How Inline Functions Can Transform Your Kotlin Development Experience
Kotlin is a modern and expressive programming language that boasts of a rich set of features, which empower developers to write cleaner, more concise, and efficient code. Among these features, inline functions are a potent tool for optimization, especially when dealing with higher-order functions.
In this blog post, we’ll delve into the intricacies of inline functions, examining how and when to use them, as well as the benefits they bring to the table.
1. What are Inline Functions?
In Kotlin, when you define a function as `inline`, the compiler does not generate a new method for this function during compilation. Instead, it embeds the function body directly into the place where the function is called. This eliminates the overhead of a function call, which can be particularly beneficial for short functions and lambda expressions.
The Syntax
Here’s how you can define an inline function:
```kotlin inline fun simpleInlineFunction() { // Your code here } ```
The Benefits of Using Inline Functions
- Performance optimization: Reducing the call overhead, especially in frequently called functions, can yield performance improvements.
- Lambdas optimization: When passing lambdas to inline functions, the lambdas are inlined too, eliminating the object allocation and invocation overhead for them.
- Accessing non-local returns: Non-local returns from lambdas are only possible when the lambda is passed to an inline function.
2. Examples to Illustrate Inline Functions
Let’s look at some practical scenarios to understand the use-cases of inline functions better.
- Basic Inline Function
Consider a simple function that prints a message:
```kotlin inline fun printMessage(message: String) { println(message) } fun main() { printMessage("Hello, Kotlin!") } ```
During compilation, the code inside `printMessage` will be directly embedded into the `main` function. So, the resultant bytecode will be equivalent to:
```kotlin fun main() { println("Hello, Kotlin!") } ```
2. Inline with Lambdas
Inline functions shine when used with lambdas:
```kotlin inline fun operateOnNumbers(a: Int, b: Int, operation: (Int, Int) -> Int): Int { return operation(a, b) } fun main() { val result = operateOnNumbers(5, 3) { x, y -> x + y } println(result) // Outputs: 8 } ```
Here, not only is the `operateOnNumbers` function inlined, but the lambda `{ x, y -> x + y }` is also inlined, eliminating any overhead associated with its invocation.
4. Non-local Returns
A standout feature of inline functions is their ability to handle non-local returns:
```kotlin inline fun loopThroughList(list: List<Int>, predicate: (Int) -> Boolean) { for (item in list) { if (predicate(item)) { return } } println("Loop completed.") } fun main() { val numbers = listOf(1, 2, 3, 4, 5) loopThroughList(numbers) { if (it == 3) return println(it) } println("End of main.") } ```
The output will be:
``` 1 2 End of main. ```
When the predicate finds the number 3, it returns not just from the lambda, but from the entire `main` function thanks to the inline property.
5. Things to be Aware Of
- Code bloat: Overusing inline functions can lead to increased code size because the same code is copied to multiple places.
- Binary compatibility: As inline functions embed code at the call site, changes in the inline function might lead to the need to recompile all the calling code.
Conclusion
Kotlin’s inline functions provide a powerful mechanism for optimization, especially when working with higher-order functions and lambda expressions. By understanding when and how to use them, developers can tap into more efficient code generation and runtime performance. However, like any tool, they should be used judiciously, keeping potential pitfalls in mind.
Table of Contents
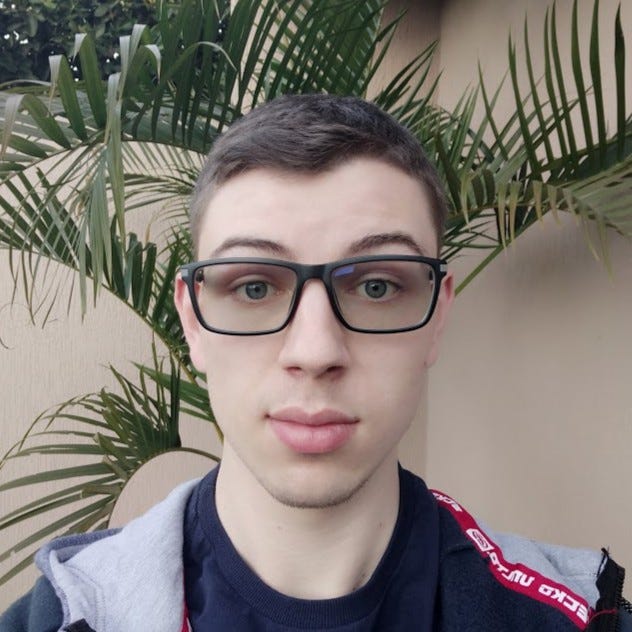
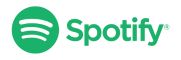