Laravel Error Handling: Effective Strategies for Debugging
As a Laravel developer, encountering errors and bugs is inevitable. However, the key to building robust applications lies in your ability to effectively handle and debug these errors. Laravel, being a powerful PHP framework, provides a range of tools and techniques to simplify the debugging process. In this blog, we will explore some effective strategies for error handling and debugging in Laravel, along with code samples and best practices. Let’s dive in!
Understanding Laravel Error Types
Before we delve into error handling strategies, it’s crucial to have a clear understanding of the different types of errors you may encounter in Laravel:
- Syntax Errors: These occur due to incorrect PHP syntax and can be easily spotted during development.
- Runtime Errors: These errors occur while the application is running and can be caused by invalid database connections, missing files, or other runtime issues.
- Logic Errors: Also known as bugs, these errors result from flawed code logic and may lead to unexpected behaviors or incorrect results.
Now that we have a grasp of the error landscape, let’s explore how to effectively handle and debug them.
Configuring Error Reporting
Laravel provides a configuration file, config/app.php, where you can set the desired error reporting level. By default, Laravel’s debug mode is set to false in production. However, during development, it’s recommended to enable debug mode by setting it to true. This allows you to see detailed error messages and stack traces, making it easier to identify and fix issues.
To enable debug mode, open config/app.php and set:
php 'debug' => env('APP_DEBUG', true),
Exception Handling in Laravel
Laravel’s exception handling mechanism allows you to catch and handle exceptions gracefully. The App\Exceptions\Handler class is responsible for managing exceptions. You can define custom exception handling logic in this class or utilize Laravel’s built-in exception handlers.
Here’s an example of handling a specific exception in Laravel:
php use Illuminate\Database\Eloquent\ModelNotFoundException; use Illuminate\Http\Response; use Symfony\Component\HttpKernel\Exception\NotFoundHttpException; ... public function render($request, Throwable $exception) { if ($exception instanceof ModelNotFoundException || $exception instanceof NotFoundHttpException) { return response()->json(['message' => 'Resource not found'], Response::HTTP_NOT_FOUND); } return parent::render($request, $exception); }
Logging and Log Files
Laravel offers a robust logging system that allows you to track and record application activities. By default, Laravel stores logs in the storage/logs directory. You can leverage logging to capture errors, debug information, and important events during runtime.
To log an error manually, you can use the Log facade:
php use Illuminate\Support\Facades\Log; ... try { // Code that may throw an exception } catch (\Exception $e) { Log::error('Something went wrong: ' . $e->getMessage()); }
Debugging with Laravel Telescope
Laravel Telescope is a powerful debugging and profiling tool that provides real-time insights into your application’s performance. It allows you to monitor database queries, exceptions, and request lifecycles, making it easier to identify and resolve issues.
To install Laravel Telescope, run the following command:
bash composer require laravel/telescope
After installation, you can access Telescope’s dashboard by visiting /telescope in your browser. From there, you can explore various tabs and panels to gain valuable debugging information.
Leveraging Debugging Tools: Xdebug and PhpStorm
Xdebug is a popular PHP extension that provides advanced debugging capabilities. When combined with PhpStorm, a powerful IDE, you can efficiently debug Laravel applications. With Xdebug, you can set breakpoints, step through code, inspect variables, and more.
To get started with Xdebug and PhpStorm, follow the official documentation for configuring and setting up Xdebug with your PHP environment and IDE.
Error Page Customization
When an error occurs in your Laravel application, Laravel automatically renders an error page with a generic message. However, you can customize this error page to provide more meaningful information to users or developers.
To customize the error page, navigate to resources/views/errors and modify the desired error view files, such as 404.blade.php or 500.blade.php. You can add your own HTML, CSS, or even Laravel Blade syntax to enhance the error pages.
Testing and Continuous Integration
A robust testing strategy is crucial for identifying and preventing errors in your Laravel application. Laravel provides a powerful testing framework that allows you to write unit tests, integration tests, and even end-to-end tests using Laravel Dusk.
By integrating testing into your continuous integration (CI) pipeline, you can automatically run tests on each commit or pull request, catching errors early in the development cycle.
Best Practices for Error Handling in Laravel
To ensure effective error handling in Laravel, consider the following best practices:
Use meaningful exception messages and log them appropriately.
Implement proper error response formats, such as JSON for APIs.
Separate exception handling logic from controllers to keep your codebase clean.
Utilize Laravel’s validation and form request classes for input validation.
Implement thorough testing, covering different error scenarios.
Regularly review log files and monitoring tools to proactively identify issues.
Conclusion
Debugging is an essential skill for Laravel developers, and with the right strategies and tools, you can effectively handle errors and build robust applications. By configuring error reporting, utilizing exception handling, logging, and leveraging debugging tools like Laravel Telescope, Xdebug, and PhpStorm, you can streamline the debugging process. Customizing error pages, implementing testing and continuous integration, and following best practices further enhance your error handling capabilities. With these techniques in your arsenal, you’re well-equipped to tackle any errors that come your way and deliver high-quality Laravel applications.
Table of Contents
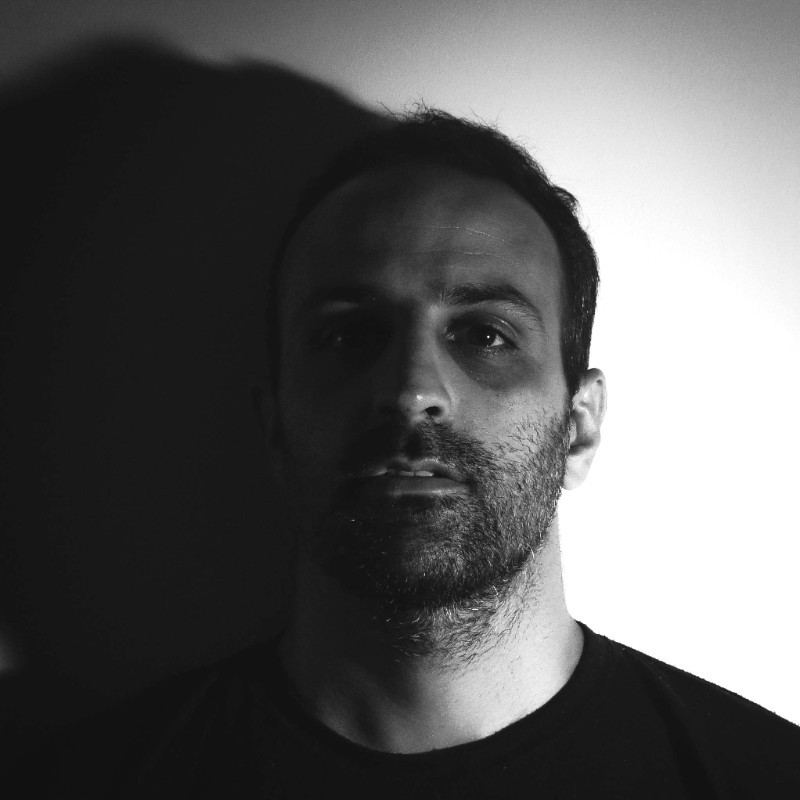
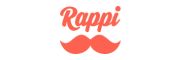