Unlock the Power of Kotlin Type Aliases in Your Development Workflow
In the fast-paced world of software development, every line of code counts. Efficiency and readability are key to maintaining and scaling your projects. Kotlin, a popular programming language for Android app development and beyond, offers various features that make your code concise and expressive. One such feature that can significantly improve code clarity is Kotlin Type Aliases.
Table of Contents
1. What Are Kotlin Type Aliases?
Kotlin Type Aliases allow developers to create alternative names for existing types. They don’t introduce new types; instead, they provide a convenient way to make your code more readable and expressive by giving complex types a more human-friendly name.
Let’s delve deeper into the benefits of Kotlin Type Aliases with some real-world examples.
Example 1: Simplifying Data Classes
Imagine you’re building a startup’s financial app, and you have a data class representing a financial transaction. The transaction may have a timestamp represented by a Long, an amount as a Double, and various other properties. Without Kotlin Type Aliases, your code might look like this:
```kotlin data class FinancialTransaction( val timestamp: Long, val amount: Double, val description: String, // ... other properties ) ```
While this code is functional, it can become less readable as your project grows. Enter Kotlin Type Aliases:
```kotlin typealias Timestamp = Long typealias Amount = Double data class FinancialTransaction( val timestamp: Timestamp, val amount: Amount, val description: String, // ... other properties ) ```
By using Type Aliases, you’ve made the code cleaner and more self-explanatory. Now, anyone reading your code instantly understands that `Timestamp` refers to a Long and `Amount` is a Double.
Example 2: Managing Complex Lambdas
In the tech world, functional programming is gaining momentum. You might find yourself working with complex lambda expressions. Type Aliases can simplify this as well. Consider this example:
```kotlin val validateUserInput: (String, (Boolean) -> Unit) -> Unit = // Complex lambda ```
It’s not immediately clear what this lambda represents. But with a Type Alias:
```kotlin typealias UserInputValidator = (String, (Boolean) -> Unit) -> Unit val validateUserInput: UserInputValidator = // Much clearer ```
The code now reads more intuitively, making it easier to understand the purpose of `validateUserInput`.
Example 3: Handling Custom Error Types
Startups often encounter unique challenges that require custom error handling. Kotlin Type Aliases can enhance the clarity of error handling code:
```kotlin typealias CustomError = Pair<String, Int> fun processCustomError(error: CustomError) { // Handle the error here } ```
Now, you’ve encapsulated the error information into a more descriptive type, making your error-handling code more self-explanatory.
Conclusion
Kotlin Type Aliases are a powerful tool for improving code readability and maintainability. By assigning human-friendly names to complex types, you can enhance collaboration among your team and make your code more accessible to future developers.
In the dynamic world of tech startups and investments, every second counts. Simplifying your codebase with Kotlin Type Aliases is a small step that can have a significant impact on your project’s success. So, embrace the power of Kotlin Type Aliases and watch your code become more expressive, efficient, and enjoyable to work with.
Table of Contents
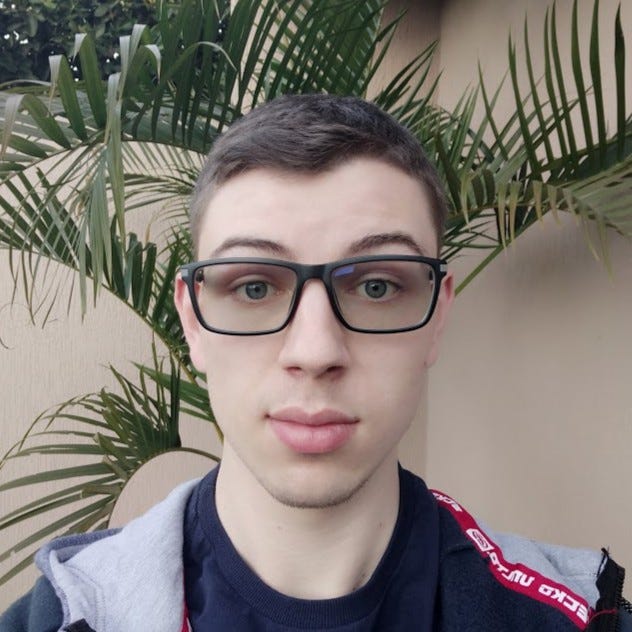
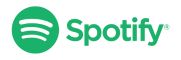