How to perform eager loading in Laravel?
Performing eager loading in Laravel is like anticipating what you’ll need ahead of time—it allows you to load related data along with your primary data query, reducing the number of database queries and improving the performance of your application. Eager loading is particularly useful when working with relationships between database tables in Laravel. Here’s how you can perform eager loading in Laravel in a user-friendly way:
Understanding Eager Loading: In Laravel, eager loading refers to the process of loading related models along with the primary model being queried. This helps you avoid the “N+1 query problem,” where fetching related models results in additional queries for each related record.
Defining Relationships: Before performing eager loading, you need to define relationships between your Eloquent models using Laravel’s relationship methods such as belongsTo, hasOne, hasMany, belongsToMany, etc. These relationships define how different database tables are related to each other.
Using with Method: The with method is used to perform eager loading in Laravel. You can chain the with method to your Eloquent query to specify which related models you want to eager load. For example:
php $posts = Post::with('user')->get();
In this example, we’re eager loading the user relationship for each Post model.
Nested Eager Loading: Laravel also supports nested eager loading, allowing you to load deeply nested relationships with a single query. You can use dot notation to specify nested relationships in the with method:
php $posts = Post::with('user', 'comments.user')->get();
In this example, we’re eager loading the user relationship for each Comment model, which is nested within the comments relationship of the Post model.
Accessing Eager Loaded Data: Once you’ve eager loaded related models, you can access them as properties of the primary model. For example, if you’ve eager loaded the user relationship for each Post model, you can access the user associated with a post like this:
php foreach ($posts as $post) { echo $post->user->name; }
Performance Benefits: Eager loading helps improve the performance of your application by reducing the number of database queries needed to fetch related data. By fetching all related data in a single query, you can avoid the overhead of making multiple database calls and optimize the overall performance of your application.
Considerations: While eager loading can improve performance, it’s essential to be mindful of the amount of data being loaded. Loading too much data eagerly can lead to performance issues and increased memory consumption. Therefore, it’s essential to strike a balance between eager loading and lazy loading based on your application’s needs.
By following these steps, you can effectively perform eager loading in Laravel and optimize the performance of your application by reducing database queries and fetching related data efficiently. Eager loading is a powerful feature of Laravel’s Eloquent ORM that helps streamline data retrieval and improve the overall user experience of your application.
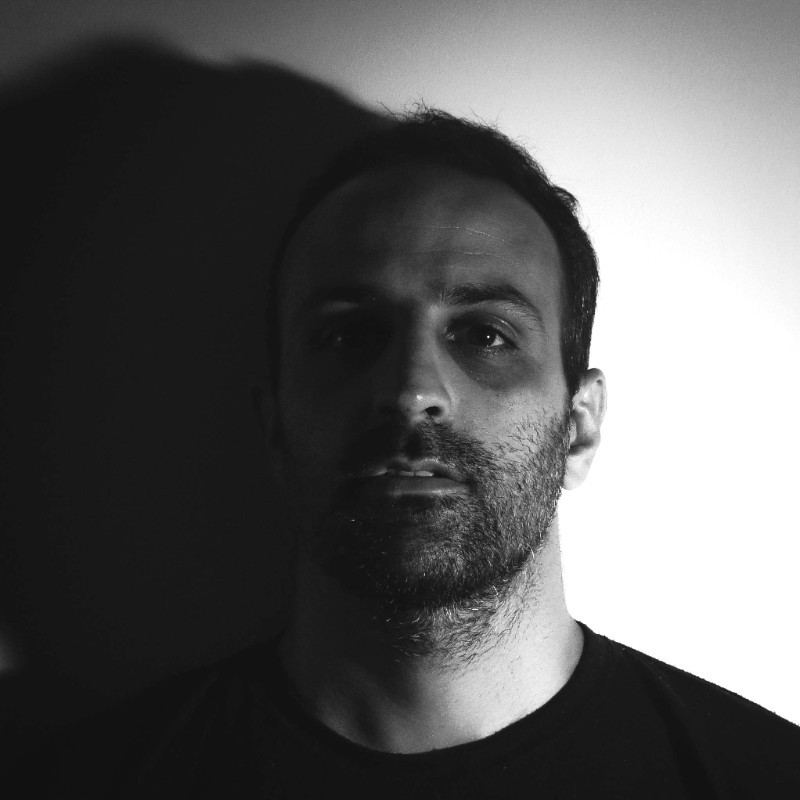
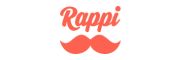