API Token-Based Authentication Made Easy with Laravel Sanctum
APIs are a cornerstone of modern web development, facilitating the seamless exchange of data between different systems. However, ensuring secure and controlled access to these resources is of paramount importance. Laravel, a popular PHP framework, offers Laravel Sanctum – a powerful tool for API token-based authentication.
Table of Contents
In this blog post, we will delve into the details of Laravel Sanctum. If the task seems overwhelming or beyond your technical expertise, remember you can always hire Laravel developers who can expertly secure your APIs using API tokens. With their assistance, you’ll be able to focus on your core business while they handle the complexities of Laravel Sanctum, providing real-world examples and explaining how to secure your APIs using API tokens.
1. What is Laravel Sanctum?
Laravel Sanctum is a Laravel-native authentication and authorization package that was designed with SPA (Single Page Application), mobile applications, and simple, token-based APIs in mind. Its primary goal is to provide a featherweight, unobtrusive authentication system to ensure your API endpoints are secure, but it’s flexible enough to handle more complex situations if required.
Sanctum offers multiple ways of handling authentication, including stateful sessions for traditional web apps and API tokens for decoupled or third-party systems. In this post, we’ll be focusing on the latter.
2. Getting Started with Laravel Sanctum
Before you can use Sanctum, you’ll need to have a Laravel project up and running. If you haven’t done this yet, the Laravel documentation provides a great starting point.
To install Sanctum, open a terminal window and navigate to your Laravel project directory. Then run the following command to add Sanctum to your project:
```bash composer require laravel/sanctum ```
Next, publish the Sanctum configuration and migration files using the vendor:publish Artisan command:
```bash php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider" ```
Once the files have been published, run the migrations:
```bash php artisan migrate ```
This creates the necessary database tables that Sanctum uses to manage API tokens.
Don’t forget to add the `HasApiTokens` trait to your user model:
```php <?php namespace App\Models; use Illuminate\Foundation\Auth\User as Authenticatable; use Laravel\Sanctum\HasApiTokens; class User extends Authenticatable { use HasApiTokens, Notifiable; } ```
This trait provides several methods that we’ll use to manage our API tokens.
3. Using API Tokens
Let’s start with the creation of API tokens. These tokens serve as the “password” that allows other systems to access your API. Here’s how you can create a token:
```php $user = User::find(1); $token = $user->createToken('token-name')->plainTextToken; ```
In the above code, we’re fetching a user (in this case, the user with ID 1), and creating a new API token named ‘token-name’. The `createToken` method returns a `Laravel\Sanctum\NewAccessToken` instance, and we’re using the `plainTextToken` property to get the actual token string.
Once a token is generated, it should be passed in the `Authorization` header in the format `Bearer your-token` for each subsequent request.
Here’s an example of an HTTP request using the API token:
```bash curl -H "Authorization: Bearer your-token" http://your-laravel-site.com/api/endpoint ```
4. Validating API Tokens
When a request is made with an API token, Laravel Sanctum validates the token and authenticates the user associated with it. This is done using the `auth:sanctum` middleware. Here’s an example of how you can use this middleware on a route:
```php Route::middleware('auth:sanctum')->get('/user', function (Request $request) { return $request->user(); }); ```
In this example, the `/user` route is protected by the `auth:sanctum` middleware. When a request is made to this route, Laravel Sanctum will authenticate the user based on the API token in the `Authorization` header.
If the token is valid, the user associated with the token will be authenticated, and the closure will return the user’s details. If the token is invalid, Laravel Sanctum will return a `401 Unauthorized` response.
5. Revoking API Tokens
It’s a good practice to allow your users to manage their API tokens. This includes the ability to revoke (or delete) their tokens when they’re no longer needed.
Here’s how you can revoke a token:
```php $user = User::find(1); $user->tokens()->where('name', 'token-name')->delete(); ```
In the above code, we’re fetching a user and then deleting the token named ‘token-name’ associated with that user.
Alternatively, you can delete all tokens for a user with the following command:
```php $user = User::find(1); $user->tokens()->delete(); ```
This is useful when you want to force a user to log out of all sessions (for example, in the case of a suspected account compromise).
Wrapping Up
In this post, we’ve navigated the complexities of Laravel Sanctum, offering insights into securing your APIs using API tokens. The steps to install Sanctum, generate and employ API tokens, validate tokens with the `auth:sanctum` middleware, and revoke tokens when necessary, have all been extensively covered.
If you’re looking to leverage these advanced features but are unsure of where to start, hiring Laravel developers can provide the expertise you need to optimize your applications.
Remember, Laravel Sanctum, though a potent package, is just a component of the broader Laravel ecosystem. It’s constructed to work seamlessly with other Laravel functionalities and packages, opening a world of opportunities. Don’t hesitate to discover what else Laravel can offer. Happy coding!
Table of Contents
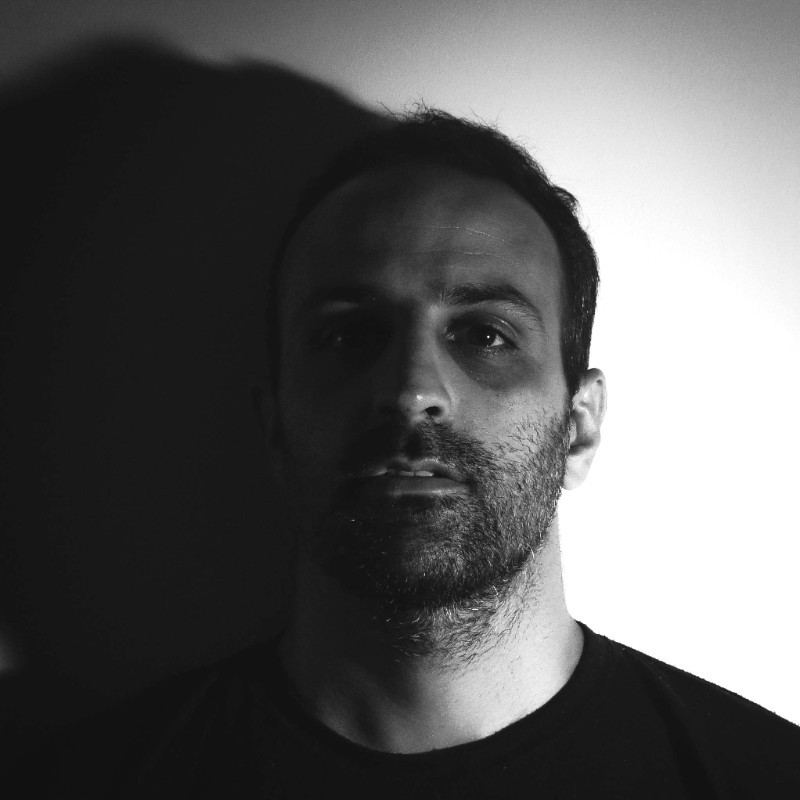
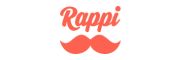