Building Reactive Applications with Kotlin and Spring Boot
Reactive programming has gained significant popularity in recent years due to its ability to handle large volumes of data and high-concurrency scenarios. In this blog, we’ll explore how you can leverage Kotlin, a modern programming language, and Spring Boot, a popular Java-based framework, to build reactive applications. We’ll delve into key concepts, best practices, and provide code samples to help you get started with building efficient and scalable reactive systems.
1. Understanding Reactive Programming
1.1 What is Reactive Programming?
Reactive programming is an asynchronous programming paradigm that focuses on building systems that react to events and handle streams of data. It enables developers to write code that is more efficient, scalable, and resilient in handling concurrent and event-driven scenarios.
1.2 Key Concepts in Reactive Programming
Reactive programming revolves around a few fundamental concepts:
- Reactive Streams: A specification that provides a standard for asynchronous stream processing with non-blocking backpressure.
- Publisher: Emits a stream of data or events.
- Subscriber: Consumes the stream of data or events emitted by the Publisher.
- Subscription: Represents the relationship between the Publisher and the Subscriber.
- Backpressure: Mechanism to handle situations when the subscriber is overwhelmed by the rate of data emitted by the publisher.
1.3 Benefits of Reactive Programming
Reactive programming offers several benefits, including:
- Asynchronous and non-blocking: Reactive applications can handle a large number of concurrent requests efficiently.
- Scalability: Reactive systems can scale seamlessly to handle varying workloads.
- Resilience: Reactive programming provides built-in error handling and fault tolerance mechanisms.
- Efficiency: Reactive applications can optimize resource utilization and reduce response times.
2. Introduction to Kotlin
2.1 Why Choose Kotlin for Reactive Applications?
Kotlin is a modern, statically typed programming language that has gained immense popularity due to its conciseness, null safety, and seamless interoperability with existing Java codebases. Kotlin’s functional programming features and coroutine support make it an excellent choice for building reactive applications.
2.2 Kotlin Features for Reactive Programming
Kotlin provides several features that are well-suited for reactive programming, including:
- Coroutines: Kotlin coroutines simplify asynchronous programming by providing a structured and sequential approach to handling asynchronous tasks.
- Extension Functions: Kotlin’s extension functions allow you to add reactive capabilities to existing classes or libraries.
- Functional Programming Constructs: Kotlin supports functional programming paradigms, such as higher-order functions, lambda expressions, and immutability, which are valuable in building reactive systems.
3. Getting Started with Spring Boot
3.1 Setting Up a Spring Boot Project
To get started with building reactive applications using Kotlin and Spring Boot, you’ll need to set up a Spring Boot project. You can use Spring Initializr (start.spring.io) or your favorite IDE to create a new project with the necessary dependencies.
3.2 Configuring Dependencies for Reactive Programming
In your build.gradle (or pom.xml), add the required dependencies for building reactive applications with Kotlin and Spring Boot. Some essential dependencies include:
- Spring Boot Starter Webflux: Provides the necessary components for building reactive web APIs.
- Kotlin Coroutines: Enables seamless integration of Kotlin coroutines for asynchronous programming.
- Spring Data MongoDB (or your preferred reactive database driver): Allows interaction with reactive databases.
4. Building Reactive Web APIs
4.1 Creating Reactive Controllers
In Spring Boot, you can define reactive controllers using annotations, similar to traditional Spring MVC controllers. Annotate your controller class with @RestController and individual methods with @GetMapping, @PostMapping, etc., to handle HTTP requests. Use Kotlin’s suspend modifier to indicate that a method is a coroutine.
kotlin @RestController class UserController(private val userRepository: UserRepository) { @GetMapping("/users") suspend fun getUsers(): List<User> { return userRepository.findAll().toList() } @PostMapping("/users") suspend fun createUser(@RequestBody user: User): User { return userRepository.save(user) } // Other CRUD operations... }
4.2 Handling Asynchronous Operations with Kotlin Coroutines
Kotlin coroutines provide a concise and expressive way to handle asynchronous operations. You can use the suspend modifier to mark functions that perform asynchronous tasks. Inside a coroutine, you can use suspend functions provided by Spring, such as repository methods, to interact with the database asynchronously.
kotlin @Repository interface UserRepository : CoroutineCrudRepository<User, String> { suspend fun findByEmail(email: String): User? // Other repository methods... }
4.3 Integrating Spring WebFlux for Reactive Endpoints
Spring WebFlux is a part of Spring Framework 5 that provides reactive programming support for building web applications. It integrates seamlessly with Kotlin coroutines and allows you to create reactive endpoints using functional or annotated programming models.
kotlin @Configuration class WebConfig { @Bean fun routerFunction(userHandler: UserHandler): RouterFunction<ServerResponse> { return coRouter { "/users".nest { GET("/", userHandler::getAllUsers) POST("/", userHandler::createUser) // Other routes... } } } } @Component class UserHandler(private val userRepository: UserRepository) { suspend fun getAllUsers(request: ServerRequest): ServerResponse { val users = userRepository.findAll().toList() return ServerResponse.ok().bodyValue(users) } suspend fun createUser(request: ServerRequest): ServerResponse { val user = request.awaitBody<User>() val savedUser = userRepository.save(user) return ServerResponse.created(URI("/users/${savedUser.id}")).build() } // Other handler methods... }
5. Reactive Database Access
5.1 Working with Reactive Database Drivers
To interact with reactive databases, you’ll need to use the appropriate reactive database driver. For example, if you’re using MongoDB, you can use Spring Data MongoDB with its reactive support. Configure the database connection and repositories to work in a reactive manner.
kotlin @Configuration @EnableReactiveMongoRepositories class MongoConfig : AbstractReactiveMongoConfiguration() { override fun getDatabaseName(): String { return "my-database" } override fun reactiveMongoClient(): MongoClient { return MongoClients.create() } override fun getMappingBasePackage(): String { return "com.example.myapp.model" } } @Repository interface UserRepository : ReactiveMongoRepository<User, String> { fun findByEmail(email: String): Mono<User> // Other repository methods... }
5.2 Implementing Reactive Data Repositories with Spring Data
Spring Data provides support for building reactive data repositories. You can define reactive repository interfaces and use the provided query methods to interact with the database asynchronously.
kotlin @Repository interface UserRepository : ReactiveCrudRepository<User, String> { fun findByEmail(email: String): Mono<User> // Other repository methods... }
6. Reactive Streams and Backpressure
6.1 Understanding Reactive Streams
Reactive Streams is a specification that provides a common set of interfaces, methods, and protocols for building asynchronous and non-blocking stream processing systems. It helps handle backpressure and ensures the flow of data between publishers and subscribers is managed efficiently.
6.2 Dealing with Backpressure in Reactive Applications
Backpressure is a mechanism to handle situations when the subscriber is unable to keep up with the rate at which the publisher is emitting data. Reactive libraries like Reactor and RxJava provide operators and strategies to handle backpressure effectively, ensuring that the system remains responsive and doesn’t get overwhelmed with excessive data.
7. Testing Reactive Applications
7.1 Unit Testing Reactive Components
When testing reactive components, you can use the built-in testing utilities provided by Spring and reactive libraries. Use Kotlin’s coroutine test support to write concise and expressive tests for your reactive code.
kotlin @RunWith(SpringRunner::class) @WebFluxTest(UserController::class) class UserControllerTests { @Autowired private lateinit var webTestClient: WebTestClient @MockBean private lateinit var userRepository: UserRepository @Test fun testGetUsers() { val user1 = User("1", "John") val user2 = User("2", "Jane") Mockito.`when`(userRepository.findAll()).thenReturn(Flux.just(user1, user2)) webTestClient.get() .uri("/users") .exchange() .expectStatus().isOk() .expectBodyList(User::class.java) .hasSize(2) .contains(user1, user2) } // Other unit tests... }
7.2 Integration Testing Reactive Endpoints
For integration testing, you can use tools like JUnit and Spring Test to test your reactive endpoints with an embedded server. You can send requests and verify responses using the provided testing APIs.
8. Deployment and Scaling
8.1 Deploying Reactive Applications
To deploy reactive applications, you can use traditional deployment methods like containerization (Docker) or deploy them on cloud platforms (AWS, Azure, GCP). Ensure that your deployment environment is configured to handle reactive workloads efficiently.
8.2 Scaling Reactive Applications
Reactive applications can scale horizontally by adding more instances to handle increased workloads. Ensure proper load balancing and implement reactive scaling strategies, such as reactive caching and reactive circuit breakers, to handle high traffic and improve system performance.
9. Best Practices for Building Reactive Applications
9.1 Choosing the Right Reactive Libraries
Select the reactive libraries that best suit your project’s requirements. Consider factors like community support, maturity, performance, and compatibility with other libraries and frameworks.
9.2 Designing for Resilience and Fault Tolerance
Design your reactive applications with resilience in mind. Use techniques like bulkheading, circuit breakers, and timeouts to handle failures gracefully and prevent cascading failures.
9.3 Monitoring and Debugging Reactive Systems
Implement proper monitoring and logging in your reactive applications to gain insights into system behavior. Use tools like Spring Sleuth, Micrometer, and distributed tracing solutions to track requests and identify performance bottlenecks.
Conclusion
Building reactive applications with Kotlin and Spring Boot empowers you to develop highly performant, scalable, and responsive systems. By leveraging Kotlin’s conciseness and Spring Boot’s robust ecosystem, you can handle large volumes of data and high-concurrency scenarios effectively. With the knowledge gained from this blog, you’re ready to embark on your journey to build reactive applications and harness the power of asynchronous and non-blocking programming.
Remember to stay updated with the latest trends and best practices in reactive programming, as the field continues to evolve. Happy coding!
Table of Contents
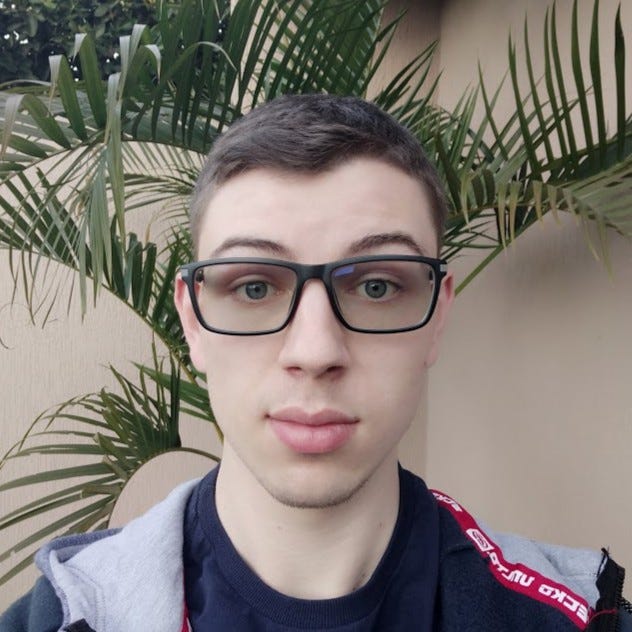
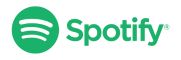