Laravel Caching: Boost Performance with Data Caching Techniques
When it comes to creating high-performance web applications, efficient data management is a critical factor that can drastically improve your application’s response time. Laravel, an elegant PHP framework for web artisans, provides a simple and expressive syntax that eases many common tasks in web development, including caching. This is why many businesses choose to hire Laravel developers who understand how to implement such features effectively.
This blog post will dive into Laravel caching, explaining what it is, why it’s important, and how you can leverage various data caching techniques to supercharge your Laravel application performance. Whether you’re a seasoned Laravel developer or looking to hire Laravel developers for your project, understanding these techniques can help you unlock your application’s full potential.
Understanding Caching
Caching, in its most simple form, is the process of storing data temporarily in high-speed storage areas to serve future requests more quickly. In web applications, caching reduces the need to access the database directly every time a client makes a request, thus significantly cutting down on response times.
Why is Caching Important in Laravel?
Laravel applications, like any other web applications, often need to perform costly database operations or intensive calculations, especially when dealing with large data sets. This is why businesses often opt to hire Laravel developers, as they are proficient in implementing advanced features such as caching. Performing these operations every time a client makes a request can negatively impact the performance and user experience. Caching comes in handy to resolve this by storing the results of such expensive operations and serving them on subsequent requests. With experienced Laravel developers, you can ensure that these caching techniques are utilized correctly to optimize your application’s performance.
Laravel Caching Techniques
Let’s delve into some of the common techniques you can employ to cache data in your Laravel application.
1. Cache Configuration
Before getting into the specifics of caching in Laravel, it’s essential to understand how to configure caching in your application. Laravel provides a central location for all caching configuration within the `config/cache.php` file. Here, you can define your cache “store,” which determines where the cache data will be stored – database, file, Memcached, Redis, or even in-memory using an array.
2. Caching Views
Caching views in Laravel involves storing the HTML generated by your views, reducing the need to compile the views every time a request is made. To cache views, Laravel provides the `view:cache` Artisan command. Running `php artisan view:cache` will compile all your blade views into plain PHP code, significantly reducing the rendering time on subsequent requests.
3. Route Caching
Laravel’s `route:cache` command is an excellent way to improve the performance of your routes. This command compiles all of your application’s routes into a single PHP file, significantly reducing the framework’s amount of work when handling a request. However, remember that any changes to your application’s routes while route caching is in effect will not be recognized until you refresh or clear the route cache.
4. Model Caching
When it comes to intensive database operations, model caching can be a game-changer. Laravel does not provide out-of-the-box support for model caching, but you can use packages like ‘laravel-model-caching’ to achieve this. Model caching involves storing the results of a database query and serving the cached result on subsequent requests, significantly reducing database interactions.
5. Full-Page Caching
For applications with mostly read operations and less write/update operations, full-page caching can boost performance drastically. Full-page caching involves storing the entire HTML of a page response and serving it directly from the cache for subsequent requests. Laravel does not have built-in support for this, but you can achieve it by custom middleware or using packages like ‘responsecache.’
6. Using Cache Drivers
Laravel supports various cache drivers, including File, Database, APC, Memcached, Array, and Redis. You can choose a cache driver that best suits your application’s needs. For applications with heavy caching needs, in-memory stores like Memcached or Redis are recommended, as they provide quicker read/write speeds compared to file or database caching.
7. Query Caching
Query caching is a simple yet effective way to optimize the performance of your Laravel application. This technique involves storing the results of a database query into the cache. When the same query is executed again, Laravel will fetch the result from the cache instead of hitting the database, thereby saving execution time.
Here’s a simple example of query caching in Laravel:
```php $users = Cache::remember('users', 600, function () { return DB::table('users')->get(); });
In this example, the `remember` method is used to retrieve the data from the cache if it exists; otherwise, the closure is executed, the result is stored in the cache, and then returned. The number 600 is the amount of time in seconds that the data should be kept in the cache.
8. Object Caching
Laravel also supports object caching, which is incredibly useful when you have expensive-to-create objects or pieces of data. Instead of recreating these objects each time they’re needed, you can create them once, cache them, and then pull them from the cache when required.
Let’s consider an example:
```php $expensiveObject = Cache::remember('expensiveObject', 600, function () { return new ExpensiveObject(); });
In this code, an instance of `ExpensiveObject` is cached. So when you need to use this object, you can just pull it from the cache, which is much faster than creating a new instance.
Applying Caching in Laravel
Laravel provides an expressive, unified API for various caching systems. Here’s a basic example of setting and retrieving items from the cache:
```php use Illuminate\Support\Facades\Cache; // Storing Items in the Cache Cache::put('key', 'value', 600); // Retrieving Items from the Cache $value = Cache::get('key');
In the example above, `Cache::put` method is used to store an item in the cache for 600 seconds. And the `Cache::get` method is used to retrieve the item from the cache.
Laravel Cache also provides a helper `cache()` function, which you can use to get or set cache data:
```php // Store data for 600 seconds cache(['key' => 'value'], 600); // Retrieve data $value = cache('key');
Clearing the Cache
There may be situations where you need to clear the cache, such as during deployments or when debugging. Laravel provides various Artisan commands to accomplish this:
– `php artisan cache:clear` – This command clears the application cache.
– `php artisan route:clear` – This command clears the route cache.
– `php artisan view:clear` – This command clears the compiled view files.
Conclusion
By integrating caching into your Laravel applications, you can significantly improve your application’s performance, leading to better user experiences and more efficient resource utilization. This is where the expertise of Laravel developers becomes crucial. Companies often hire Laravel developers who can effectively utilize the out-of-the-box support Laravel provides or various packages to extend its functionality. By leveraging Laravel’s caching capabilities, these skilled developers can greatly enhance your application’s responsiveness and scalability.
Table of Contents
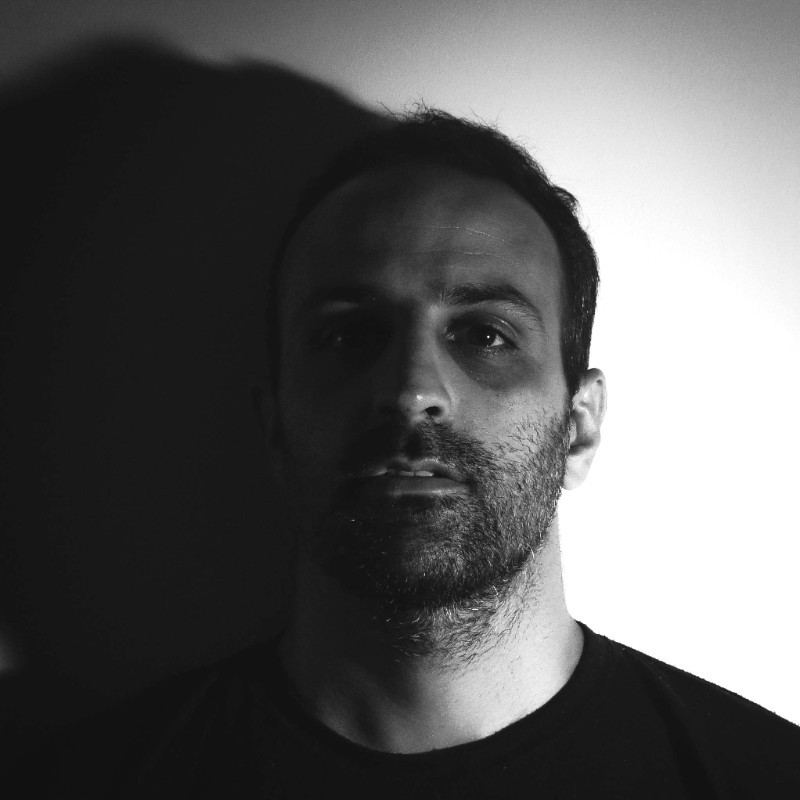
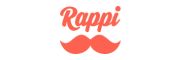