Instant Updates, Lightning Speed: A Laravel Broadcasting & Socket.io Primer
The web is evolving rapidly, with more applications requiring real-time data updates than ever before. Whether it’s a chat application, a live statistics dashboard, or even a real-time notification system, the need to push data to the client without them asking is increasing. Enter Laravel Broadcasting coupled with Socket.io, a match made in developer heaven for creating real-time applications.
1. What is Laravel Broadcasting?
Laravel Broadcasting provides an expressive API to broadcast your application’s events over a web socket connection, making it easy to push real-time data to your client applications. It abstracts much of the complexity of setting up a real-time broadcasting solution, allowing developers to focus on the core logic.
2. Socket.io
Socket.io is a JavaScript library that enables real-time, bi-directional communication between web clients and servers. It works on every platform, browser, or device, focusing equally on reliability and speed.
3. Setting up Laravel Broadcasting with Socket.io
Before delving into examples, let’s get our environment set up.
- Install Laravel: If you haven’t, install Laravel using Composer:
```bash composer create-project --prefer-dist laravel/laravel real-time-app ```
- Install necessary Node.js packages:
Navigate to your Laravel project and install the required packages.
```bash npm install socket.io-client socket.io redis ioredis express --save ```
- Configure Laravel Broadcasting:
– Update `.env` to use Redis as the BROADCAST_DRIVER.
``` BROADCAST_DRIVER=redis ```
– Uncomment the `App\Providers\BroadcastServiceProvider` in the `config/app.php`.
4. Creating a Simple Chat Application
To demonstrate, we’ll build a rudimentary chat application.
4.1. Backend Setup:
– First, create a chat event:
```bash php artisan make:event MessageSent ```
– In the `MessageSent` event, implement the `ShouldBroadcast` interface. This tells Laravel to broadcast this event.
```php class MessageSent implements ShouldBroadcast { public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat'); } } ```
– Next, set up a route and controller to trigger this event:
```bash php artisan make:controller ChatController ```
And in the controller:
```php public function sendMessage(Request $request) { $message = $request->input('message'); event(new MessageSent($message)); return ['status' => 'OK']; } ```
4.2. Frontend Setup:
– Set up Socket.io to listen for our events.
```javascript let socket = io('http://localhost:3000'); socket.on('chat:MessageSent', function(data) { console.log(data); }); ```
4.3. Socket.io Server Setup:
Create a `server.js` file in your root directory:
```javascript const io = require('socket.io')(6001); const Redis = require('ioredis'); const redis = new Redis(); redis.subscribe('chat'); redis.on('message', function(channel, message) { message = JSON.parse(message); io.emit(channel + ':' + message.event, message.data); }); ```
Start the Socket.io server:
```bash node server.js ```
5. Testing the Application
- Start your Laravel application.
- Trigger a message by hitting the endpoint or using a form on the frontend.
- Watch as the Socket.io client receives and logs the message in real-time.
Conclusion
Laravel Broadcasting and Socket.io make a powerful duo for building real-time applications. This blog post touched upon the basics, but the potential applications are vast, ranging from real-time dashboards, live sports updates, online multiplayer games, collaborative tools, and much more.
By integrating these two technologies, developers can unlock a world of interactive and dynamic user experiences that were previously cumbersome to implement. So, the next time you think of building a real-time application, consider giving Laravel Broadcasting and Socket.io a shot!
Table of Contents
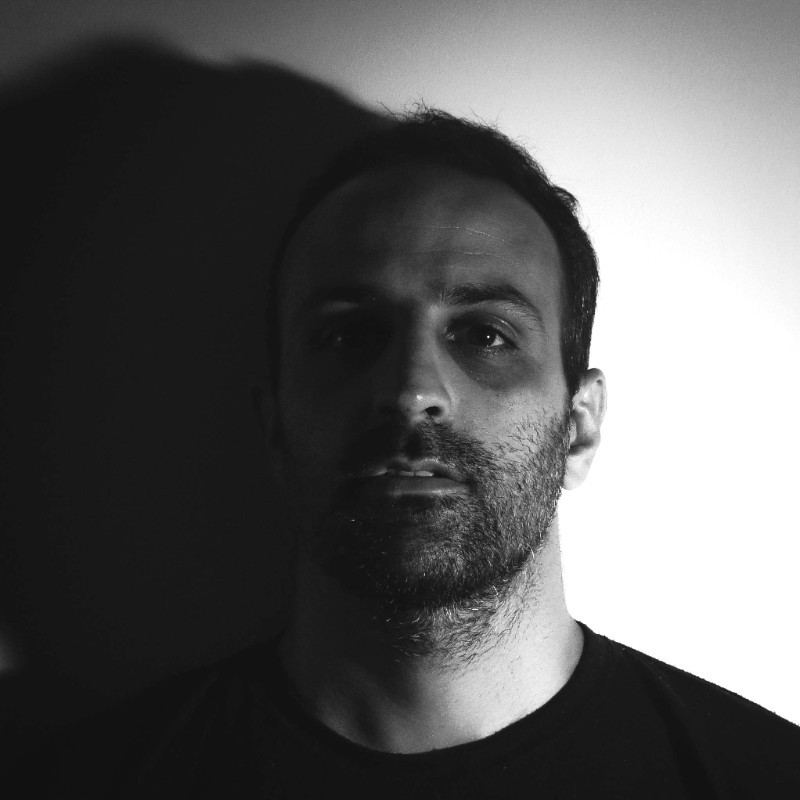
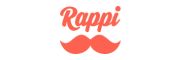