Visual Intelligence: The Kotlin Developer’s Guide to Google Cloud Vision
In the ever-evolving world of mobile and web applications, incorporating cutting-edge technologies can set your product apart. One such technology that’s gaining prominence is image recognition, and with Kotlin, Google Cloud Vision, and a dash of creativity, you can bring this powerful feature to your apps. In this blog post, we’ll explore the possibilities of integrating image recognition into your Kotlin-based applications.
Table of Contents
1. The Power of Image Recognition
Image recognition, also known as computer vision, allows machines to interpret and understand the visual world. With the rise of smartphones and the proliferation of images and videos on the internet, this technology has become increasingly important. Here are a few scenarios where image recognition can be a game-changer:
- E-commerce: Imagine building a shopping app where users can simply snap a picture of an item, and the app instantly finds similar products in your inventory.
- Healthcare: Integrating image recognition can help identify diseases, analyze X-rays, and provide early diagnosis, saving lives.
- Security: Enhance security with facial recognition or object detection for access control systems.
- Social Media: Automatically tag friends in photos, identify objects in images, and create immersive augmented reality experiences.
Now, let’s dive into how you can implement image recognition in your Kotlin apps using Google Cloud Vision.
2. Setting Up Google Cloud Vision
Before you begin, make sure you have a Google Cloud Platform (GCP) account and have enabled the Google Cloud Vision API. Here’s a step-by-step guide:
- Create a GCP Project: Log in to your GCP Console, create a new project, and select it.
- Enable Billing: To use the Vision API, you need to enable billing for your project.
- Enable the Vision API: In the GCP Console, go to the APIs & Services > Dashboard, and click on “+ ENABLE APIS AND SERVICES.” Search for “Cloud Vision API” and enable it.
- Set Up Authentication: Create a service account key, download the JSON key file, and set the `GOOGLE_APPLICATION_CREDENTIALS` environment variable to point to the JSON key file.
Now that you’ve set up Google Cloud Vision, let’s move on to integrating it with your Kotlin application.
3. Kotlin and Google Cloud Vision Integration
Step 1: Add Dependencies
In your Kotlin project, open the `build.gradle` file and add the Google Cloud Vision dependency:
```kotlin dependencies { implementation 'com.google.cloud:google-cloud-vision:2.3.1' } ```
Step 2: Write Kotlin Code
Here’s a Kotlin code snippet that demonstrates image recognition using Google Cloud Vision. We’ll focus on label detection as an example:
```kotlin import com.google.cloud.vision.v1.Image import com.google.cloud.vision.v1.ImageAnnotatorClient import com.google.cloud.vision.v1.ImageAnnotatorSettings import com.google.protobuf.ByteString import java.nio.file.Files import java.nio.file.Path import java.nio.file.Paths fun detectLabels(filePath: String): List<String> { val imageBytes = Files.readAllBytes(Paths.get(filePath)) val image = Image.newBuilder().setContent(ByteString.copyFrom(imageBytes)).build() val settings = ImageAnnotatorSettings.newBuilder() .setCredentialsProvider(FixedCredentialsProvider.create(credentials)) .build() ImageAnnotatorClient.create(settings).use { vision -> val response = vision.labelDetection(image) val labels = response.labelAnnotationsList.map { it.description } return labels } } ```
In this code, we read an image file, send it to Google Cloud Vision for label detection, and retrieve the detected labels.
Step 3: Execute the Function
To use the `detectLabels` function, simply call it with the file path of the image you want to analyze:
```kotlin val labels = detectLabels("path/to/your/image.jpg") labels.forEach { println(it) } ```
Conclusion
In this blog post, we explored how to harness the power of image recognition in your Kotlin applications using Google Cloud Vision. We covered the setup process, added the necessary dependencies, and provided a Kotlin code example for label detection.
The potential applications of image recognition are vast and can add tremendous value to your apps, from simplifying e-commerce to revolutionizing healthcare. By combining the capabilities of Kotlin with Google Cloud Vision, you can create innovative and feature-rich applications that outperform the competition.
For more information and resources on Kotlin, Google Cloud Vision, and image recognition, check out these external links:
- Google Cloud Vision API Documentation – https://cloud.google.com/vision/docs
- Kotlin Programming Language Official Website – https://kotlinlang.org/
- OpenCV Library for Computer Vision in Kotlin – https://opencv.org/
Table of Contents
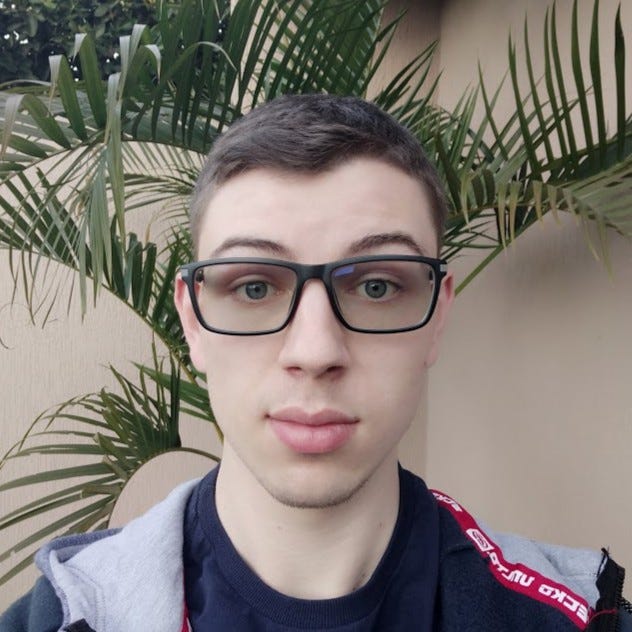
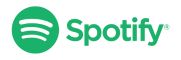