Laravel Dusk Browser Testing: Ensuring Quality in Your Laravel Applications
Laravel Dusk is an end-to-end browser testing tool designed specifically for the Laravel framework, enhancing the capabilities of Laravel developers. It provides an expressive and easy-to-use API that allows developers to test their applications like real users, performing actions such as clicking buttons, filling forms, and even handling JavaScript-driven behaviors. This level of intricate testing is often a compelling reason to hire Laravel developers. Now, let’s dive into the specifics of Laravel Dusk and explore how it empowers Laravel developers to build robust test suites.
Table of Contents
1. Getting Started with Laravel Dusk
Before we get into the meat of Laravel Dusk, it’s crucial to understand the prerequisites. Laravel Dusk requires a fresh Laravel installation of version 5.4 or higher. If you have an older version, consider updating your Laravel before proceeding.
To install Laravel Dusk, use the following composer command:
```bash composer require --dev laravel/dusk ```
After the installation, run the `dusk:install` Artisan command:
```bash php artisan dusk:install ```
This command will set up Dusk for your application and create a `Browser` directory under the `tests` directory. You can write all your browser tests in this directory.
2. Writing Your First Browser Test
Laravel Dusk makes writing browser tests feel like a breeze. Let’s write a simple browser test to validate the login functionality of a Laravel application.
In the `tests/Browser` directory, create a new test file called `LoginTest.php` and write the following code:
```php <?php namespace Tests\Browser; use Laravel\Dusk\Browser; use Tests\DuskTestCase; class LoginTest extends DuskTestCase { /** * A basic browser test example. * * @return void */ public function testBasicExample() { $this->browse(function (Browser $browser) { $browser->visit('/login') ->type('email', 'taylor@laravel.com') ->type('password', 'secret') ->press('Login') ->assertPathIs('/home'); }); } } ```
In this example, we’re defining a test called `testBasicExample`. This test uses the `browse` method to initiate a browser instance. The test visits the login page, types in the email and password, clicks the login button, and then verifies that the user is redirected to the home page.
3. Advanced Browser Interactions
3.1. Using the Dusk Selector
Laravel Dusk provides a `@` selector to select HTML elements on a page. This allows you to avoid using verbose selectors like CSS or XPath. To define a Dusk selector, add a `dusk` attribute to your HTML element:
```html <button dusk="login-button">Login</button> ```
You can then use the `@` selector in your Dusk tests like so:
```php $browser->press('@login-button'); ```
4. Handling JavaScript Modals
Laravel Dusk also provides methods to interact with JavaScript-driven modal windows. These include alert, confirm, and prompt boxes. The `acceptDialog` and `dismissDialog` methods can be used to interact with these modals. Here’s an example:
```php $browser->press('@delete-button') ->acceptDialog() ->assertSee('Record deleted successfully.'); ```
In this example, pressing the delete button triggers a confirmation dialog. The `acceptDialog` method accepts the dialog, and the test asserts that a success message is displayed.
5. Running Tests
To run your Dusk tests, use the `dusk` Artisan command:
```bash php artisan dusk ```
This command will execute all of your Dusk tests. If you want to only run a single test, you can pass the file path of the test as an argument:
```bash php artisan dusk tests/Browser/LoginTest.php ```
6. Building Robust Test Suites
A test suite is only as good as its coverage and reliability. Laravel Dusk provides various features to build robust and reliable test suites.
6.1. Page Objects
To reduce duplication and promote reusability in your tests, Laravel Dusk introduces the concept of Page Objects. Page objects allow you to define a class that corresponds to a specific page of your application, where you can define methods for the various actions a user can perform on that page.
For example, let’s define a `LoginPage` object:
```php <?php namespace Tests\Browser\Pages; use Laravel\Dusk\Browser; use Laravel\Dusk\Page as BasePage; class LoginPage extends BasePage { /** * Get the URL for the page. * * @return string */ public function url() { return '/login'; } /** * Assert that the browser is on the page. * * @param Browser $browser * @return void */ public function assert(Browser $browser) { $browser->assertPathIs($this->url()); } /** * Get the element shortcuts for the page. * * @return array */ public function elements() { return [ '@emailField' => 'input[name=email]', '@passwordField' => 'input[name=password]', '@loginButton' => 'button[type=submit]', ]; } public function loginUser(Browser $browser, $email, $password) { $browser->type('@emailField', $email) ->type('@passwordField', $password) ->press('@loginButton'); } } ```
With this page object, we can now refactor our login test:
```php <?php namespace Tests\Browser; use Tests\Browser\Pages\LoginPage; use Laravel\Dusk\Browser; use Tests\DuskTestCase; class LoginTest extends DuskTestCase { /** * A basic browser test example. * * @return void */ public function testUserCanLogin() { $this->browse(function (Browser $browser) { $browser->visit(new LoginPage) ->loginUser('taylor@laravel.com', 'secret') ->assertPathIs('/home'); }); } } ```
This version of the test uses the `LoginPage` object’s `loginUser` method, reducing duplication and improving readability.
6.2. Continuous Integration
Continuous Integration (CI) is a key practice in modern software development, allowing you to detect problems early by integrating code changes regularly and automatically running tests on these changes. Laravel Dusk supports running tests on CI services like Travis CI, CircleCI, and GitHub Actions.
By using Laravel Dusk in your CI pipeline, you can ensure your web application works as expected before any code reaches production.
6.3. Testing JavaScript Applications
One major advantage of Laravel Dusk is its ability to test JavaScript applications. Dusk operates with the ChromeDriver by default, which supports all the modern JavaScript features.
You can easily test Vue.js components, Livewire components, or any other JavaScript-driven behavior in your Laravel application.
Wrapping Up
In this post, we delved into Laravel Dusk, an incredibly powerful tool for end-to-end browser testing in Laravel applications. Leveraging such tools is part of what makes a Laravel developer a valuable asset. We discussed the setup process for Dusk, how to create both simple and advanced browser tests, and examined key features such as Dusk selectors and JavaScript modals. Plus, we navigated the steps to building robust test suites using page objects, continuous integration, and JavaScript testing. This advanced understanding of Dusk is one of the many reasons to hire Laravel developers for your web application needs.
Table of Contents
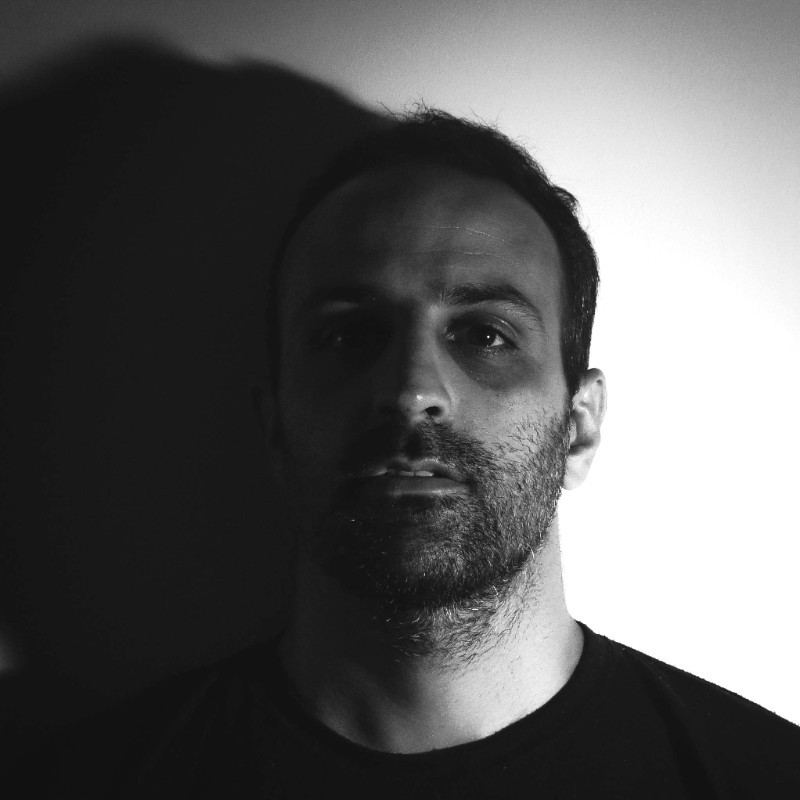
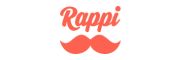