Conquer Cron Jobs with Laravel Scheduler: An In-Depth Guide
Laravel, a PHP framework revered for its elegant syntax, is frequently utilized by professional Laravel developers to handle complex tasks. Among its features, the Laravel Scheduler is a powerful tool that simplifies the process of handling scheduled tasks. Traditionally, tasks in a Linux/UNIX environment were scheduled using cron jobs.
Table of Contents
While effective, cron jobs can become quite complex to manage, especially as your application grows in size and complexity. This is where hiring Laravel developers can be of significant advantage. The Laravel Scheduler, which they master, provides an abstraction layer on top of these cron jobs, making the entire process easier to manage and maintain, even for large-scale applications.
1. The Basics of Laravel Scheduler
The Laravel Scheduler is included out of the box in Laravel and allows developers to schedule tasks in an intuitive and user-friendly way. This scheduling system allows you to fluently and expressively define your command schedule within Laravel itself, and only a single cron entry is needed on your server. The Laravel command scheduler allows you to fluently and expressively define your command schedule within Laravel itself.
1.1. Setting Up The Scheduler
The first step in using Laravel Scheduler is to add the following cron entry to your server. This command schedules the Laravel command scheduler to run every minute:
``` * * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1 ```
Here, `/path-to-your-project` should be the absolute path to the Laravel project on your server. This cron entry checks every minute if there are any scheduled tasks to run.
1.2. Scheduling Tasks
To schedule tasks, you must write the tasks in the `schedule` method of the `App\Console\Kernel` class. Laravel includes multiple options to schedule your tasks, such as:
– `->cron(‘…’)`: define the cron expression yourself
– `->everyMinute()`: run the task every minute
– `->hourly()`: run the task every hour
– `->daily()`: run the task once a day at midnight
– `->weekly()`: run the task once a week
– `->monthly()`: run the task once a month
Examples of Using Laravel Scheduler
Let’s delve into some practical examples to understand the usage of Laravel Scheduler more clearly.
Example 1: Send Weekly Email Reports
Imagine you want to send weekly email reports to your users. To achieve this, you could create a Laravel command `SendEmailReports`:
```php php artisan make:command SendEmailReports ```
This command creates a new command class in the `app/Console/Commands` directory. In the `handle` method, you can define the logic to send the emails:
```php public function handle() { $users = User::all(); foreach ($users as $user) { Mail::to($user->email)->send(new WeeklyReport); } } ```
To schedule this command to run weekly, you can add the following code to the `schedule` method of the `App\Console\Kernel` class:
```php protected function schedule(Schedule $schedule) { $schedule->command('email:send-reports')->weekly(); } ```
Example 2: Clear Cache Daily
If you want to clear your application cache every day at midnight, you can use the `schedule` method like this:
```php protected function schedule(Schedule $schedule) { $schedule->command('cache:clear')->daily(); } ```
Example 3: Complex Scheduling
For more complex scenarios, you can combine different methods. For example, if you want to run a command every day at 3:30 PM, you can use the `dailyAt` method:
```php protected function schedule(Schedule $schedule) { $schedule->command('emails:send')->dailyAt('15:30'); } ```
You can also use the `twiceDaily` method to run a task twice a day at specific hours:
```php protected function schedule(Schedule $schedule) { $schedule->command('backup:run')->twiceDaily(1, 13); } ```
Here, the `backup:run` command will run at 1 AM and 1 PM.
Example 4: Conditional Scheduling
In some situations, you may want to run tasks only on specific days of the week. For example, you may want to run a task every hour, but only on weekdays. You can achieve this with the `weekdays` method:
```php protected function schedule(Schedule $schedule) { $schedule->command('emails:send')->hourly()->weekdays(); } ```
Example 5: Preventing Task Overlaps
By default, scheduled tasks will run even if the previous instance of the task is still running. If a task should not be started if it’s already running, you can use the `withoutOverlapping` method:
```php protected function schedule(Schedule $schedule) { $schedule->command('emails:send')->hourly()->withoutOverlapping(); } ```
Conclusion
Laravel’s task scheduler is an incredible tool, mastered by professional Laravel developers, that provides an intuitive and straightforward way to manage scheduled tasks in a Laravel application. It brings the power of task scheduling right into your Laravel application, without the need to manually manage numerous cron job entries.
Furthermore, it allows for handling complex scheduling scenarios with relative ease. With the Laravel Scheduler, developers can focus on the core features of the application, knowing that the scheduling of tasks is well taken care of. If your project requires this level of expertise, consider the option to hire Laravel developers who are proficient in effectively utilizing such powerful tools.
Table of Contents
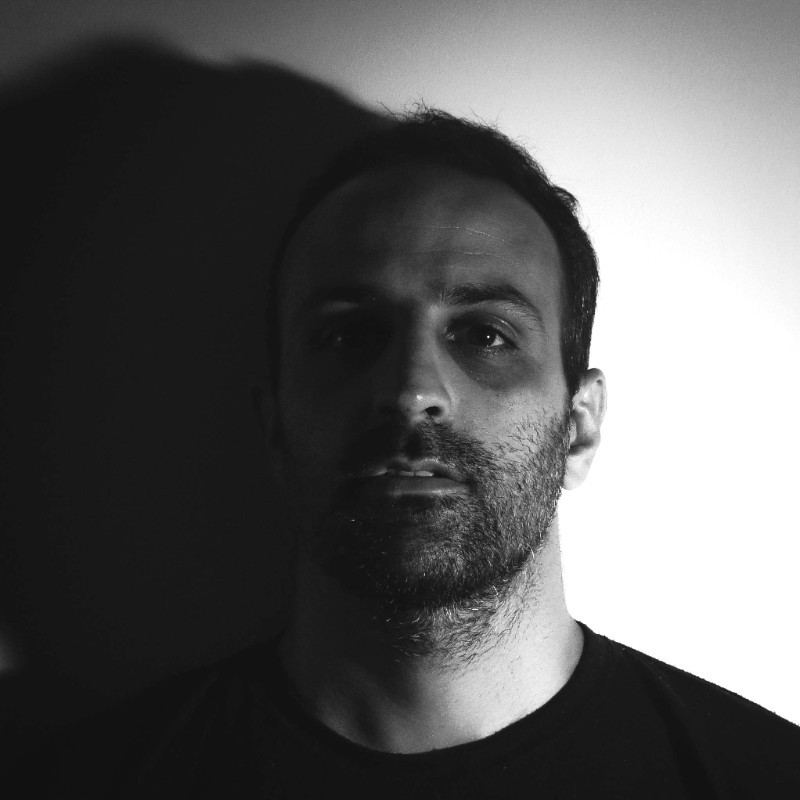
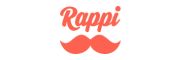