Mastering Kotlin Generics: Writing Type-Safe Code
Kotlin, with its concise syntax and powerful features, has rapidly gained popularity in the developer community. One of the essential features that make Kotlin a favorite among developers is its support for generics. Generics enable developers to write type-safe and flexible code, ensuring that potential runtime errors are caught at compile-time. In this blog, we’ll dive deep into Kotlin generics and learn how to leverage them effectively to write robust and type-safe code. Whether you’re a beginner or an experienced Kotlin developer, mastering generics will undoubtedly take your coding skills to the next level.
1. Understanding Generics
Generics allow us to create classes, interfaces, and functions that can work with different types while maintaining type safety. The primary benefit of using generics is to avoid code duplication and enable a high level of code reuse. Instead of writing separate methods or classes for each specific data type, generics allow us to write code that can handle a wide range of data types, providing the added advantage of compile-time type-checking.
1.1. Generic Classes
Let’s start by exploring generic classes in Kotlin. A generic class is a class that takes one or more type parameters, allowing us to define placeholders for the types that will be used later. We use angle brackets (<>) to specify the type parameters.
kotlin class Box<T>(val item: T) { fun getItem(): T = item }
In the example above, we’ve defined a generic class called Box. The type parameter T acts as a placeholder for the actual type that will be used when creating an instance of Box. We can create instances of Box with different types:
kotlin val box1: Box<Int> = Box(42) val box2: Box<String> = Box("Kotlin Generics")
Now, box1 will hold an integer value, and box2 will hold a string.
1.2. Generic Functions
Like classes, we can also define generic functions. A generic function allows us to operate on different types without the need to create separate functions for each type.
kotlin fun <T> printItem(item: T) { println(item) }
The printItem function above takes a type parameter T and prints the passed item. We can call this function with various data types:
kotlin printItem(42) // Output: 42 printItem("Kotlin Generics") // Output: Kotlin Generics
1.3. Generic Constraints
Sometimes, we need to restrict the types that can be used with a generic class or function. We can achieve this by using generic constraints. By specifying constraints, we ensure that only certain types can be used with the generic class or function.
For example, let’s create a generic function that works with types that implement the Comparable interface:
kotlin fun <T : Comparable<T>> findMax(a: T, b: T): T { return if (a > b) a else b }
In this example, we used the syntax <T : Comparable<T>> to specify that the type parameter T must implement the Comparable interface. This constraint allows us to use comparison operators (>, <, >=, <=) inside the function body.
1.4. Variance in Generics
Understanding variance is crucial when working with Kotlin generics, especially when dealing with subtyping relationships. In Kotlin, we have three main variance annotations: out, in, and invariant.
- Covariance (out): We use out to mark a type parameter as covariant. It means that the generic class can produce instances of T but cannot consume them. We often use out with read-only data structures.
kotlin interface Producer<out T> { fun produce(): T }
- Contravariance (in): We use in to mark a type parameter as contravariant. It means that the generic class can consume instances of T but cannot produce them. We often use in with write-only data structures.
kotlin interface Consumer<in T> { fun consume(item: T) }
- Invariant: If we don’t specify any variance annotation, the type parameter is considered invariant. It means that the generic class can both produce and consume instances of T.
kotlin class Box<T>(val item: T)
2. Reified Type Parameters
Kotlin allows us to use the reified keyword with inline functions to obtain type information at runtime. This feature is especially useful when working with generic functions.
kotlin inline fun <reified T> getClassName(): String { return T::class.simpleName ?: "Unknown" }
The getClassName function above returns the name of the class represented by the type parameter T. We can call this function as follows:
kotlin val className = getClassName<Int>() println(className) // Output: Int
3. Type Erasure
It’s important to note that Kotlin, like Java, suffers from type erasure. This means that generic type information is not available at runtime. The type arguments used with generic classes and functions are removed during compilation. As a result, the JVM doesn’t know the actual type used with generics at runtime.
kotlin class Box<T>(val item: T) val box = Box(42)
In the example above, the type T is erased during compilation, and box is equivalent to Box<Any>(42) at runtime.
4. Use Cases of Kotlin Generics
Kotlin generics can significantly enhance the design and safety of your code. Let’s explore some practical use cases where generics shine.
4.1. Collections and Data Structures
Generics are widely used in Kotlin’s standard library, especially in collections and data structures. The most common example is the List<T> interface, which is generic and allows you to create lists of various types.
kotlin val numbers: List<Int> = listOf(1, 2, 3, 4, 5) val names: List<String> = listOf("Alice", "Bob", "Charlie")
The generic List<T> enables type safety and ensures that you cannot insert elements of the wrong type into the list.
4.2. Custom Data Containers
Using generics, you can create custom data containers that work with various data types, providing a higher level of code reusability.
kotlin class Pair<T, U>(val first: T, val second: U) val stringIntPair: Pair<String, Int> = Pair("one", 1) val doubleCharPair: Pair<Double, Char> = Pair(3.14, 'A')
The Pair class can store a pair of values with different types, giving you the flexibility to use it with various data types.
4.3. Type-Safe Builders
Generics play a crucial role in type-safe builders, which allow you to create domain-specific languages (DSLs) that provide a natural and expressive way to define complex structures.
kotlin fun buildPerson(block: PersonBuilder.() -> Unit): Person { val builder = PersonBuilder() builder.block() return builder.build() } class PersonBuilder { var name: String = "" var age: Int = 0 fun build(): Person { return Person(name, age) } } val person = buildPerson { name = "John" age = 30 }
In the example above, we create a type-safe builder for a Person class. The DSL allows us to construct a Person object using natural language-like syntax.
5. Best Practices for Writing Type-Safe Code with Generics
While Kotlin generics provide immense flexibility, they can also introduce complexity if not used carefully. Here are some best practices to follow when working with generics:
5.1. Use Descriptive Type Parameter Names
When defining generic classes or functions, use descriptive names for type parameters. This will make your code more readable and understandable.
kotlin class Box<T> { ... } // Not recommended class Box<ItemType> { ... } // Recommended
5.2. Utilize Generic Constraints
Leverage generic constraints to restrict the types that can be used with your generic classes or functions. This helps prevent unexpected behaviors and improves the robustness of your code.
5.3. Avoid Explicit Type Casting
Try to avoid explicit type casting within generic code, as it can lead to runtime errors. Instead, use generic constraints or other techniques to ensure type safety.
5.4. Be Mindful of Variance
Understand the variance annotations (out, in, and invariant) and use them appropriately. Incorrect use of variance can lead to compiler errors or runtime exceptions.
5.5. Test Your Code Thoroughly
As with any complex feature, test your code thoroughly with different data types and scenarios to ensure it behaves as expected.
Conclusion
Kotlin generics are a powerful tool in a developer’s arsenal for writing type-safe and flexible code. By creating generic classes, functions, and leveraging generic constraints, you can enhance code reusability and safety. Remember to follow best practices and understand the variance annotations to make the most of Kotlin’s generics. With this newfound knowledge, you’ll be well-equipped to write robust and type-safe code in your Kotlin projects. Happy coding!
Table of Contents
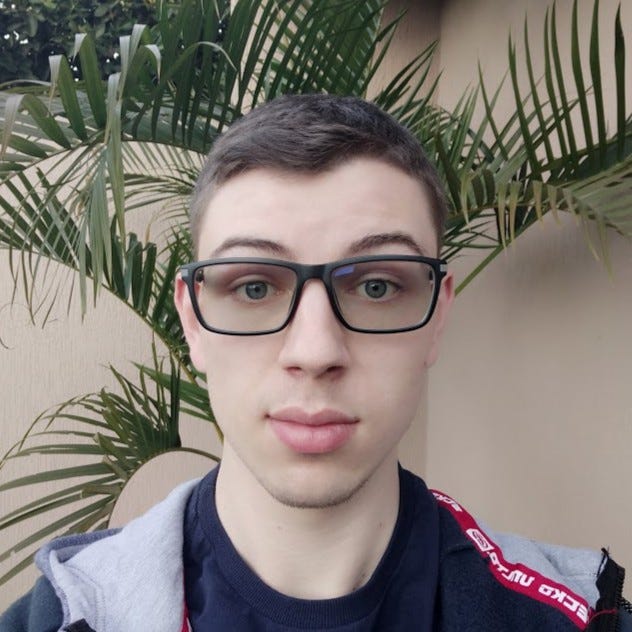
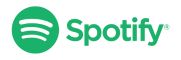