Kotlin and Room Persistence Library: Efficient Data Storage on Android
In the world of mobile app development, efficient data storage is a crucial aspect of creating a smooth and responsive user experience. Android developers often face the challenge of managing complex data structures while ensuring optimal performance. Kotlin, the modern and concise programming language for Android development, has made significant strides in simplifying Android app development. Paired with the Room Persistence Library, Kotlin empowers developers to build robust and efficient data storage solutions effortlessly. In this blog post, we will explore how Kotlin and Room work together harmoniously to handle data storage efficiently on Android.
1. Introduction to Kotlin and Room Persistence Library
1.1 Kotlin – A Brief Overview
Kotlin, developed by JetBrains, is a statically-typed, modern programming language that runs on the Java Virtual Machine (JVM). Google officially announced Kotlin as a first-class language for Android app development in 2017, and since then, it has gained immense popularity among Android developers due to its conciseness, null safety, and rich standard library.
1.2 Room Persistence Library – Why Use It?
Room Persistence Library is an Android Architecture Component that provides an abstraction layer over SQLite to enable efficient and optimized data access. Room simplifies database operations by mapping database objects to Java/Kotlin objects, saving developers from the hassle of writing complex SQL queries. It offers several benefits, including compile-time validation of queries, support for LiveData for reactive data observing, and seamless integration with other Architecture Components.
2. Advantages of Using Kotlin for Android Development
Before diving into the specifics of Room, let’s briefly highlight some of the key advantages of using Kotlin for Android app development:
2.1 Conciseness and Readability
Kotlin’s concise syntax reduces boilerplate code significantly, making the codebase more readable and maintainable. Features like data classes, lambda expressions, and extension functions allow developers to achieve more with fewer lines of code.
kotlin // Data class example data class User(val id: Int, val name: String, val email: String)
2.2 Null Safety
One of the most significant pain points in Android development is dealing with null references. Kotlin’s type system includes nullable and non-nullable types, reducing the risk of NullPointerExceptions at compile-time.
kotlin // Nullable type val name: String? = null // Non-nullable type val age: Int = 25
2.3 Enhanced Java Interoperability
Kotlin is fully interoperable with Java, allowing developers to seamlessly use existing Java libraries and frameworks in Kotlin projects. Additionally, Kotlin’s extension functions can be utilized to enhance existing Java classes without modifying their source code.
kotlin // Kotlin extension function fun String.isEmailValid(): Boolean { // Email validation logic }
2.4 Coroutines for Asynchronous Programming
Kotlin Coroutines simplify asynchronous programming by providing a structured and sequential approach. Coroutines allow developers to write asynchronous code in a more linear fashion, reducing callback hell and improving code readability.
kotlin // Coroutine example viewModelScope.launch { val data = fetchDataFromApi() displayData(data) }
3. What is Room Persistence Library?
Room is an SQLite object mapping library that provides an easy and efficient way to work with SQLite databases on Android. It acts as a layer on top of SQLite, offering compile-time verification of SQL queries and easy-to-use APIs for database operations.
3.1 Components of Room
Room consists of three primary components:
- Entity: Represents a table within the database. Each entity class corresponds to a row in the table, and each property of the class represents a column.
kotlin @Entity(tableName = "users") data class User( @PrimaryKey val id: Int, val name: String, val age: Int )
- Database: Represents the database itself and acts as the main access point for the underlying data. It should be an abstract class extending RoomDatabase.
kotlin @Database(entities = [User::class], version = 1) abstract class AppDatabase : RoomDatabase() { abstract fun userDao(): UserDao }
- DAO (Data Access Object): Contains the methods for accessing the database. DAOs define the SQL queries and expose them as functions.
kotlin @Dao interface UserDao { @Query("SELECT * FROM users") fun getAllUsers(): List<User> @Insert fun insertUser(user: User) // Additional CRUD methods... }
4. Setting Up Kotlin and Room in Your Android Project
Integrating Kotlin and Room into your Android project is a straightforward process. Follow these steps to set up the environment:
4.1 Configure Gradle for Kotlin
First, make sure you have Kotlin configured in your project by adding the Kotlin Gradle plugin to your build.gradle file:
gradle plugins { id 'com.android.application' id 'kotlin-android' //... }
4.2 Add Room Dependencies
Next, add the necessary Room dependencies to your app’s build.gradle file:
gradle dependencies { def room_version = "2.4.0-alpha02" implementation "androidx.room:room-runtime:$room_version" kapt "androidx.room:room-compiler:$room_version" // For Kotlin coroutines support with Room implementation "androidx.room:room-ktx:$room_version" }
The room-runtime dependency contains the core Room components, while room-compiler is required for generating the necessary code at compile time. If you plan to use Kotlin coroutines with Room, also include the room-ktx dependency.
4.3 Enable Kapt for Annotation Processing
To enable annotation processing for Room, add the following to your gradle.properties file:
gradle android.useAndroidX=true android.enableJetifier=true kotlin.useOldKapt=true
By enabling Kapt, the Room annotations in your code will be processed during compilation.
4.4 Create the Database Class
Now, create your Room database by extending RoomDatabase and defining your entities and their corresponding DAOs.
kotlin @Database(entities = [User::class], version = 1) abstract class AppDatabase : RoomDatabase() { abstract fun userDao(): UserDao companion object { private const val DATABASE_NAME = "app_database" @Volatile private var INSTANCE: AppDatabase? = null fun getInstance(context: Context): AppDatabase { return INSTANCE ?: synchronized(this) { val instance = Room.databaseBuilder( context.applicationContext, AppDatabase::class.java, DATABASE_NAME ).build() INSTANCE = instance instance } } } }
In the above code, we create the AppDatabase class and provide an abstract method userDao() to access the UserDao. The getInstance() method ensures that we use a single instance of the database throughout the app’s lifecycle.
4.5 Initialize the Database
Finally, initialize the database instance in your Application class.
kotlin class MyApp : Application() { val appDatabase by lazy { AppDatabase.getInstance(this) } }
By using lazy, the database instance will be created only when it is accessed for the first time.
5. Defining Entities and Creating the Database
With Room set up in your Android project, you can now define entities and create your database. Let’s create a simple User entity and use it in our database.
5.1 Define the User Entity
kotlin @Entity(tableName = "users") data class User( @PrimaryKey(autoGenerate = true) val id: Long = 0, val name: String, val age: Int )
In this example, we define a User entity with three properties: id, name, and age. The @Entity annotation specifies the table name, and the @PrimaryKey annotation indicates the primary key for the table.
5.2 Create the UserDao
Next, create the UserDao interface with methods for database operations:
kotlin @Dao interface UserDao { @Insert suspend fun insertUser(user: User) @Update suspend fun updateUser(user: User) @Delete suspend fun deleteUser(user: User) @Query("SELECT * FROM users") fun getAllUsers(): LiveData<List<User>> @Query("SELECT * FROM users WHERE age >= :minAge") fun getUsersOlderThan(minAge: Int): LiveData<List<User>> }
The @Dao annotation identifies this interface as a DAO, and the methods are annotated with @Insert, @Update, @Delete, and @Query to specify the corresponding database operations.
5.3 Create the AppDatabase
Finally, define the AppDatabase class as mentioned in section 4.4.
Now that our database is set up, we can use it to perform CRUD (Create, Read, Update, Delete) operations with Room.
6. Performing CRUD Operations with Room
6.1 Inserting Data
To insert data into the database, use the insertUser() method defined in the UserDao.
kotlin class UserRepository(private val userDao: UserDao) { suspend fun insertUser(user: User) { userDao.insertUser(user) } } // Usage example viewModelScope.launch { val user = User(name = "John Doe", age = 30) userRepository.insertUser(user) }
The suspend keyword is used here because database operations should be performed asynchronously. We’ll use Kotlin coroutines for asynchronous tasks in Room.
6.2 Retrieving Data
To retrieve data from the database, use the getAllUsers() method defined in the UserDao.
kotlin class UserRepository(private val userDao: UserDao) { val allUsers: LiveData<List<User>> = userDao.getAllUsers() } // Usage example val userRepository = UserRepository(appDatabase.userDao()) userRepository.allUsers.observe(this, Observer { users -> // Update UI with the list of users })
By returning a LiveData object from the getAllUsers() method, Room ensures that any changes to the database are automatically observed, and the UI is updated accordingly.
6.3 Updating Data
Updating data with Room is straightforward using the updateUser() method defined in the UserDao.
kotlin class UserRepository(private val userDao: UserDao) { suspend fun updateUser(user: User) { userDao.updateUser(user) } } // Usage example viewModelScope.launch { val userToUpdate = userRepository.allUsers.value?.firstOrNull() userToUpdate?.let { it.name = "Updated Name" userRepository.updateUser(it) } }
6.4 Deleting Data
To delete data from the database, use the deleteUser() method defined in the UserDao.
kotlin class UserRepository(private val userDao: UserDao) { suspend fun deleteUser(user: User) { userDao.deleteUser(user) } } // Usage example viewModelScope.launch { val userToDelete = userRepository.allUsers.value?.firstOrNull() userToDelete?.let { userRepository.deleteUser(it) } }
7. Room Database Migrations
As your app evolves, you might need to modify the database schema. Room provides migration support to handle such changes seamlessly without losing data. Migrations are essential when you update your app and need to adjust the database schema accordingly. Without migrations, your app might crash when users try to update to the latest version.
7.1 Creating Migrations
To create a migration, define a Migration object that specifies the version change and the SQL commands to migrate the data.
kotlin val migration1to2 = object : Migration(1, 2) { override fun migrate(database: SupportSQLiteDatabase) { database.execSQL("ALTER TABLE users ADD COLUMN last_login TEXT") } }
In this example, we’re adding a new column last_login to the users table.
7.2 Applying Migrations
To apply migrations, add them to the AppDatabase configuration using the addMigrations() method.
kotlin @Database(entities = [User::class], version = 2) abstract class AppDatabase : RoomDatabase() { // ... companion object { // ... fun getInstance(context: Context): AppDatabase { return Room.databaseBuilder( context.applicationContext, AppDatabase::class.java, DATABASE_NAME ) .addMigrations(migration1to2) // Add migrations here .build() } } }
Now, when you update your app’s version in the @Database annotation, Room will automatically handle the migration for you.
8. Asynchronous Database Operations with Kotlin Coroutines
Room supports asynchronous database operations with Kotlin coroutines. Instead of using callbacks or other traditional methods for asynchronous tasks, you can use coroutines to make your code more sequential and readable.
8.1 DAO Methods with Coroutines
To make a DAO method asynchronous, add the suspend modifier to the method signature.
kotlin @Dao interface UserDao { @Query("SELECT * FROM users") suspend fun getAllUsers(): List<User> @Insert suspend fun insertUser(user: User) // Other suspend DAO methods... }
8.2 Using Coroutines in ViewModel
In the ViewModel, use the viewModelScope.launch builder to call the suspend methods.
kotlin class UserViewModel(private val userRepository: UserRepository) : ViewModel() { val allUsers: LiveData<List<User>> = userRepository.allUsers fun addUser(name: String, age: Int) { viewModelScope.launch { val newUser = User(name = name, age = age) userRepository.insertUser(newUser) } } // Other ViewModel methods... }
By utilizing coroutines, the code becomes more streamlined and easier to understand, reducing callback hell and enhancing maintainability.
9. LiveData and Room: Reactive Data Observing
LiveData is a part of Android’s Architecture Components and is designed to observe changes to data and react to those changes. Room integrates seamlessly with LiveData, allowing your app to react to changes in the database automatically.
9.1 LiveData in DAO
As shown in previous examples, Room supports returning LiveData objects from DAO methods.
kotlin @Dao interface UserDao { @Query("SELECT * FROM users") fun getAllUsers(): LiveData<List<User>> @Insert suspend fun insertUser(user: User) // Other DAO methods... }
By returning a LiveData<List<User>> from the getAllUsers() method, the UI can observe the list of users and automatically update when changes occur.
9.2 Observing LiveData in ViewModel
In the ViewModel, observe the LiveData returned by the DAO.
kotlin class UserViewModel(private val userRepository: UserRepository) : ViewModel() { val allUsers: LiveData<List<User>> = userRepository.allUsers // Other ViewModel methods... }
The ViewModel provides the LiveData to the UI, and any changes in the database will trigger updates to the UI automatically.
10. ProGuard and Room
If you are using ProGuard for code shrinking and obfuscation, you need to add appropriate rules to keep Room-related classes and annotations intact.
proguard # Room -keep class androidx.room.RoomDatabase -keep class * extends androidx.room.RoomDatabase -keepclassmembers class * extends androidx.room.RoomDatabase { public static <fields>; } # Room annotations -keep class androidx.room.Entity -keep class androidx.room.PrimaryKey -keep class androidx.room.TypeConverters -keepattributes *Annotation*
By adding these ProGuard rules, you ensure that Room’s functionality is preserved after code obfuscation.
Conclusion
In this blog post, we explored how Kotlin and Room Persistence Library join forces to provide an efficient and seamless data storage solution for Android apps. We discussed the advantages of using Kotlin for Android development, the benefits of Room Persistence Library, and the step-by-step process of setting up Kotlin and Room in an Android project.
With Room, you can handle complex database operations with ease, while Kotlin’s concise and expressive syntax simplifies the overall development process. By leveraging Kotlin coroutines and LiveData, you ensure that your app is both performant and responsive, even with asynchronous tasks.
Kotlin and Room are indeed a powerful combination, and they open up a world of possibilities for Android developers to create efficient, reliable, and delightful apps for their users. So, go ahead and experiment with Kotlin and Room to build data storage solutions that will take your Android app development to new heights!
Table of Contents
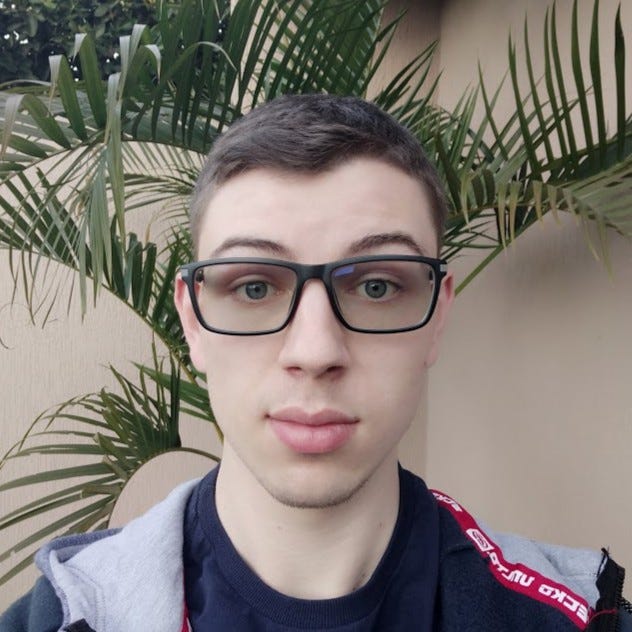
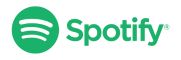