Demystifying Asynchronous Programming: A Deep Dive into Kotlin Coroutines
The challenges of concurrency in programming have long been a formidable hurdle for developers, often leading to complex and hard-to-debug code. Kotlin, a statically typed, open-source programming language developed by JetBrains, introduced a powerful tool to make concurrent programming simpler and cleaner – Kotlin Coroutines.
Kotlin Coroutines are transforming the way of writing asynchronous, non-blocking code. They simplify the management of multiple threads, ensuring smooth program execution without UI interruptions or wasteful CPU resource usage. This is one of the reasons why many businesses choose to hire Kotlin developers. In this post, we’ll delve into the fundamental concepts and advantages of Kotlin Coroutines, accompanied by practical examples. This understanding could help if you’re looking to hire Kotlin developers, as it highlights the efficiencies and improved productivity they can bring to your project.
Understanding Kotlin Coroutines
Before diving into Coroutines, it’s important to understand the concept of ‘blocking’ in programming. When a function is executing a long-running task like a network call or a complex calculation, it ‘blocks’ the thread until the task is complete. This can lead to performance issues, particularly in the context of UI-based applications where a blocked thread could result in a frozen or unresponsive user interface.
Coroutines address these issues by allowing long-running tasks to be performed without blocking the main thread, freeing it up to keep the application responsive.
Here’s an example. Consider this traditional code for making a network call:
```kotlin fun getData(): Data { // Simulate a long-running network call Thread.sleep(2000) return Data() } ``` Using coroutines, we can write this instead: ```kotlin suspend fun getData(): Data { delay(2000) return Data() } ```
By prefixing the function declaration with `suspend`, we’ve transformed it into a suspending function. These are at the heart of coroutines and allow a long-running task to suspend its execution without blocking the thread it’s running on.
Launching Coroutines
Coroutines can be launched within a specific context. The `GlobalScope` provides a general context that is not confined to any lifecycle. The `launch` function starts a new coroutine:
```kotlin GlobalScope.launch { val data = getData() // suspending function println(data) } ```
The code within the `launch` block is executed in a separate coroutine, and `getData()` is now non-blocking.
Coroutine Builders: launch and async
Coroutine builders are functions that are used to start new coroutines. The most commonly used are `launch` and `async`.
As we have seen earlier, `launch` is used to fire and forget coroutines, meaning it does not have any result value. It just executes the code within the block.
On the other hand, `async` is used when we need a result back from the coroutine. It returns an instance of `Deferred<T>`, which is a promise of a future value:
```kotlin GlobalScope.launch { val dataDeferred: Deferred<Data> = async { getData() } val data: Data = dataDeferred.await() // suspends until result is available println(data) } ```
The `await()` function is used to get the result of the `Deferred`.
Structured Concurrency
Kotlin advocates for structured concurrency, which means that coroutines operate within defined scopes, rather than being launched globally. This approach links the lifecycle of the coroutine to the lifecycle of the application, thereby ensuring more efficient resource management and stability. These technical advantages are part of the reason why many organizations choose to hire Kotlin developers. Their expertise in handling structured concurrency can lead to the development of highly optimized, efficient applications, further underscoring the value of the decision to hire Kotlin developers.
The most common structured scopes apart from `GlobalScope` are the Coroutine scope provided by the `viewModelScope` in Android and `lifecycleScope` for lifecycle-aware components. Here is an example:
```kotlin viewModelScope.launch { val data = getData() updateUI(data) } ```
In this case, if the ViewModel is cleared (for instance, if the user navigates away from the screen), all
ongoing coroutines within `viewModelScope` are automatically canceled, preventing potential memory leaks or crashes.
Error Handling
In the event of an exception within a coroutine, it can be caught in a `try/catch` block:
```kotlin try { GlobalScope.launch { val data = getData() println(data) } } catch (e: Exception) { println("Caught exception: ${e.message}") } ```
This code catches any exceptions that occur within the launched coroutine.
Concluding Thoughts
Kotlin Coroutines offer a clean and efficient way to manage asynchronous tasks in your application, with less boilerplate and more readable code. They have rich API support and integrate smoothly with other asynchronous APIs. By making use of structured concurrency, they ensure resources are used efficiently and potential memory leaks are avoided.
It’s crucial to note that, while coroutines aren’t a one-size-fits-all solution to concurrency issues, they can considerably streamline the asynchronous programming model when leveraged appropriately. Understanding the basics and using them wisely can enhance the efficiency and joy of writing your Kotlin code. This, in fact, is a core reason to hire Kotlin developers for your team.
Whether you’re crafting a mobile app, a web application, or a back-end service, coroutines can serve as a potent tool in your Kotlin toolbox for robust asynchronous programming. By opting to hire Kotlin developers, you tap into these benefits, enhancing your project’s productivity and efficiency.
Table of Contents
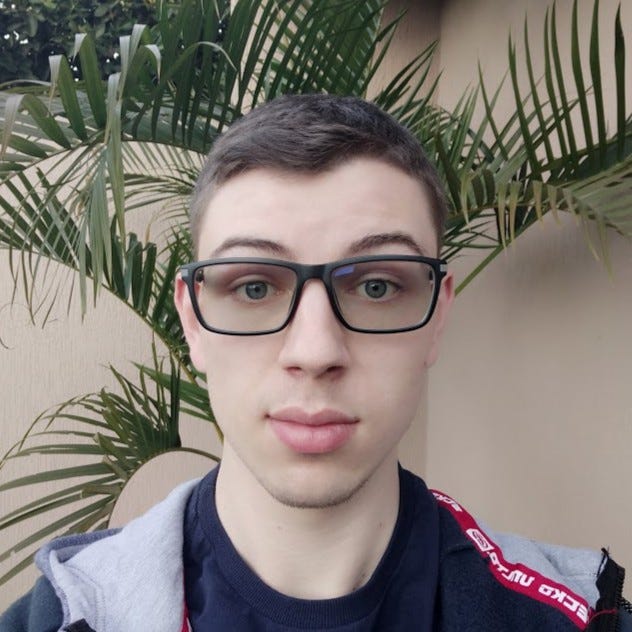
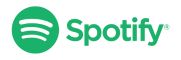