Exploring Laravel’s Eloquent ORM: Simplify Database Operations
In today’s software development landscape, efficient data management has become the backbone of any web application. One of the popular tools that have redefined how developers handle databases is Laravel’s Eloquent Object-Relational Mapping (ORM). This is why businesses are increasingly looking to hire Laravel developers, who can harness the power of Laravel’s robust tools.
The Eloquent ORM, Laravel’s flagship data management tool, brings an easy, eloquent, and enjoyable method to manage database operations in your PHP applications. Experienced Laravel developers make the most of this powerful tool to build dynamic and efficient applications. This blog post will delve into Laravel’s Eloquent ORM, showcasing why you should consider hiring Laravel developers, and how they can simplify database operations.
Introduction to Laravel’s Eloquent ORM
“Eloquent ORM stands out as one of the main features of Laravel that provide an incredibly easy-to-use and powerful tool to interact with your database. This is a primary reason why many organizations look to hire Laravel developers. Through its active record implementation, Eloquent allows developers to perform database queries in an intuitive and object-oriented way.
Rather than writing SQL code, with Eloquent, developers deal with “models.” These are classes that map to database tables. This approach allows you to work with your database data as if they were PHP objects. In the hands of skilled Laravel developers, Eloquent is a formidable tool that simplifies complex database operations. Let’s dive into its crucial aspects and see why it’s beneficial to hire Laravel developers to leverage Eloquent efficiency.”
Eloquent Models
At the heart of Eloquent lies the Model. A model in Laravel corresponds to a table in your database. It represents the data in your table as a set of objects where each object corresponds to a row in the table.
Creating a model is straightforward. To create a `User` model, for instance, you would use the following artisan command:
php artisan make:model User
This command creates a new model class in the `app/Models` directory. By convention, Eloquent uses the plural, snake_case version of the class name to determine the associated database table. Therefore, in this case, the `User` model would be tied to the `users` table. You can also specify a different table name by defining a `protected $table` property in your model.
Basic Operations: CRUD
Eloquent simplicity shines when performing basic CRUD (Create, Read, Update, Delete) operations. Let’s take a look at each operation.
Create: To create a new record in the database, you create a new model instance, set attributes on the model, then call the `save` method.
```php $user = new User; $user->name = 'John Doe'; $user->email = 'johndoe@example.com'; $user->save();
Read: To retrieve records, you can use various methods provided by Eloquent, such as `all`, `find`, `first`, and so on. You can also add conditions to your queries using methods like `where`.
```php // Retrieve all users $users = User::all(); // Find a user by ID $user = User::find(1); // Retrieve the first user who matches the conditions $user = User::where('votes', '>', 100)->first();
Update: Updating a record is as simple as retrieving it, changing an attribute, and calling `save`.
```php $user = User::find(1); $user->email = 'newemail@example.com'; $user->save();
Delete: To delete a record, retrieve it, then call the `delete` method.
```php $user = User::find(1); $user->delete();
Relationships
In a typical database, tables are related. Laravel makes it seamless to work with these relationships using Eloquent. There are several types of relationships: One to One, One to Many, Many to Many, Has_many Through, Polymorphic Relations, and Many to Many Polymorphic Relations.
Each type of relationship is represented as a method in your Eloquent model. Let’s take a quick look at how a One to Many relationship can be defined:
```php class User extends Model { public function posts() { return $this->hasMany('App\Models\Post'); } }
In the example above, we assume that a user can have many posts. To retrieve all posts for a user, we can simply do:
```php $user = User::find(1); $posts = $user->posts;
Eloquent Collections
When you fetch multiple models from the database, Eloquent does not just give you an array, but a powerful Collection instance. Collections are like supercharged arrays with a lot of useful methods for working with data sets.
```php $users = User::all(); // Use the count method on a collection $count = $users->count(); // Use the max method on a collection $max = $users->max('votes');
Conclusion
Laravel’s Eloquent ORM is a powerful tool for managing and manipulating data. Its simplicity, elegance, and large feature set make it a joy to use. This is one of the many reasons why businesses choose to hire Laravel developers. By allowing developers to work with their data as objects instead of writing raw SQL queries, Eloquent significantly simplifies the database operations in Laravel applications.
Whether you are a beginner or an experienced PHP developer, Laravel’s Eloquent ORM is a tool that, once mastered, will undoubtedly boost your productivity and make your development process more enjoyable. As you become more adept with it, you’ll understand why organizations hire Laravel developers to capitalize on this tool. The more you use Eloquent, the more you’ll appreciate its power and eloquence, and the benefits of hiring expert Laravel developers.
Table of Contents
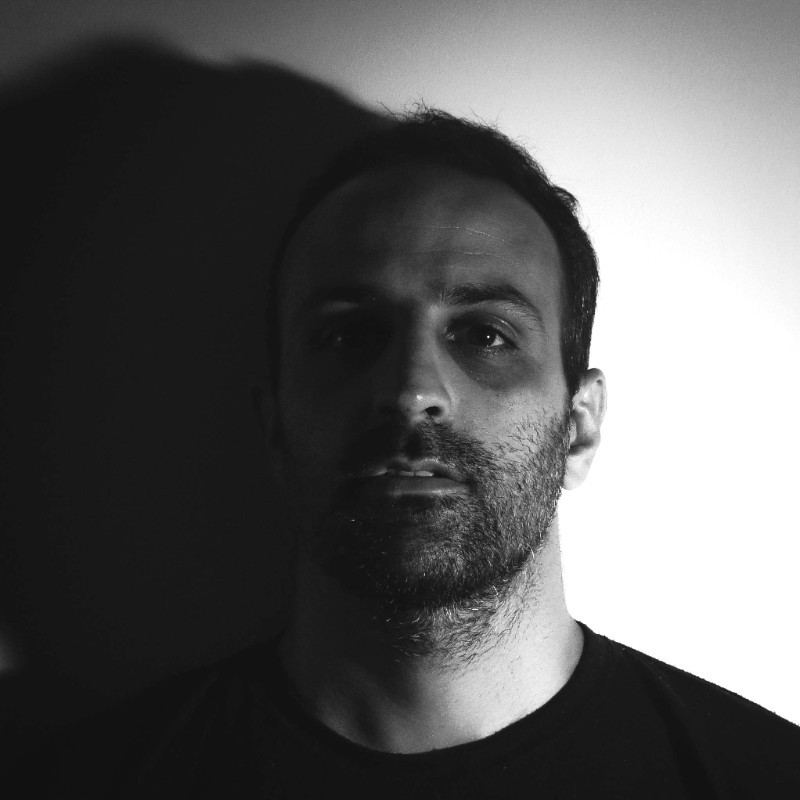
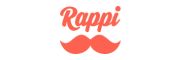