Safeguarding User Data: The Kotlin and Firebase Authentication Duo
In the ever-evolving landscape of mobile app development, ensuring secure user authentication is paramount. Kotlin, the modern and concise programming language, combined with Firebase Authentication, provides a powerful solution for securing user access to your applications. In this post, we’ll delve into the world of Kotlin and Firebase Authentication, exploring their integration, benefits, and providing you with real-world examples.
Table of Contents
1. Why Kotlin and Firebase Authentication?
Before we dive into the technical details, let’s briefly explore why Kotlin and Firebase Authentication make a winning combination.
1.1 Kotlin: The Power of Modern Programming
Kotlin has rapidly gained popularity in the Android development community due to its conciseness, expressiveness, and safety features. It’s fully interoperable with Java, making it an excellent choice for both new and existing Android projects. With Kotlin, you can write clean and maintainable code, reducing the likelihood of security vulnerabilities.
1.2 Firebase Authentication: A Robust Identity Solution
Firebase Authentication is a part of the broader Firebase platform offered by Google. It provides a secure and easy-to-integrate solution for managing user authentication, identity verification, and user data storage. Firebase offers various authentication methods, including email and password, Google Sign-In, and more.
2. Integration of Kotlin and Firebase Authentication
Now, let’s explore how to integrate Kotlin with Firebase Authentication.
2.1. Setting Up Firebase Project
To get started, create a Firebase project in the Firebase Console – https://console.firebase.google.com/.
Once your project is set up, you’ll receive a configuration file that you’ll include in your Kotlin project.
2.2. Adding Firebase to Your Kotlin Project
In your Kotlin project, add Firebase Authentication as a dependency. You can do this by adding the following line to your app-level `build.gradle` file:
```kotlin implementation 'com.google.firebase:firebase-auth:21.0.0' ```
2.3. Initialize Firebase
In your Kotlin code, initialize Firebase Authentication with the configuration file you received when setting up your Firebase project. Here’s an example of how to initialize Firebase in your application:
```kotlin // Import necessary Firebase modules import com.google.firebase.auth.FirebaseAuth import com.google.firebase.auth.FirebaseUser // Initialize Firebase Authentication val auth = FirebaseAuth.getInstance() ```
2.4. User Authentication
You can now use Firebase Authentication to handle user registration and login. Here’s an example of registering a new user with an email and password:
```kotlin // Create a new user with email and password auth.createUserWithEmailAndPassword(email, password) .addOnCompleteListener(this) { task -> if (task.isSuccessful) { // Registration successful val user = auth.currentUser } else { // Registration failed // Handle errors here } } ```
2.5. User Sign-In
To allow existing users to sign in, use the following code:
```kotlin // Sign in with email and password auth.signInWithEmailAndPassword(email, password) .addOnCompleteListener(this) { task -> if (task.isSuccessful) { // Sign-in successful val user = auth.currentUser } else { // Sign-in failed // Handle errors here } } ```
2.6. Additional Authentication Methods
Firebase Authentication offers various methods, including Google Sign-In, Facebook Login, and more. You can provide multiple options for user authentication in your Kotlin app.
3. Secure User Authentication Best Practices
- Password Policies: Enforce strong password policies to protect user accounts.
- OAuth Providers: Implement OAuth providers for additional security layers.
- Multi-Factor Authentication (MFA): Encourage users to enable MFA for added account security.
- Error Handling: Implement proper error handling to provide clear feedback to users during authentication processes.
- Session Management: Manage user sessions securely, including token expiration and refresh mechanisms.
- Security Auditing: Regularly audit your authentication system for vulnerabilities and updates.
Conclusion
Incorporating Kotlin and Firebase Authentication into your mobile app development process provides a robust and secure solution for user authentication. With Kotlin’s modern capabilities and Firebase Authentication’s comprehensive features, you can create user-friendly and highly secure applications.
By following best practices and staying updated on security measures, you’ll be well-equipped to protect your users’ data and ensure a seamless authentication experience.
Remember, security is an ongoing process, and staying vigilant is key to maintaining the trust of your users.
Further Reading:
- Firebase Authentication Documentation – https://firebase.google.com/docs/auth
- Kotlin Programming Language – https://kotlinlang.org/
- OWASP Mobile Security Testing Guide – https://owasp.org/www-project-mobile-security-testing-guide/
Table of Contents
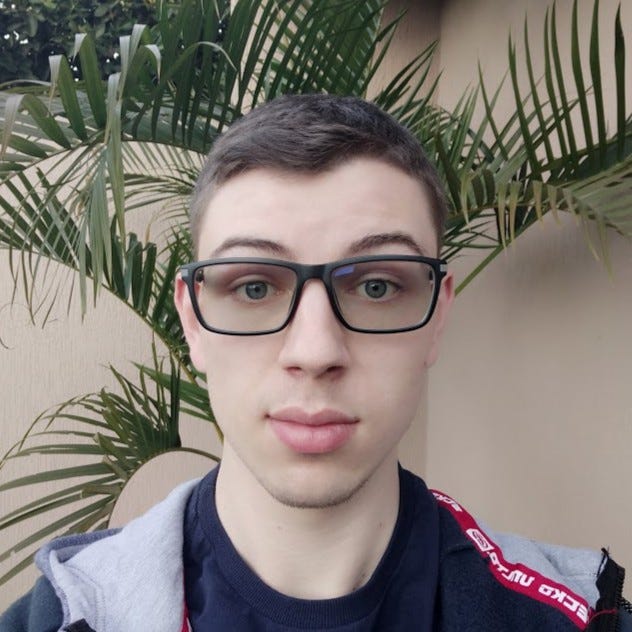
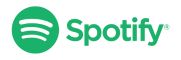