How do you define an interface in Kotlin?
In Kotlin, you define an interface using the interface keyword followed by the interface name and its member declarations. Here’s a simple example of an interface in Kotlin:
kotlin interface Shape { fun area(): Double fun perimeter(): Double }
In this example, the Shape interface declares two abstract methods: area() and perimeter(). Classes that implement the Shape interface must provide concrete implementations for these methods.
To implement an interface, a class uses the : InterfaceName notation after its class declaration. For example:
kotlin class Circle(val radius: Double) : Shape { override fun area(): Double { return Math.PI * radius * radius } override fun perimeter(): Double { return 2 * Math.PI * radius } }
In this example, the Circle class implements the Shape interface by providing concrete implementations for the area() and perimeter() methods.
Interfaces in Kotlin enable the implementation of polymorphic behavior, allowing objects to be treated uniformly based on their common interface. They facilitate code reuse, maintainability, and extensibility by promoting loose coupling between components.
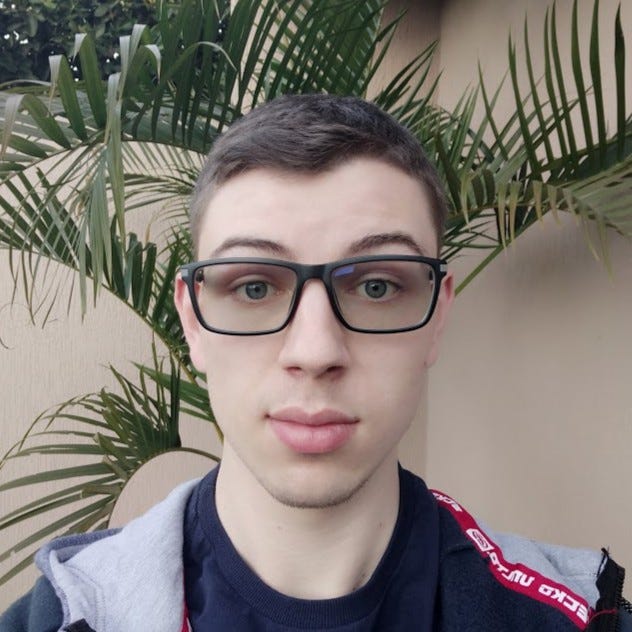
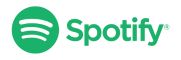