Elevate Your Kotlin App: The Koin Framework for Dependency Injection
In the fast-paced world of modern software development, managing dependencies efficiently is crucial. Dependency Injection (DI) is a design pattern that helps achieve this by providing a way to decouple components and make your code more maintainable and testable. In this blog post, we’ll dive into the world of Kotlin and Dependency Injection, focusing on the Koin framework. So grab your coffee and get ready to explore this powerful combination.
Table of Contents
1. Understanding Dependency Injection
Before we jump into Koin, let’s briefly understand what Dependency Injection is all about. Dependency Injection is a technique where the dependencies required by a class or module are provided from the outside rather than being created within the class itself. This promotes code reusability, modularity, and testability.
2. Kotlin: A Brief Introduction
Kotlin, a statically typed programming language developed by JetBrains, has gained immense popularity in recent years, especially in the Android app development community. Its concise syntax and powerful features make it an excellent choice for building robust and efficient applications.
3. Meet Koin: A Lightweight DI Framework for Kotlin
Koin is a lightweight Dependency Injection framework specifically designed for Kotlin. It offers a simple and elegant way to handle dependency injection in your Kotlin applications. Now, let’s dive into some examples to see how Koin works.
Example 1: Setting Up Koin
To get started with Koin, you need to include the Koin dependency in your project. You can do this by adding the following lines to your Gradle build file:
```kotlin dependencies { implementation "org.koin:koin-android:3.2.0" // Add other dependencies as needed } ```
Next, you need to initialize Koin in your application. Create an Application class and initialize Koin in its `onCreate()` method:
```kotlin class MyApplication : Application() { override fun onCreate() { super.onCreate() startKoin { // Define your modules here } } } ```
Example 2: Defining Modules
In Koin, you define modules that contain the dependencies to be injected. Here’s an example of how to define a module for a hypothetical UserService:
```kotlin val userServiceModule = module { single { UserService(get()) } } ```
In this example, we’re creating a single instance of the `UserService` class. The `get()` function is used to retrieve other dependencies.
Example 3: Injecting Dependencies
Now that we have defined our module, we can inject dependencies into our classes. Here’s how you can inject the `UserService` into a ViewModel:
```kotlin class MyViewModel : ViewModel() { private val userService: UserService by inject() // Use userService in your ViewModel } ```
Koin takes care of providing the `UserService` instance to the ViewModel.
4. Resources for Further Reading
- Official Koin Documentation – https://insert-link-here.com: Dive deep into Koin with the official documentation.
- Koin GitHub Repository – https://github.com/insert-link-here: Explore the source code and contribute to the Koin framework.
- Kotlin Official Website – https://kotlinlang.org/: Learn more about Kotlin and its features.
Conclusion
In this blog post, we’ve explored the powerful combination of Kotlin and the Koin framework for Dependency Injection. By using Koin, you can simplify the management of dependencies in your Kotlin applications, making your code more maintainable and testable. So, whether you’re an early-stage startup founder, a VC investor, or a tech leader, consider incorporating Koin into your tech stack to outperform the competition.
Table of Contents
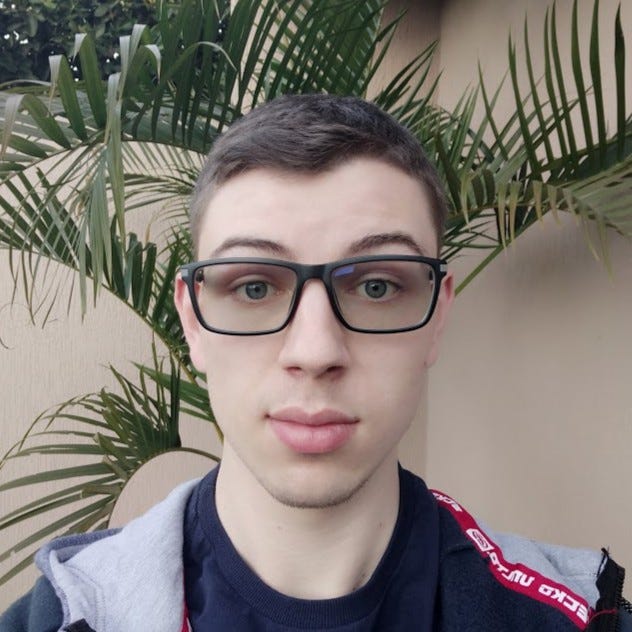
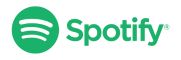