How are loops implemented in Kotlin?
In Kotlin, loops are implemented using various constructs that facilitate iteration over collections, arrays, ranges, and other data structures. Kotlin provides several loop constructs, including the traditional for loop, the enhanced for..in loop, and functional-style operations such as forEach() and forEachIndexed().
One of the most common loop constructs in Kotlin is the for loop, which iterates over a range of values or any iterable object. For example:
kotlin for (i in 1..10) { println(i) }
This loop iterates from 1 to 10, inclusive, and prints each value of i.
Kotlin also supports the while loop, which executes a block of code repeatedly as long as the specified condition is true. Here’s an example:
kotlin var x = 0 while (x < 5) { println(x) x++ }
This loop prints values of x from 0 to 4.
Additionally, Kotlin offers functional-style iteration methods such as forEach() and forEachIndexed(), which allow concise iteration over collections without explicit index management. For instance:
kotlin val list = listOf("apple", "banana", "orange") list.forEach { println(it) }
This code iterates over each element of the list and prints it.
Kotlin’s support for functional programming paradigms enables developers to write expressive and concise code for iteration tasks. Whether it’s iterating over collections, arrays, or ranges, Kotlin provides flexible and efficient loop constructs to suit various programming scenarios.
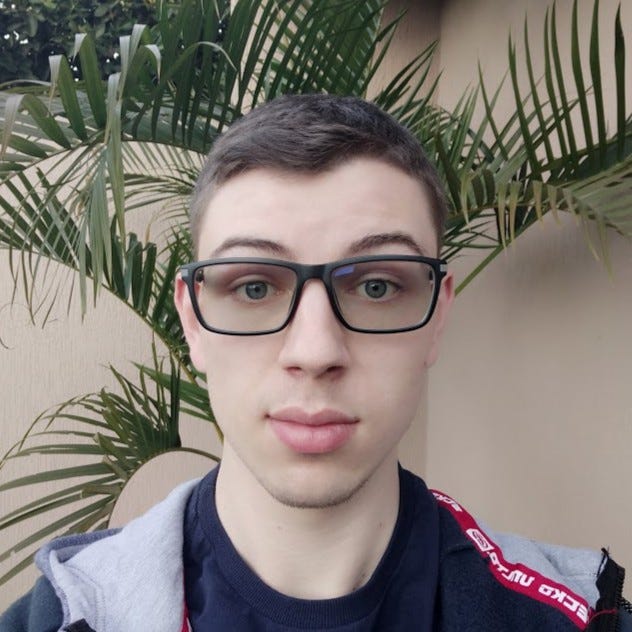
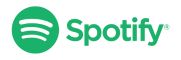