Revolutionize Laravel Response Times with Redis Caching
Caching is one of the most effective ways to improve the performance and responsiveness of a web application. Laravel, one of the most popular PHP frameworks, has native support for a variety of caching backends, including file-based caching, database caching, and in-memory caching solutions like Redis. In this article, we’ll explore how to harness the power of Redis in a Laravel application to achieve significant speed enhancements.
1. Why Redis?
Redis, short for Remote Dictionary Server, is an in-memory data structure store that can be used for various purposes, including caching. Some advantages of using Redis as a caching backend include:
- Speed: As an in-memory data store, Redis offers rapid data access.
- Persistence: Unlike other in-memory stores, Redis offers options to persist data.
- Scalability: Redis can be easily scaled out with replication and partitioning.
2. Setting Up Redis with Laravel
Before diving into the code, ensure that you have Redis installed and running. Once that’s set up, you can proceed with integrating Redis into your Laravel application:
- Install the Redis package: Use composer to install Laravel’s Redis package.
```bash composer require predis/predis ```
- Update `.env` file: Modify the `.env` file in your Laravel application to specify Redis as your cache and session driver.
``` CACHE_DRIVER=redis SESSION_DRIVER=redis ```
- Configuring Redis: In `config/database.php`, ensure the Redis configurations are set up correctly.
3. Caching with Redis in Laravel
- Storing Items in Cache
```php use Illuminate\Support\Facades\Cache; // Store an item in the cache for 10 minutes Cache::put('key', 'value', 600); ```
- Retrieving Items from Cache
```php $value = Cache::get('key'); ```
With a default value if the key doesn’t exist:
```php $value = Cache::get('key', 'default'); ```
- Checking for an Item in Cache
```php if (Cache::has('key')) { // Key exists in cache } ```
- Removing Items from Cache
```php Cache::forget('key'); ```
4. Examples of Using Redis Caching in Laravel
- Caching Database Queries:
In many applications, database queries are the primary bottleneck. By caching the results of these queries, you can drastically reduce the load on your database and increase your application’s speed.
Here’s an example of caching a database query result:
```php use Illuminate\Support\Facades\Cache; $users = Cache::remember('all-users', 600, function () { return DB::table('users')->get(); }); ```
In the above example, if the key `all-users` doesn’t exist in the cache, the query will execute, and its result will be stored in the cache for 10 minutes. If the key does exist, the cached result is returned instead.
- Caching API Calls:
API calls, especially to external services, can be time-consuming. Caching the results can be highly beneficial.
```php $data = Cache::remember('api-response', 3600, function() { return Http::get('https://api.example.com/data'); }); ```
- Caching Configurations or Settings:
For applications with dynamic settings stored in a database, consider caching them for quicker access:
```php $settings = Cache::rememberForever('site-settings', function () { return DB::table('settings')->pluck('value', 'key'); }); ```
5. Cache Tagging with Redis
Cache tags are a feature in Laravel that allow you to tag related items in the cache and then clear all cached items with a given tag. This is useful when you want to clear a subset of the cache.
Example:
```php // Storing tagged cache Cache::tags(['people', 'authors'])->put('John', $john, 3600); Cache::tags(['people', 'authors'])->put('Jane', $jane, 3600); // Clearing all cache data tagged with 'authors' Cache::tags('authors')->flush(); ```
Conclusion
Integrating Redis with Laravel for caching purposes is a practical step towards optimizing your application for speed and performance. Whether you’re caching database results, API responses, or configuration settings, Redis offers a robust and efficient solution. Remember, while caching is powerful, always ensure the integrity and relevance of your data by invalidating and refreshing your cache when necessary.
Table of Contents
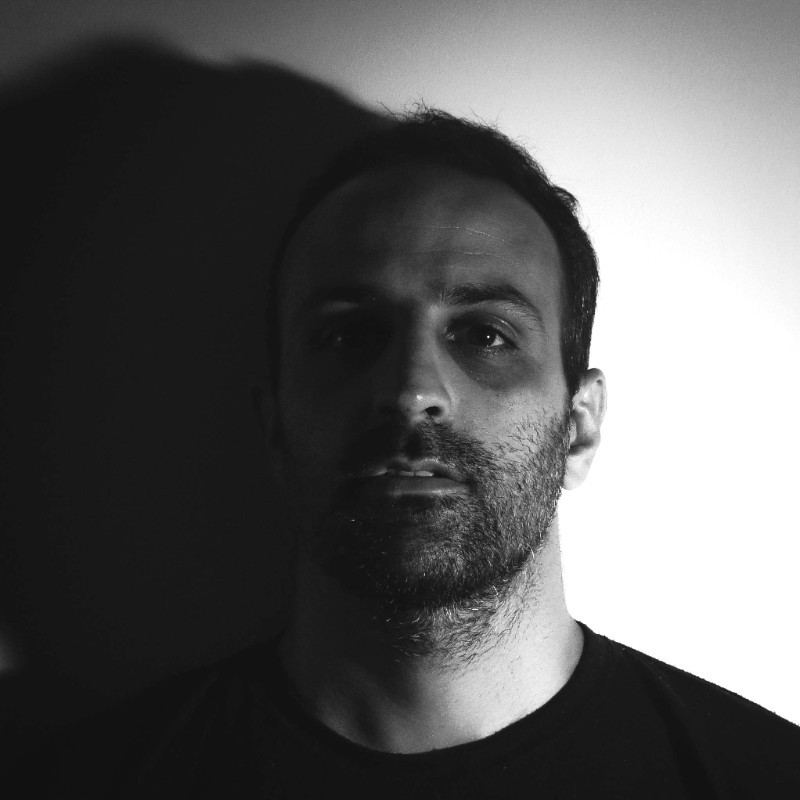
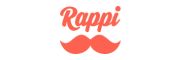