Essential Guide: Writing & Running Tests with Laravel Dusk
Laravel, known for its expressive and elegant syntax, has a suite of tools for different types of testing. One of them is Laravel Dusk, which provides an expressive API for browser testing. Unlike other testing tools, Dusk does not require you to install JDK or Selenium, making the setup relatively straightforward.
This article will walk you through the basics of setting up, writing, and running browser tests using Laravel Dusk.
1. Installation
Before you begin, make sure you have a fresh Laravel installation ready.
To install Laravel Dusk, run:
```bash composer require --dev laravel/dusk ```
Once installed, you can register Dusk’s service provider:
```bash php artisan dusk:install ```
This command will set up Dusk for your application and also create a `Browser` directory under the `tests` folder, where you’ll be storing your Dusk tests.
2. Writing Your First Test
Navigate to `tests/Browser` and you will find an example test provided by Dusk.
Let’s create a simple test to ensure that a user can log in. Consider the following example:
```php <?php namespace Tests\Browser; use App\Models\User; use Laravel\Dusk\Browser; use Tests\DuskTestCase; class LoginTest extends DuskTestCase { /** * A basic test example. * * @return void */ public function testUserCanLogin() { $user = User::factory()->create([ 'email' => 'taylor@laravel.com', 'password' => bcrypt('secret'), ]); $this->browse(function (Browser $browser) use ($user) { $browser->visit('/login') ->type('email', $user->email) ->type('password', 'secret') ->press('Login') ->assertPathIs('/dashboard'); }); } } ```
In the test above:
– A new user is created using Laravel’s Eloquent factories.
– The `browse` method accepts a closure which receives an instance of the browser, allowing you to interact with your application.
– The `visit` method navigates the browser to the given URI.
– The `type` method inputs the given text into the given field.
– Finally, the `assertPathIs` method asserts the current URL matches the given path.
3. Running Tests
To run your Dusk tests, use the `dusk` Artisan command:
```bash php artisan dusk ```
Ensure that you’re not running any local development server like `php artisan serve` when executing Dusk tests, as Dusk will start its server.
4. Interacting with Elements
Dusk offers a range of interaction methods. Let’s explore some of them:
4.1 Clicking Links
You can use the `clickLink` method:
```php $browser->clickLink('Click Me!'); ```
4.2 Using Forms
For forms, Dusk provides methods like `select`, `radio`, `check`, `uncheck`, and `attach`:
```php $browser->select('account_type', 'premium') ->radio('plan', 'monthly') ->check('terms') ->attach('photo', __DIR__.'/photo.jpg'); ```
4.3 Dragging and Dropping
For drag-and-drop interfaces:
```php $browser->drag('.from', '.to'); ```
5. Making Assertions
Dusk allows you to make a wide variety of assertions against your application:
```php $browser->assertTitle('Laravel Dusk') ->assertSee('Welcome, User!') ->assertInputValue('name', 'Taylor') ->assertChecked('terms') ->assertPathIs('/home'); ```
6. Screenshots and Debugging
When a test fails, it’s useful to see what the browser was rendering. Dusk provides:
```php $browser->screenshot('name-for-screenshot'); ```
For more detailed debugging:
```php $browser->dump(); ```
7. Handling Modals
Dusk provides an easy API to work with modals:
```php $browser->press('Show Modal') ->waitForText('Modal Title') ->press('Close Modal'); ```
8. Waiting for Elements
Sometimes you may need to pause the test or wait for certain elements:
```php $browser->waitUntilMissing('.selector') ->waitForText('Hello World'); ```
Conclusion
Laravel Dusk provides a clean, fluent API to write browser tests in a way that feels natural and intuitive. The ability to interact with your application’s UI and make assertions ensures your application is working as expected.
Remember, while Dusk is powerful, it’s also essential to combine it with other types of testing (unit, feature) to ensure complete coverage and reliability of your Laravel application. Happy testing!
Table of Contents
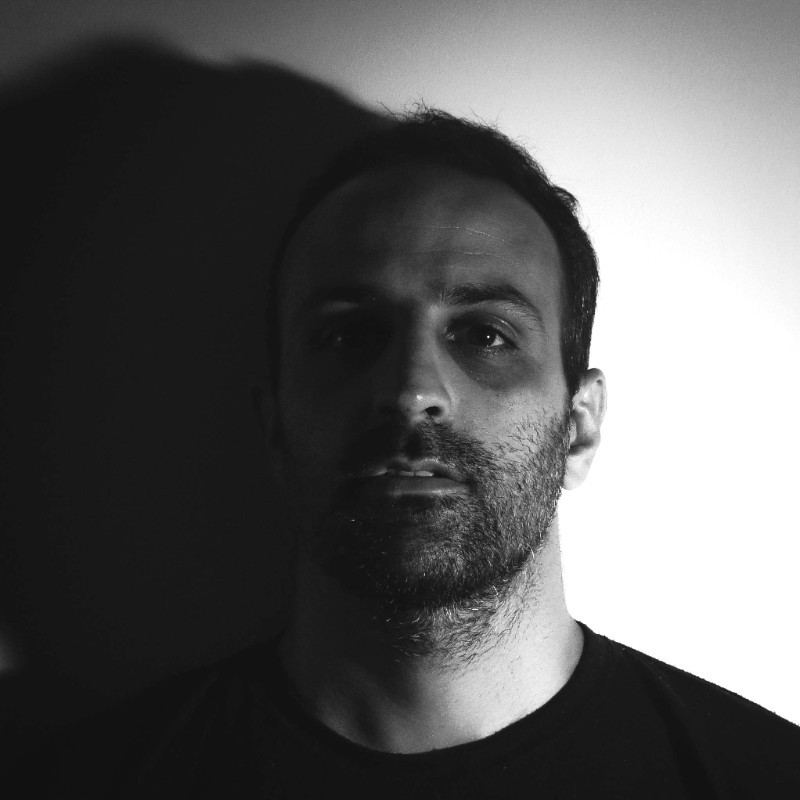
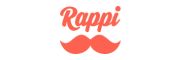