Integrating with Conversational UI Frameworks
Kotlin’s flexibility allows integration with various frameworks, one of them being Botpress, a popular chatbot framework. Here’s how you can integrate a Kotlin-built bot logic with Botpress:
- Create a RESTful API using Ktor: First, expose your chatbot logic as an API endpoint.
```kotlin import io.ktor.application.* import io.ktor.response.* import io.ktor.routing.* import io.ktor.server.engine.embeddedServer import io.ktor.server.netty.Netty fun main() { embeddedServer(Netty, port = 8080) { routing { post("/ask") { val input = call.receiveText() val chatbot = Chatbot() call.respond(chatbot.getResponse(input)) } } }.start(wait = true) } ```
- Connect Botpress to your Kotlin API: Within Botpress, set up an HTTP action to send user messages to your API and get responses.
4. Making Your Bot Smarter with NLP
Natural Language Processing (NLP) can improve chatbot comprehension. Libraries like OpenNLP or platforms like Dialogflow can be integrated with Kotlin to achieve this. Instead of using hardcoded conditions, the bot can understand intents and entities in user queries.
Final Thoughts
Kotlin isn’t only for Android app development. Its simplicity and expressive syntax can be utilized to create powerful chatbots. While the examples here were basic, they can be extended with more advanced features, machine learning, and integrations to suit any business need.
Building chatbots with Kotlin offers the potential of harnessing the power of modern programming paradigms, robust frameworks, and the extensive JVM ecosystem. The conversational UI has never been simpler. So, dive into Kotlin and start building!
Table of Contents
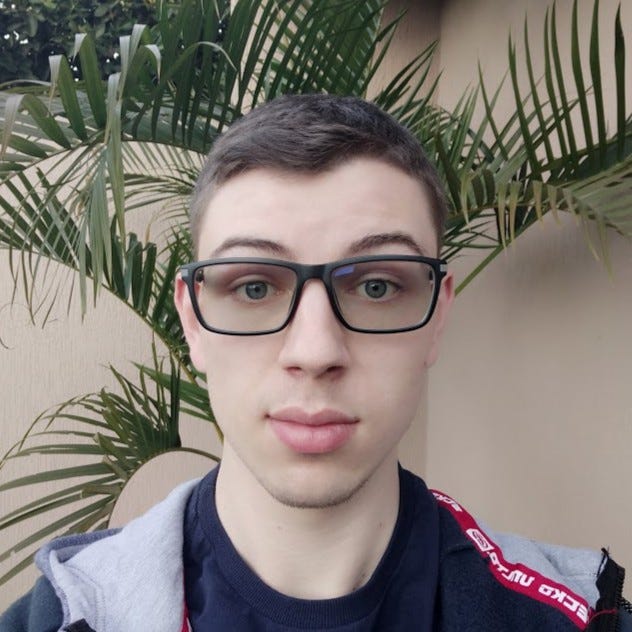
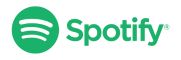