How to implement API rate limiting in Laravel?
Implementing API rate limiting in Laravel is like setting boundaries for your application’s interactions—it helps prevent abuse, ensures fair usage, and maintains system stability. Here’s how you can implement API rate limiting in Laravel in a user-friendly manner:
Understanding API Rate Limiting: API rate limiting is a technique used to control the number of requests made to an API within a specified time period. It helps prevent abuse, overloading, and denial-of-service attacks by restricting the rate at which clients can make requests to the API.
Using Laravel’s Rate Limiting Middleware: Laravel provides a built-in rate limiting middleware that makes it easy to implement rate limiting for your API routes. This middleware allows you to define rate limit rules based on the number of requests per minute, hour, or any other desired time interval.
Enabling Rate Limiting Middleware: To enable rate limiting for your API routes, you need to apply the rate limiting middleware to the routes or route groups that you want to limit. You can do this by adding the middleware to your route definitions in the routes/api.php file:
php Route::middleware('throttle:rate_limit,rate_limit_interval')->group(function () { // Define your API routes here });
Replace rate_limit with the maximum number of requests allowed within the specified time interval, and rate_limit_interval with the time interval in minutes, hours, or seconds.
Handling Rate Limit Exceeded Responses: When a client exceeds the rate limit, Laravel automatically responds with an HTTP status code 429 (Too Many Requests). You can customize the response message and headers by modifying the Illuminate\Http\Exceptions\ThrottleRequestsException class in your application.
Fine-tuning Rate Limiting Settings: Laravel allows you to fine-tune rate limiting settings and behavior according to your application’s requirements. You can customize the rate limit key, throttle middleware, storage driver, and other configuration options in the config/rate_limiting.php configuration file.
Testing Rate Limiting: It’s important to test your rate limiting implementation to ensure that it behaves as expected under different scenarios. Use tools like Postman or cURL to simulate requests and verify that rate limiting is applied correctly based on the defined rules.
Monitoring and Adjusting: Monitor your application’s usage patterns and adjust rate limiting settings as needed to maintain optimal performance and prevent abuse. Keep an eye on server logs, performance metrics, and user feedback to identify potential issues and make necessary adjustments.
By following these steps, you can effectively implement API rate limiting in your Laravel application, ensuring fair usage, preventing abuse, and maintaining system stability in a user-friendly manner.
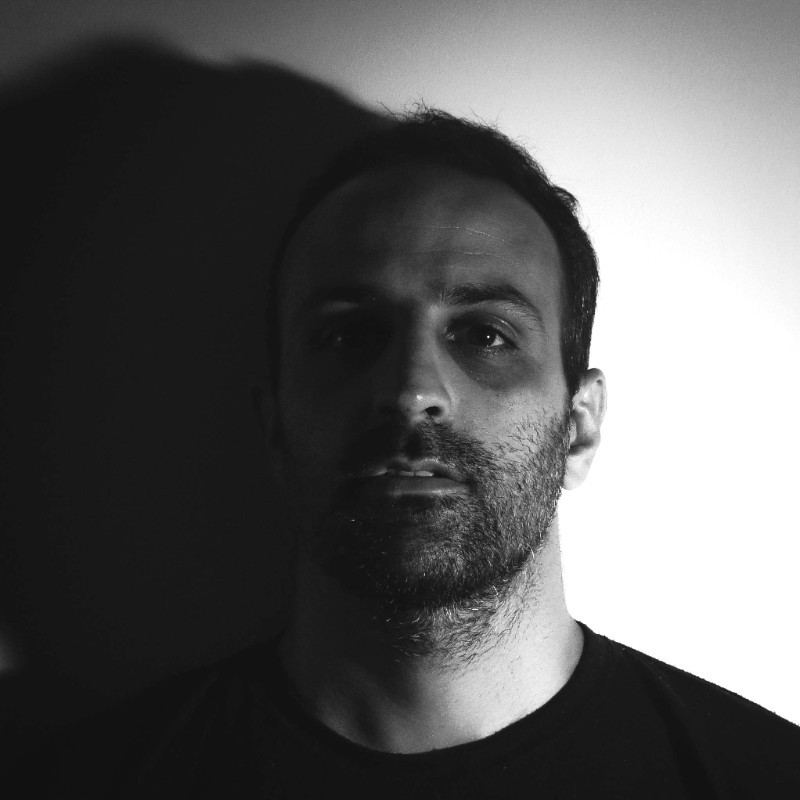
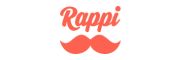