From Idea to Impact: Mastering Real-Time Data with Kotlin and Firebase
In the fast-paced world of tech startups, staying ahead of the competition often means having the right tools and strategies in place. For early-stage startup founders, especially those in the Seed to Series C stage, leveraging the power of Kotlin and Firebase Realtime Database can be a game-changer. In this post, we’ll explore how these technologies can help you sync data in real-time and provide examples of their implementation.
Table of Contents
1. Why Real-Time Data Sync Matters
Before diving into the technical aspects, let’s briefly discuss why real-time data synchronization is crucial for startups. In a competitive landscape, having up-to-date information at your fingertips can make all the difference. Here are some reasons why real-time data sync is vital:
1.1. Instant User Engagement
For startups building mobile or web applications, real-time updates can enhance user engagement. Imagine a messaging app where new messages appear instantly or a collaborative document editor where changes are visible in real time. These features keep users engaged and satisfied.
1.2. Data Accuracy
Real-time data sync ensures that your users are always working with the latest information. Whether it’s stock prices, sports scores, or collaborative project management, accurate data is essential for informed decision-making.
1.3. Competitive Edge
In the startup world, speed is often the key to success. Real-time data synchronization allows you to respond quickly to market changes, customer feedback, or emerging trends, giving you a competitive edge.
2. Kotlin and Firebase Realtime Database: A Powerful Combination
Now that we understand the importance of real-time data sync, let’s explore how Kotlin and Firebase Realtime Database can help you achieve this.
2.1 Kotlin: The Language of Choice
Kotlin, a statically typed programming language developed by JetBrains, has gained significant popularity in the Android app development community. It seamlessly integrates with Java, making it an ideal choice for developers who want modern features without sacrificing compatibility.
With Kotlin, you can write concise and expressive code, reducing the chances of errors and increasing development speed. Its null safety feature helps prevent null pointer exceptions, a common source of bugs.
3. Firebase Realtime Database: Real-Time Data Storage
Firebase Realtime Database is a cloud-hosted NoSQL database that allows you to store and synchronize data in real-time. It’s a part of the Firebase platform by Google, designed to streamline backend development for web and mobile applications.
Here’s how Firebase Realtime Database works:
- Data Structure: The database is organized as a JSON tree, which is both flexible and intuitive. You can nest data and create complex structures to suit your application’s needs.
- Real-Time Sync: Firebase provides SDKs for various platforms, including Android with Kotlin support. When data changes in the database, connected clients receive updates in real-time without the need for manual refreshes.
- Authentication: Firebase offers robust authentication options, ensuring that only authorized users can access and modify data.
Now, let’s dive into some practical examples of how Kotlin and Firebase Realtime Database can be used together:
Example 1: Real-Time Chat Application
Imagine you’re building a real-time chat application for your startup. With Kotlin and Firebase Realtime Database, you can create a chat interface where messages appear instantly as users send them. Here’s a simplified code snippet to demonstrate:
```kotlin // Create a reference to the chat messages in Firebase val messagesRef = FirebaseDatabase.getInstance().getReference("chat_messages") // Listen for new messages messagesRef.addChildEventListener(object : ChildEventListener { override fun onChildAdded(snapshot: DataSnapshot, previousChildName: String?) { val newMessage = snapshot.getValue(ChatMessage::class.java) // Display the new message in the chat interface } // ... }) ```
This code sets up a listener that triggers when new messages are added to the database, providing a seamless real-time chat experience for your users.
Example 2: Live Dashboard for Analytics
For data-driven startups, having a live dashboard that displays real-time analytics can be a game-changer. Kotlin and Firebase Realtime Database make it possible to update charts and graphs in real time as new data points come in.
By storing analytics data in Firebase, you can easily sync it with your Kotlin-powered dashboard. Here’s a high-level example:
```kotlin // Create a reference to the analytics data in Firebase val analyticsRef = FirebaseDatabase.getInstance().getReference("analytics_data") // Listen for changes in analytics data analyticsRef.addValueEventListener(object : ValueEventListener { override fun onDataChange(snapshot: DataSnapshot) { // Update the dashboard with the latest analytics data } // ... }) ```
In this scenario, your dashboard remains up to date with the most recent user behavior and performance metrics.
Example 3: Real-Time Collaboration Tool
If your startup is focused on collaboration tools, Kotlin and Firebase Realtime Database can help you build features like real-time document editing, task management, or whiteboarding. Users can collaborate seamlessly without worrying about version conflicts.
To demonstrate, here’s a simplified example of real-time document editing:
```kotlin // Create a reference to the shared document in Firebase val documentRef = FirebaseDatabase.getInstance().getReference("shared_document") // Listen for changes in the document content documentRef.addValueEventListener(object : ValueEventListener { override fun onDataChange(snapshot: DataSnapshot) { val documentContent = snapshot.getValue(String::class.java) // Update the document editor with the latest content } // ... }) ```
With Firebase Realtime Database, changes made by one user are instantly reflected to others, creating a smooth collaborative experience.
Conclusion
In the competitive world of tech startups, real-time data synchronization is a valuable asset. Kotlin’s modern features and Firebase Realtime Database’s real-time capabilities make them a potent combination for early-stage founders, investors, and tech leaders.
Whether you’re building a real-time chat app, a live analytics dashboard, or a collaborative tool, Kotlin and Firebase Realtime Database empower you to provide the real-time experiences your users crave. Embrace these technologies, and you’ll be well on your way to outperforming the competition.
External Resources:
- Kotlin Programming Language – https://kotlinlang.org/
- Firebase Realtime Database Documentation – https://firebase.google.com/docs/database
- Firebase Authentication – https://firebase.google.com/docs/auth
Table of Contents
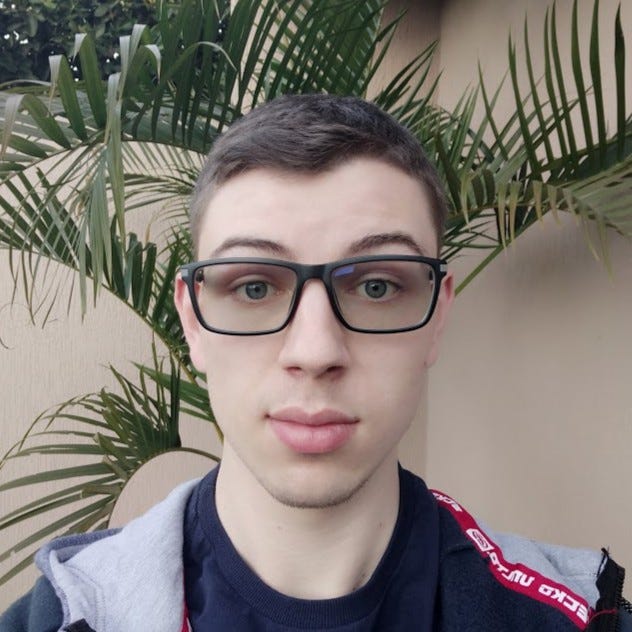
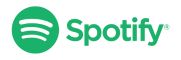