How to work with events and listeners in Laravel?
Working with events and listeners in Laravel is like orchestrating a symphony of actions within your application—it allows you to respond to specific events and execute custom logic in a decoupled and efficient manner. Here’s how you can work with events and listeners in Laravel in a user-friendly manner:
Understanding Events and Listeners: In Laravel, events represent meaningful occurrences within your application, such as user registration, file uploads, or database updates. Listeners are responsible for responding to these events and executing the necessary actions or tasks.
Defining Events: To define an event in Laravel, you can create a new event class that extends the Illuminate\Foundation\Events\Event base class. Within the event class, you can define properties and methods to provide context and data related to the event.
Firing Events: Once you’ve defined an event, you can fire (dispatch) it from any part of your application using Laravel’s event dispatcher. To fire an event, you simply use the event() helper function and pass an instance of the event class:
php event(new UserRegistered($user));
This code fires a UserRegistered event with the specified $user object as its payload.
Creating Listeners: Listeners are classes that handle the logic associated with an event. You can create listener classes using Laravel’s php artisan make:listener command:
bash php artisan make:listener SendWelcomeEmail --event=UserRegistered
This command generates a new listener class named SendWelcomeEmail and associates it with the UserRegistered event.
Implementing Listener Logic: Within the generated listener class, you can define the logic that should be executed when the associated event is fired. For example, if the event is UserRegistered, the listener might send a welcome email to the newly registered user.
Registering Listeners: To register listeners in Laravel, you can use the $listen property in the EventServiceProvider class. This property maps events to their corresponding listener classes:
php protected $listen = [ 'App\Events\UserRegistered' => [ 'App\Listeners\SendWelcomeEmail', ], ];
This configuration tells Laravel to call the SendWelcomeEmail listener whenever a UserRegistered event is fired.
Testing Events and Listeners: It’s important to test your events and listeners to ensure they behave as expected. Laravel provides testing utilities and helpers for simulating event firing and listener execution in your application’s test suite.
By following these steps, you can effectively work with events and listeners in Laravel to build flexible, decoupled, and maintainable applications that respond dynamically to user actions and system events.
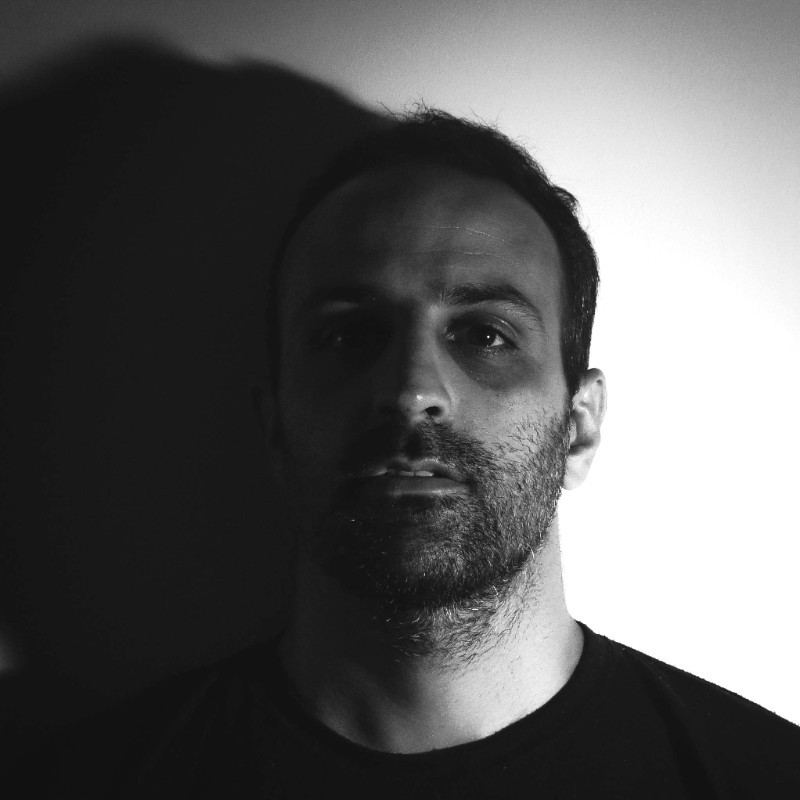
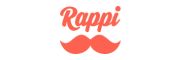