Building Efficient Applications with Laravel’s Event-Driven Architecture
Laravel is an open-source PHP framework teeming with powerful features, including an elegant event system. With Laravel’s built-in event and listeners system, we can implement an event-driven architecture to build decoupled, scalable, and maintainable applications. This architecture’s benefits can be amplified when you hire Laravel developers, as they bring the necessary expertise to fully leverage these features.
In this blog post, we will delve into Laravel’s Events and Listeners. We will explore what they are, why they are crucial, and how we can optimally use them, illustrated with examples. By the end of this post, not only will you have a greater understanding of this pivotal feature but also appreciate the value that professional Laravel developers can add to your project.
What are Events and Listeners?
In Laravel, events provide a simple observer implementation, enabling you to subscribe and listen for various events happening in your application. Event classes mainly hold data related to the event, while listeners are responsible for handling the logic that occurs when an event is triggered.
The key is that events serve as a great way to decouple various aspects of your application, since a single event can have multiple listeners that don’t depend on each other.
Why use Events and Listeners?
- Decoupling Code: Events and listeners allow for decoupling of code, promoting cleaner and more manageable code.
- Easier Testing: With decoupled code, it becomes much easier to write tests since you can test individual components in isolation.
- Better Performance: You can queue listeners to improve your application’s response time for users.
Setting up Laravel Events and Listeners
To generate an event and listener, you can use the following artisan commands:
```bash php artisan make:event OrderShippedEvent php artisan make:listener SendShipmentNotificationListener --event=OrderShippedEvent ```
These commands will generate an event and a listener class. The event will be placed in the `app/Events` directory, and the listener will be in the `app/Listeners` directory.
Now that we have our event and listener classes generated, let’s see them in action.
Example: Implementing Order Shipment Notification
Suppose we have an e-commerce application, and we want to perform a series of actions when an order is shipped. These could include sending an email notification to the customer, logging the shipment, and updating the inventory. These tasks can be handled by different listeners when the order shipped event is fired.
The Event
First, let’s define our `OrderShippedEvent`. The event is merely a data carrier, and its job is to transfer the data to the listener.
```php namespace App\Events; use App\Models\Order; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class OrderShippedEvent { use Dispatchable, InteractsWithSockets, SerializesModels; public $order; /** * Create a new event instance. * * @param Order $order * @return void */ public function __construct(Order $order) { $this->order = $order; } } ```
In the constructor, we accept an `Order` instance and assign it to a public property. Laravel automatically serializes the model instance for us when the event is dispatched.
The Listeners
Next, we have our listeners. We’ll start with the `SendShipmentNotificationListener`.
```php namespace App\Listeners; use App\Events\OrderShippedEvent; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Queue\InteractsWithSockets; use Illuminate\Support\Facades\Mail; class SendShipmentNotificationListener implements ShouldQueue { use InteractsWithSockets; /** * Handle the event. * * @param OrderShippedEvent $event * @return void */ public function handle(OrderShippedEvent $event) { // Access the order using $event->order... Mail::to($event->order->customer_email)->send(new ShipmentNotification($event->order)); } } ```
By implementing `ShouldQueue`, Laravel automatically queues this listener, thereby improving the response time of the application.
Let’s create the `LogShipmentListener`:
```php namespace App\Listeners; use App\Events\OrderShippedEvent; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Support\Facades\Log; class LogShipmentListener implements ShouldQueue { /** * Handle the event. * * @param OrderShippedEvent $event * @return void */ public function handle(OrderShippedEvent $event) { Log::info('Order Shipped: ' . $event->order->id); } } ```
Here, we are simply logging the shipment. We are also queueing this listener by implementing `ShouldQueue`.
Registering Events and Listeners
You need to register the event and its listeners in your `EventServiceProvider`:
```php protected $listen = [ OrderShippedEvent::class => [ SendShipmentNotificationListener::class, LogShipmentListener::class, ], ]; ```
Dispatching the Event
Now, when the order is shipped, you can dispatch the event:
```php event(new OrderShippedEvent($order)); ```
This will trigger all the listeners associated with the `OrderShippedEvent`, thereby sending the email, logging the shipment, and any other actions you’ve specified.
Conclusion
The Laravel event system is a powerful feature that enables a clean, decoupled architecture for your applications. By understanding and leveraging events and listeners, you can write efficient, testable, and maintainable code. This event-driven architecture also helps create applications that are flexible, easier to scale, and have improved performance due to asynchronous processing with queued listeners. If you’re looking to maximize the potential of your project, consider hiring Laravel developers who are adept at implementing this robust feature into your applications. Start experiencing the difference it makes today.
Table of Contents
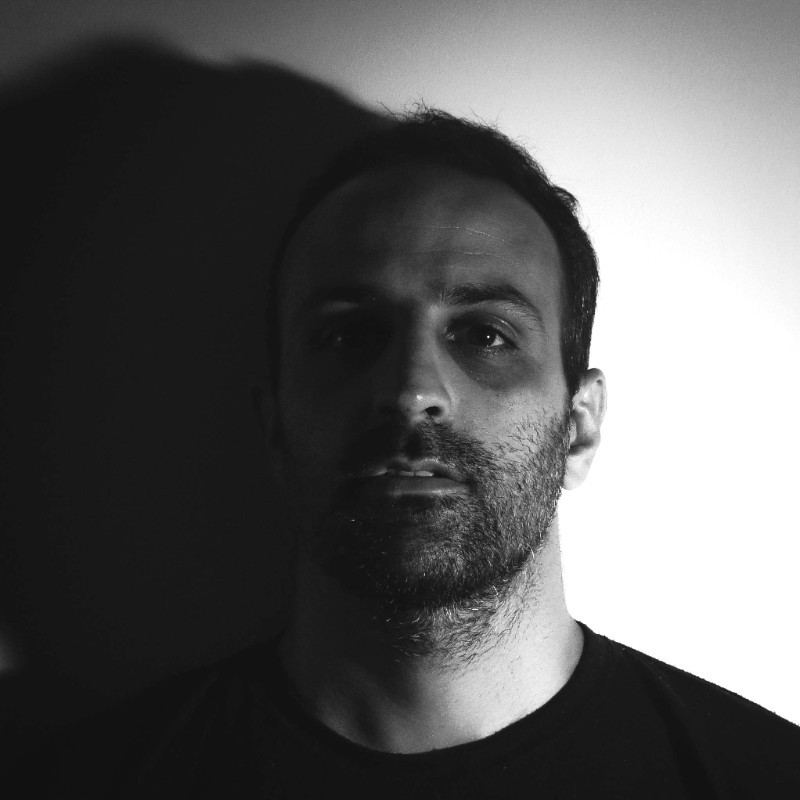
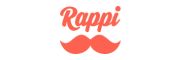