The Definitive Guide to Laravel Cashier Paddle for Subscription Management
Managing subscriptions in a web application can be a daunting task, especially if you’re on the lookout to hire Laravel developers for your project. The process involves handling recurring billing, securely storing credit card details, prorating charges, and more. Laravel Cashier, an official Laravel package, simplifies these tasks, making handling subscription billing a breeze.
Table of Contents
This eases the workload for both seasoned Laravel developers and those looking to hire Laravel developers. Today, we’ll focus on Laravel Cashier’s integration with Paddle, a popular payment and subscription platform.
1. Introduction to Laravel Cashier
Laravel Cashier is a tool that provides an expressive, fluent interface to Paddle’s subscription billing services. It handles almost all of the boilerplate subscription billing code you dread writing. With Laravel Cashier, developers can focus on creating the rest of their application without worrying about the details of handling subscription billing.
2. Introduction to Paddle
Paddle is a subscription and billing management platform that unifies payments, subscriptions, and invoicing into one platform. It is popular for its ability to handle global taxes and its built-in compliance features. With Paddle, you can sell your products worldwide without the hassle of dealing with tax regulations.
3. Setting Up Laravel Cashier Paddle
Before diving in, you need to have a Laravel project set up. You also need to have an account with Paddle and your vendor ID and authentication details ready.
Install Laravel Cashier Paddle through Composer:
```bash composer require laravel/cashier-paddle ```
Next, you need to publish the Cashier migration and migrate it:
```bash php artisan cashier:install php artisan migrate ```
You also need to add your Paddle API details to your `.env` file:
```bash PADDLE_VENDOR_ID=your-paddle-vendor-id PADDLE_VENDOR_AUTH_CODE=your-paddle-vendor-auth-code ```
Finally, in your `Billable` model, usually the `User` model, use the `Billable` trait:
```php use Laravel\Paddle\Billable; class User extends Authenticatable { use Billable; } ```
4. Creating Subscriptions
With Laravel Cashier Paddle, you can create subscriptions easily. Suppose we have a form where users can select a subscription plan. Once the form is submitted, you can create the subscription like this:
```php $user = Auth::user(); $subscription = $user->newSubscription('main', $planId)->create($checkoutId); ```
In the example above, `main` is the name of the subscription, `$planId` is the ID of the subscription plan in Paddle, and `$checkoutId` is the checkout ID from the Paddle checkout process.
5. Handling Webhooks
Paddle communicates with your application through webhooks. Laravel Cashier automatically handles Paddle’s webhooks via its included controller. All you need to do is point a route to this controller.
```php Route::post( 'webhook-url', '\Laravel\Paddle\Http\Controllers\WebhookController@handleWebhook' ); ```
Replace `’webhook-url’` with the URL Paddle will use to send webhook notifications to your application. Be sure to configure this route in your Paddle dashboard as well.
6. Checking Subscription Status
Laravel Cashier provides several methods to check the status of a user’s subscription:
```php if ($user->subscribed('main')) { // User is subscribed to the "main" subscription } if ($user->subscribedToPlan($planId, 'main')) { // User is subscribed to "main" and on plan $planId } if ($user->subscription('main')->active()) { // User subscription is active } if ($user->subscription('main')->paused()) { // User subscription is paused } if ($user->subscription('main')->pastDue()) { // User subscription has past due payment } if ($user->subscription('main')->canceled()) { // User subscription is canceled } ```
7. Pausing and Resuming Subscriptions
To pause a subscription:
```php $user->subscription('main')->pause(); ```
And to resume a paused subscription:
```php $user->subscription('main')->resume(); ```
8. Canceling Subscriptions
Canceling a subscription is also straightforward:
```php $user->subscription('main')->cancel(); ```
By default, the subscription will remain active until the end of the billing period. You can immediately cancel the subscription with:
```php $user->subscription('main')->cancelNow(); ```
Conclusion
Laravel Cashier Paddle provides a clean, simple API for handling subscription billing with Paddle. It abstracts various aspects of subscription management, allowing developers, including those looking to hire Laravel developers, to focus more on the core features of their applications.
Whether you’re a seasoned Laravel developer or a business seeking to hire Laravel developers, this guide should provide a better understanding of how to effectively use Laravel Cashier with Paddle. Embrace this tool and simplify your subscription management tasks. Happy coding!
Table of Contents
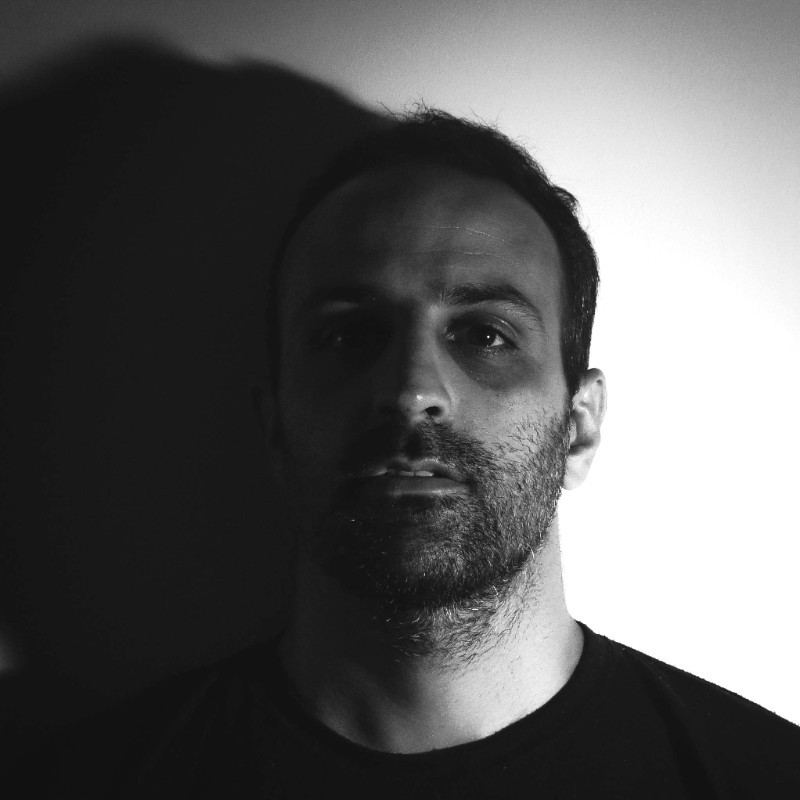
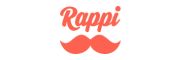