Laravel Blade Templating: Creating Dynamic Views with Ease
As we plunge into the world of web development, one thing becomes evident: the code we write is more than a series of commands. It’s a way of bringing ideas to life, expressing business logic in a language that machines can understand. It’s about making a user’s online journey as enjoyable and intuitive as possible. This is why many companies opt to hire Laravel developers who can leverage tools like the Blade templating engine, a game-changer in Laravel, and a robust PHP framework. These skilled professionals utilize Blade to simplify and enhance the coding process, leading to improved user experiences and more efficient web development.
Understanding Blade Templating Engine
Laravel’s Blade templating engine offers a way to write dynamic HTML content. This process helps to separate an application’s logic from its presentation, enhancing overall readability and maintainability. With Blade, developers can effortlessly create reusable templates, inherit and extend views, and write cleaner code, thereby increasing efficiency.
Setting Up a Blade View
Creating a Blade view is a straightforward task, even for those just starting to explore Laravel. In your Laravel application, navigate to the `resources/views` directory. This is where you, or the Laravel developers you hire, can create new files with the `.blade.php` extension. It’s a simple, efficient process that epitomizes the ease of working with Laravel. For instance, if you’re making a blog, you can easily create a view named `blog.blade.php`.
Displaying Data
Blade allows you to display data from your PHP variables easily. You can display data using the double curly braces `{}` syntax. For example:
```php Hello, {{ $name }}.
In this case, `$name` is a variable available in your view. Laravel’s Blade engine will replace `{{ $name }}` with the content of the variable. Note that Blade automatically escapes any HTML entities in the displayed data for security reasons, preventing XSS attacks.
Control Structures
Blade offers several useful control structures, such as conditional statements and loops, similar to PHP. The key difference is that Blade’s syntax is more intuitive and cleaner.
If Statements
Consider this Blade syntax for an `if` statement:
```php @if (condition) <!-- This code will run if the condition is true --> @endif
Loops
Blade also simplifies the use of loops. Here’s how you’d write a `foreach` loop:
```php @foreach ($users as $user) <p>This is user {{ $user->id }}</p> @endforeach
Template Inheritance
One of Blade’s most powerful features is template inheritance, which lets you create a base template with a layout that other views can inherit.
To define a layout in Blade, you use the `@section` and `@yield` directives.
– `@section`: This directive is used to define a section of content.
– `@yield`: This directive is used to display the contents of a given section.
Consider a simple layout named `app.blade.php`:
```php <!DOCTYPE html> <html> <head> <title>@yield('title')</title> </head> <body> @yield('content') </body> </html>
To extend this layout, you’d use the `@extends` directive:
```php @extends('app') @section('title', 'Page Title') @section('content') <p>This is my body content.</p> @endsection
Blade Components
With Laravel 7, Blade components have seen an upgrade. Components are reusable parts of a webpage, like headers, footers, or any reusable HTML layout. They also provide a clean, powerful way to create custom tags with pure PHP.
To create a component, use the `make:component` Artisan command:
```bash php artisan make:component Alert
This command will create a class and view file for the component.
Blade Directives
Blade offers numerous custom directives to ease your development process, such as:
– `@json`: The `@json` directive converts an array into a JSON string.
– `@csrf`: The `@csrf` directive generates a CSRF token field inside forms.
– `@method`: The `@method` directive generates a method field for non-GET requests.
And many more!
Conclusion
Laravel’s Blade templating engine is a powerful tool that helps developers, especially those who hire Laravel developers, to write clean, efficient, and secure code with ease. It simplifies many tasks such as displaying data, writing control structures, and template inheritance. Blade’s elegance and simplicity make Laravel a highly preferred PHP framework among developers, prompting many companies to hire Laravel developers for their projects. Once you start using Blade, either by mastering it yourself or by leveraging the expertise of hired Laravel developers, you will appreciate how it helps to streamline the development process and improve your application’s structure.
Table of Contents
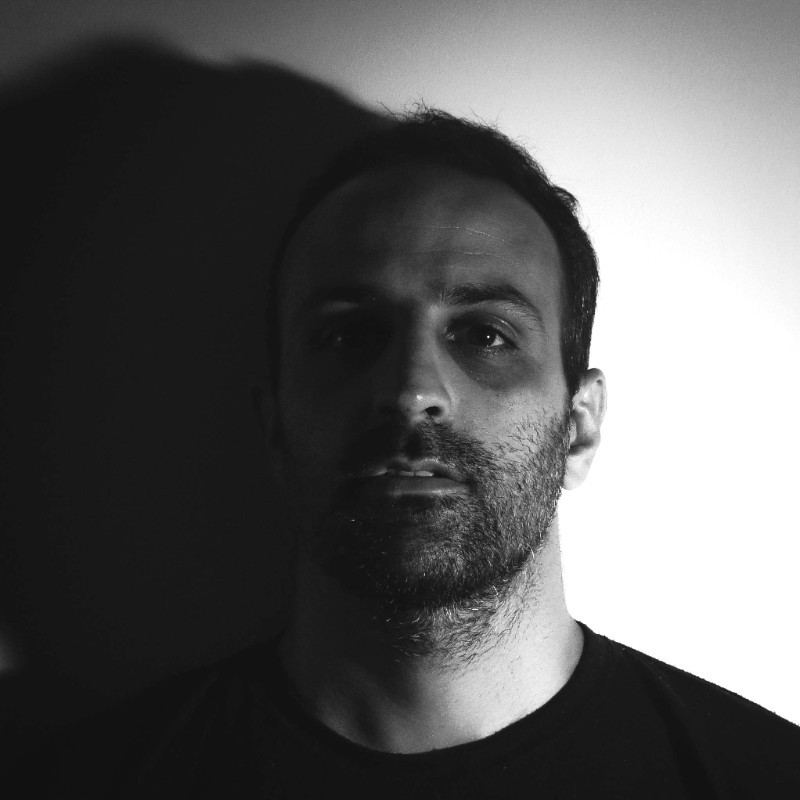
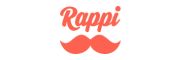